In Laravel, the best way to pass different types of flash messages in the session
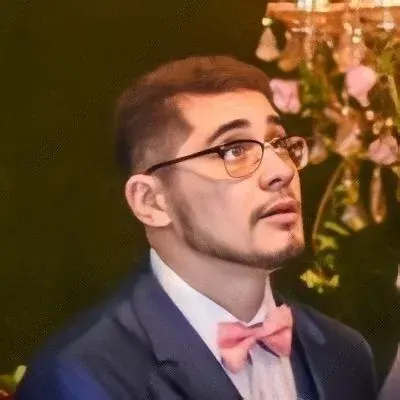
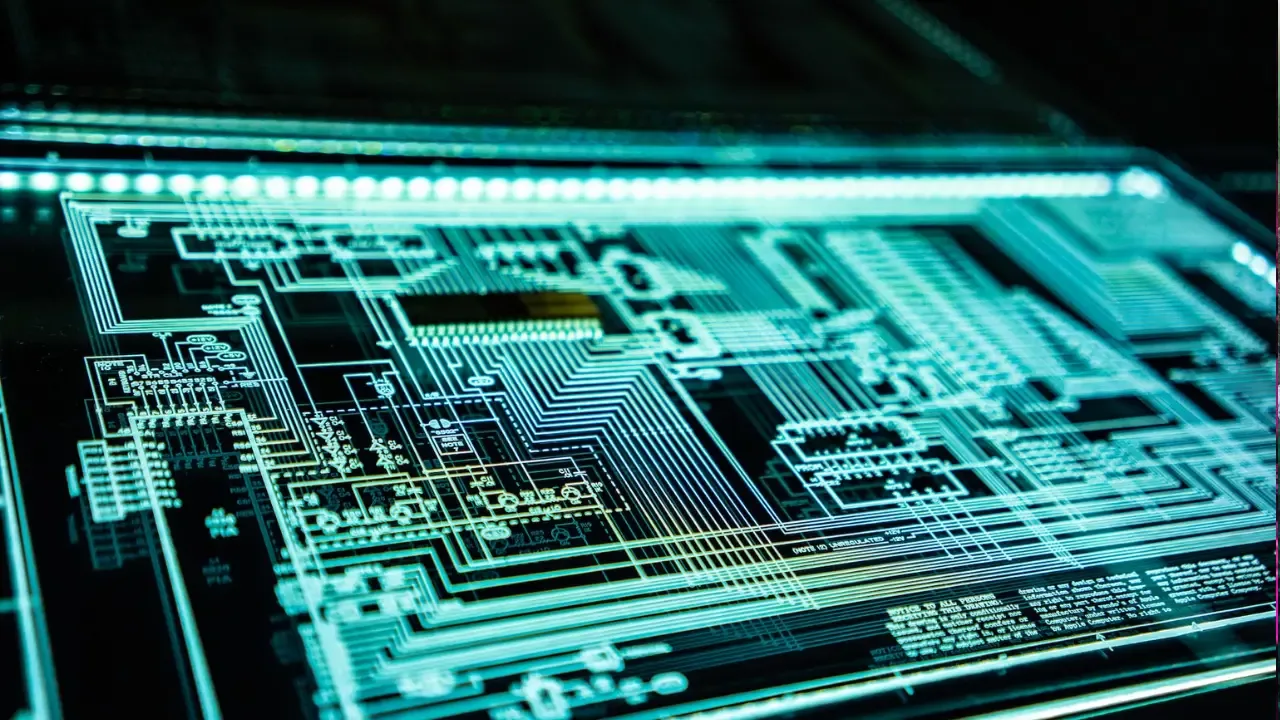
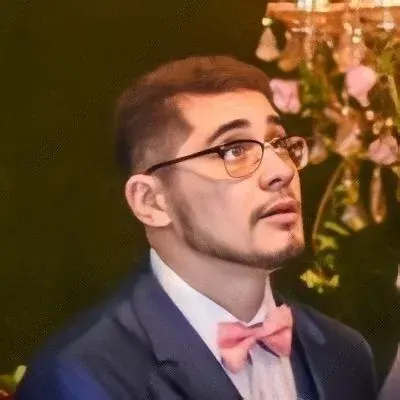
š Laravel Flash Messages - A Comprehensive Guide
š Hey there, fellow Laravel developer! Today, I want to talk to you about a topic that often confuses newbies and even some experienced developers - flash messages in Laravel. Flash messages are a powerful way to provide feedback or notify users about important actions or events. In this blog post, we'll dive into the best practices for passing and displaying different types of flash messages in your Laravel application.
š” Understanding Flash Messages
Before we jump into the solution, let's quickly understand what flash messages are. Flash messages are temporary messages that are stored in the session for a single request. They are usually displayed once to the user and then automatically removed from the session.
š The Problem(s)
The original question highlights a couple of challenges that developers often face when working with flash messages. The first challenge is how to set the flash message in the controller action. The second challenge is how to handle and display different types of flash messages in the view using Bootstrap classes.
š” Solution
Let's tackle these challenges one by one. Firstly, to set a flash message in the controller action, you have a couple of options. The first option mentioned in the question, Redirect::to('users/login')->with('message', 'Thanks for registering!');
, is perfectly valid. It sets the flash message in the session, ready to be displayed in the subsequent request.
Another option, as mentioned in the question, is to use Session::flash('message', 'This is a message!');
. This achieves the same result but is more concise.
š Handling Different Types of Flash Messages
To handle different types of flash messages and display them with Bootstrap classes, we can follow a neat solution. Instead of setting a separate flash message in the session for each message type, we can set a single session key with an array of messages. Each message in the array will have two properties: text
(the actual message) and type
(the Bootstrap class).
Here's an example of how you can achieve this:
// In your controller action
$messages = [
['text' => 'Thanks for registering!', 'type' => 'alert-info'],
['text' => 'Something went wrong!', 'type' => 'alert-danger'],
// Add more messages as needed
];
Session::flash('flash_messages', $messages);
In your blade template, you can loop through the messages array and display them accordingly:
@if(Session::has('flash_messages'))
@foreach(Session::get('flash_messages') as $message)
<p class="alert {{ $message['type'] }}">{{ $message['text'] }}</p>
@endforeach
@endif
š Conclusion and Call-to-Action
And there you have it! You now know the best way to pass and display different types of flash messages in Laravel. By following this approach, you can easily handle multiple flash messages with Bootstrap classes, providing a better user experience.
I hope this guide helped you clear up any confusion you had about flash messages in Laravel. If you have any further questions or tips to share, feel free to leave a comment below. Happy coding! š