Disable all Database related auto configuration in Spring Boot
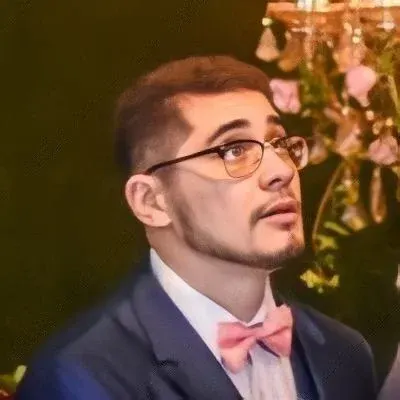
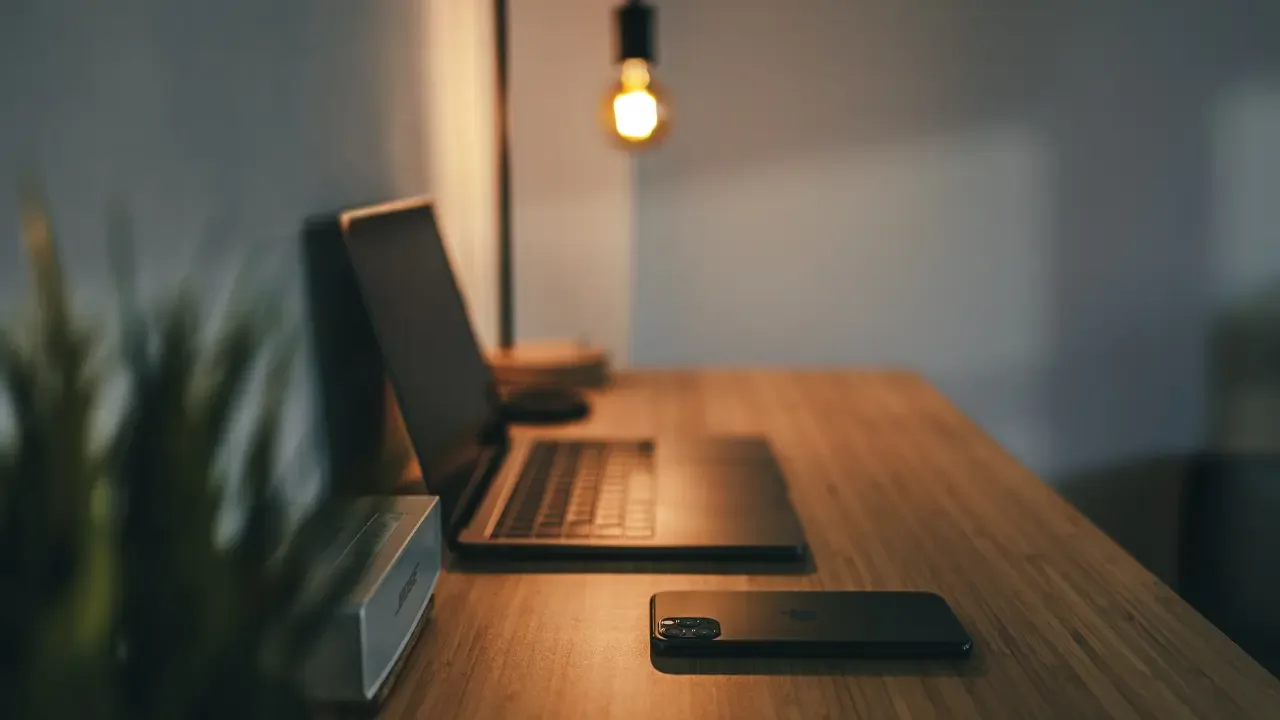
How to Disable Database Auto Configuration in Spring Boot
Are you developing a Spring Boot application and want to disable all database-related auto configuration for a specific profile? You've come to the right place. In this guide, we'll walk you through the steps to achieve this and address common issues you might encounter along the way.
The Problem
You mentioned that you have two applications based on Spring Boot - a server app and a client app. These apps share the same codebase but function differently based on the active profile. For the client app, you want to disable all database-related auto configuration. This means the client app should not establish a database connection or utilize any Spring Data or Hibernate features.
Using application.properties for Conditional Configuration
You asked if creating two different application.properties
files for each profile would solve the problem. While this approach seems reasonable, the exclusions you tried might not be sufficient. Let's explore alternative solutions.
