How to avoid the "Circular view path" exception with Spring MVC test
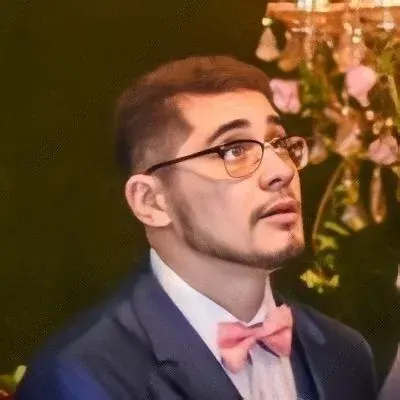
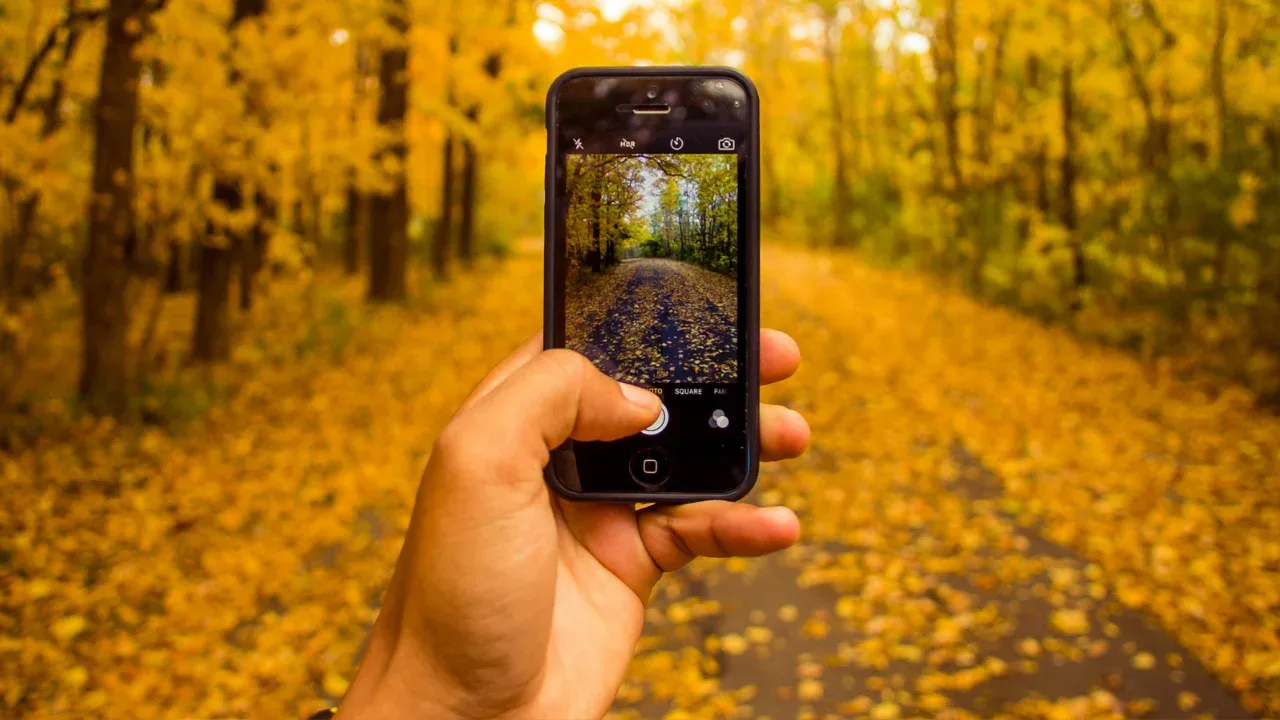
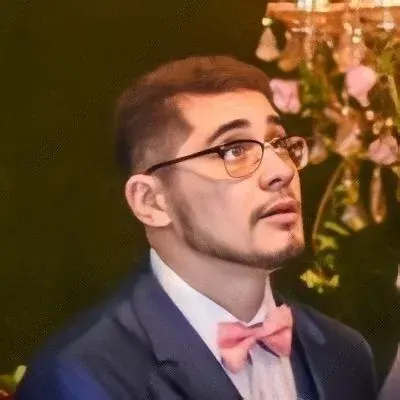
How to Avoid the "Circular view path" Exception with Spring MVC Test
Testing your Spring MVC application is important to ensure that it functions correctly. However, you might encounter the "Circular view path" exception when trying to test a controller that renders views. In this blog post, we will explore this issue and provide easy solutions to avoid this exception with Spring MVC Test.
The Problem
Let's start by understanding the problem at hand. You have a controller called PreferenceController
with a request mapping for /preference
. When testing this controller using Spring MVC Test, you encounter the following exception:
Circular view path [preference]: would dispatch back to the current handler URL [/preference] again. Check your ViewResolver setup! (Hint: This may be the result of an unspecified view, due to default view name generation.)
This exception occurs when the view resolver is unable to resolve the view properly, resulting in a circular reference.
The Solution
To avoid the "Circular view path" exception, you can follow these steps:
Step 1: Configure the View Resolver
In your context configuration, ensure that you have properly configured the view resolver. Use the appropriate view resolver for your view technology (e.g., Thymeleaf, Freemarker, JSP).
For example, if you are using Thymeleaf, you can configure the view resolver as follows:
<bean class="org.thymeleaf.templateresolver.ServletContextTemplateResolver" id="webTemplateResolver">
<property name="prefix" value="WEB-INF/web-templates/" />
<property name="suffix" value=".html" />
<property name="templateMode" value="HTML5" />
<property name="characterEncoding" value="UTF-8" />
<property name="order" value="2" />
<property name="cacheable" value="false" />
</bean>
Make sure that the prefix
and suffix
properties match your actual view templates' location and extension.
Step 2: Use the Standalone Setup
To avoid the "Circular view path" exception when testing with Spring MVC Test, use the standalone setup instead of loading the full context configuration.
In your test class, modify the setup method as follows:
@Before
public void setup() {
mockMvc = MockMvcBuilders.standaloneSetup(new PreferenceController()).build();
}
By using the standalone setup, you isolate the controller being tested and only load the necessary dependencies. This prevents any circular references that might occur due to a complete context configuration.
Step 3: Write Your Test
Now, you can write your test method without encountering the "Circular view path" exception:
@Test
public void preferenceTest() throws Exception {
mockMvc.perform(get("/preference"))
.andExpect(status().isOk())
.andExpect(view().name("preference"));
}
In this example, we perform a GET request to /preference
and expect a successful response with a view name of "preference".
Conclusion
Testing your Spring MVC application using Spring MVC Test is essential for validating its functionality. By following the steps outlined in this blog post, you can avoid the "Circular view path" exception and effectively test your application.
Remember, properly configuring your view resolver and using the standalone setup are crucial to prevent circular references and ensure successful testing.
Now that you have learned how to avoid the "Circular view path" exception, it's time to put this knowledge into practice and enhance your testing efforts!
If you have any questions or face any issues, feel free to leave a comment below. Happy testing! 😊