How to call a method after bean initialization is complete?
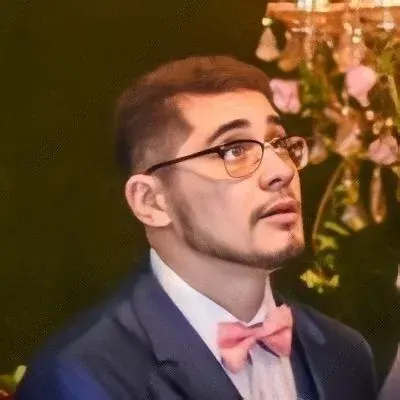
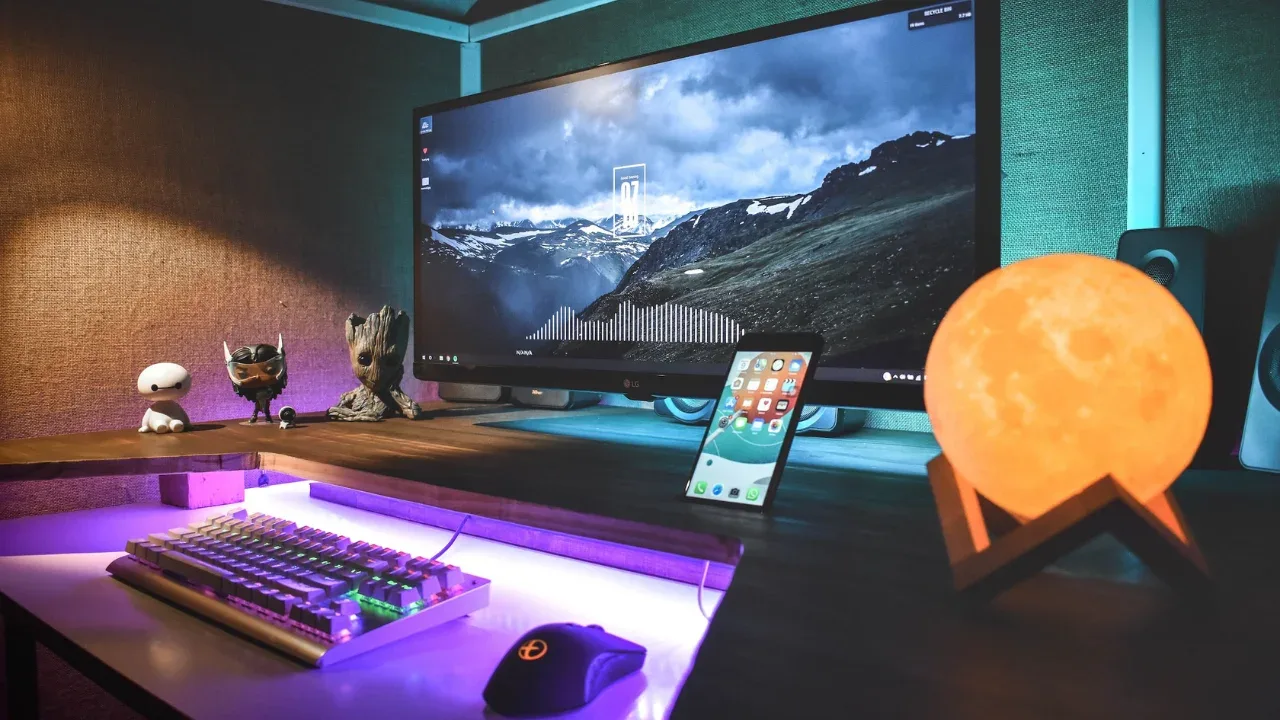
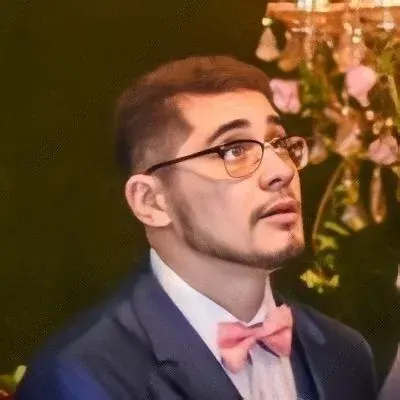
🎉 How to call a method after bean initialization is complete? 🌱
Have you ever encountered a situation where you need to call a method in a bean only once, immediately after the ApplicationContext is loaded? 🤔 This could be a common use case in a web application where you want certain initialization tasks to be performed before serving any requests. But what's the best way to achieve this? Let's find out! 💪
🚀 Option 1: MethodInvokingFactoryBean
One approach you can take is to use the MethodInvokingFactoryBean
. This handy bean from Spring Framework allows you to invoke a method on a target object during initialization. It's easy to use and can get the job done. 💡
Here's how you can do it:
<bean class="org.springframework.beans.factory.config.MethodInvokingFactoryBean">
<property name="targetObject">
<bean class="com.example.MyBean"/>
</property>
<property name="targetMethod">
<value>methodA</value>
</property>
</bean>
In the example above, we define a MethodInvokingFactoryBean
and set the target object to an instance of the MyBean
class. We also specify the method we want to invoke, which is methodA
in this case. Once the bean is instantiated, the specified method will be called automatically. 😎
🚀 Option 2: InitializingBean interface
Another way to achieve the desired behavior is by using the InitializingBean
interface. This is a marker interface provided by Spring Framework that allows you to perform initialization logic after a bean has been constructed. It provides a single method: afterPropertiesSet()
, which you can override to define your custom initialization code. 🛠️
Here's an example:
import org.springframework.beans.factory.InitializingBean;
public class MyBean implements InitializingBean {
// ...
@Override
public void afterPropertiesSet() throws Exception {
// Your initialization logic here
methodA();
}
}
In this approach, we create a custom class MyBean
and implement the InitializingBean
interface. Inside the afterPropertiesSet()
method, we can perform any initialization tasks we want, including calling methodA()