Solution: Conditional Exclusions in application.properties
To disable database-related auto configuration, you can specify exclusion properties in your application.properties
file. However, the exclusions you provided might not cover all the necessary configurations. Here's an improved version of the exclusions:
spring.autoconfigure.exclude=org.springframework.boot.autoconfigure.jdbc.DataSourceAutoConfiguration,\
org.springframework.boot.autoconfigure.jdbc.DataSourceTransactionManagerAutoConfiguration,\
org.springframework.boot.autoconfigure.orm.jpa.HibernateJpaAutoConfiguration,\
org.springframework.boot.autoconfigure.data.web.SpringDataWebAutoConfiguration
Make sure to include the backslash \
at the end of each line, except the last one. This ensures proper line continuation.
Caveat: Class Loading and Order
Sometimes, even with the correct exclusions in place, your application may still attempt to connect to the database. This is because Spring Boot's auto configuration mechanism relies on class loading and the order in which the configurations are processed. If the excluded configurations are loaded before the exclusions take effect, they may still attempt to establish a database connection.
To overcome this issue, you can create a custom @Configuration
class and use the @AutoConfigureBefore
annotation to ensure it is loaded before the problematic configurations. In this custom configuration class, you can provide your own implementation of the beans that would have been auto-configured by Spring Boot.
For example:
@Configuration
@AutoConfigureBefore({DataSourceAutoConfiguration.class, HibernateJpaAutoConfiguration.class})
public class DatabaseConfiguration {
// Your custom configuration code here
}
By placing this custom configuration class before the auto configuration classes you want to exclude, you can prevent the unwanted database connections.
Conclusion
Disabling database-related auto configuration for a specific profile in Spring Boot can be a bit tricky. However, by using conditional exclusions in your application.properties
file and understanding the class loading order, you can overcome this challenge.
Remember to continuously test and iterate your solutions to ensure they meet your specific requirements. Happy coding! 🚀
Your Feedback Matters!
We hope this guide helped you resolve your database auto configuration issue in your Spring Boot project. If you have any questions or face any difficulties, feel free to leave a comment below. We'd love to hear from you.
Let's make tech easy together! 😊
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
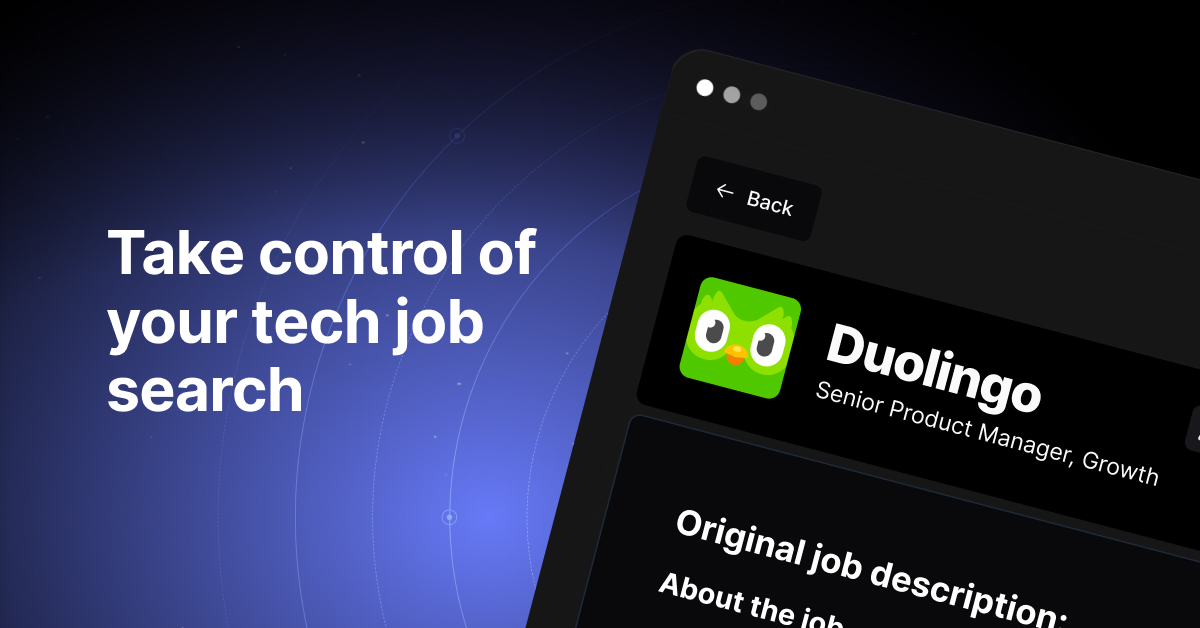