. This method will be automatically invoked once the bean has been constructed. 💫
⚡️ The Better Solution
While both options mentioned above will work, there might be an even better solution for your use case. Instead of tying your initialization logic directly to the bean instantiation process, you can utilize Spring's event mechanism.
By listening to the ContextRefreshedEvent
, you can perform your initialization task only when the ApplicationContext has finished initializing. This ensures that all the beans are successfully constructed and ready to be used. 🌟
Here's how you can achieve this:
import org.springframework.context.ApplicationListener;
import org.springframework.context.event.ContextRefreshedEvent;
public class MyApplicationListener implements ApplicationListener<ContextRefreshedEvent> {
@Override
public void onApplicationEvent(ContextRefreshedEvent event) {
// Your initialization logic here
methodA();
}
}
In this approach, we create a custom class MyApplicationListener
that implements ApplicationListener<ContextRefreshedEvent>
. Inside the onApplicationEvent()
method, we can put the desired initialization code, including calling methodA()
. The event will be triggered once the ApplicationContext has finished loading, and your method will be executed at that point. 🎊
To register this custom listener, you can either define it in your XML configuration file or use Spring's @EventListener
annotation if you're using Spring Boot.
🚀 Conclusion
🌱 You now have multiple options to call a method after bean initialization is complete. Whether you choose the MethodInvokingFactoryBean
, implement the InitializingBean
interface, or use the ContextRefreshedEvent
listener, it ultimately depends on your specific requirements.
Remember, each option has its pros and cons, so choose the one that suits your use case the best. Experiment, test, and see what brings the most elegant and maintainable solution for your application. Happy coding! 🎉
If you found this blog post helpful, why not share it with your fellow developers? If you have any questions or other cool tips, feel free to leave a comment below. Let's keep the conversation going! 👇👇👇