Scroll to the top of the page after render in react.js
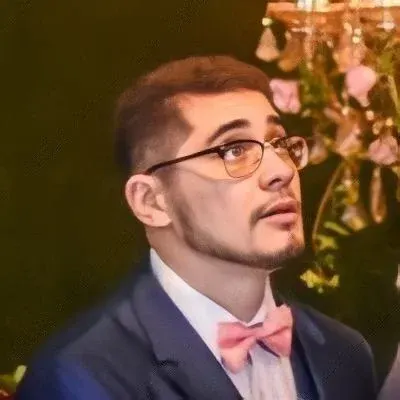
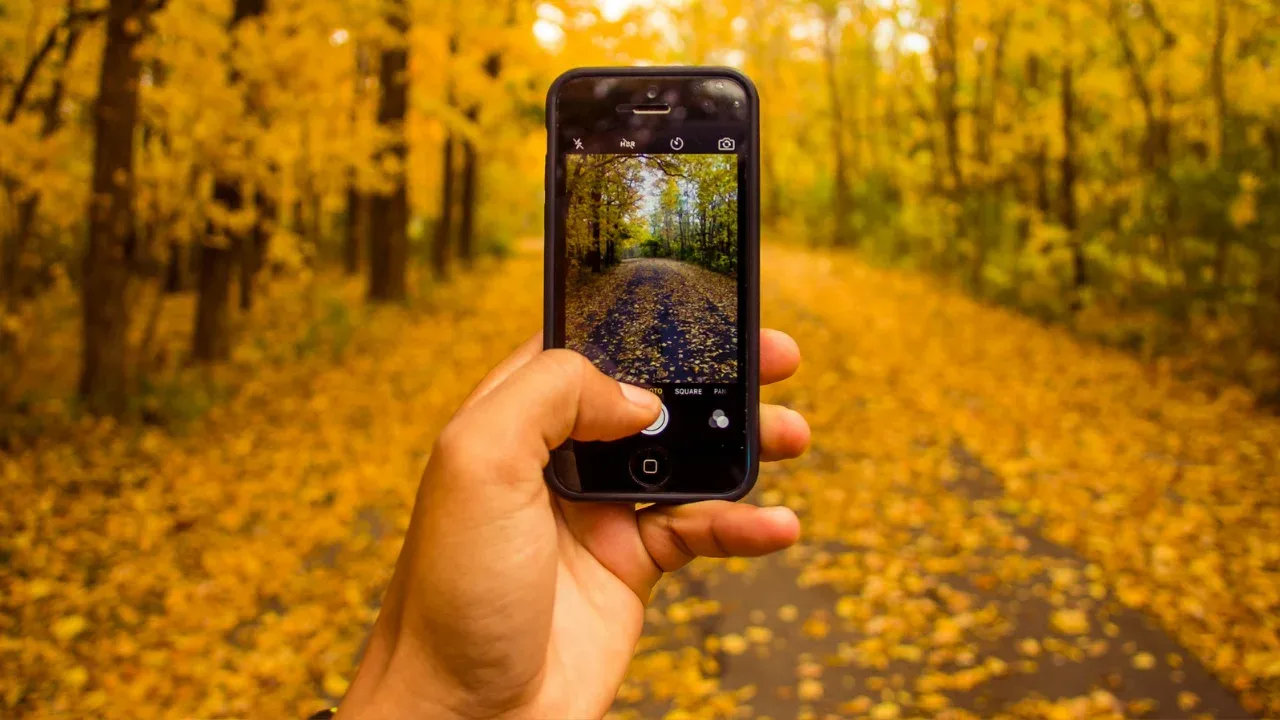
How to Scroll to the Top of the Page After Rendering in React.js
š Welcome to my tech blog, where we make complex problems more digestible! Today, we're diving into the issue of scrolling to the top of the page after rendering in React.js.
If you've ever had a long list of data in a React component and needed to scroll to the top after loading new content, you've likely encountered this challenge. But fear not, because I'm here to provide you with easy solutions! šŖ
The Problem
Let's start by understanding the problem at hand. In the given code snippet, we have a React component that displays a long list of data and a few links at the bottom. Whenever a link is clicked, the list is updated with a new collection of links, and we want the page to automatically scroll back to the top.
The issue is that we need to scroll after the new collection is rendered, but React doesn't provide a built-in solution for this particular problem. So, we have to come up with a clever workaround. š¤
The Solution
Fortunately, we can achieve the desired effect by utilizing the power of JavaScript and the DOM. Here's a step-by-step guide to implementing the solution:
Import the
useEffect
hook from React: Before we proceed, make sure you're using a version of React that supports hooks (React 16.8 or above). In your component file, add the following import statement at the top:
import React, { useEffect } from 'react';
Add a
ref
to the top element: In your component'sreturn
statement, make sure to add aref
to the top element that you want to scroll to. For example, if you want to scroll to the top of the entire page, you can add aref
to the outermost<div>
:
const scrollToTopRef = useRef();
return (
<div ref={scrollToTopRef}>
{/* Your component's content */}
</div>
);
Write the scrolling logic: After the
return
statement, add the following code to scroll to the top whenever the component's state or props change:
useEffect(() => {
if (scrollToTopRef.current) {
scrollToTopRef.current.scrollTo({
top: 0,
behavior: 'smooth',
});
}
}, [/* List any relevant dependencies here, such as props or state */]);
Sit back and enjoy the scroll: With the above code, each time the component's state or props change, it will automatically scroll to the top. You can customize the scrolling behavior by modifying the values of the
top
andbehavior
properties.
That's it! With just a few lines of code, you can now scroll to the top of the page after rendering in React.js. š
A Word of Caution
Before you go on an excited scrolling spree, keep in mind that this solution relies on the browser's built-in scrolling capabilities. If the browser doesn't support smooth scrolling (older versions or certain mobile browsers), the scrolling behavior may not be as smooth as expected. In these cases, the page will still scroll to the top, but without the smooth animation.
Conclusion
I hope this guide has helped you overcome the challenge of scrolling to the top of the page after rendering in React.js. By leveraging the useEffect
hook and the DOM's scroll functionality, you can achieve a seamless user experience.
Feel free to incorporate this solution into your own React projects and share it with your fellow developers who might be struggling with the same issue. Remember, sharing is caring! š
If you have any questions, suggestions, or other topics you'd like me to cover in future blog posts, please leave a comment below. Let's keep the conversation going! š£ļøš¬
Happy scrolling, and until next time! š
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
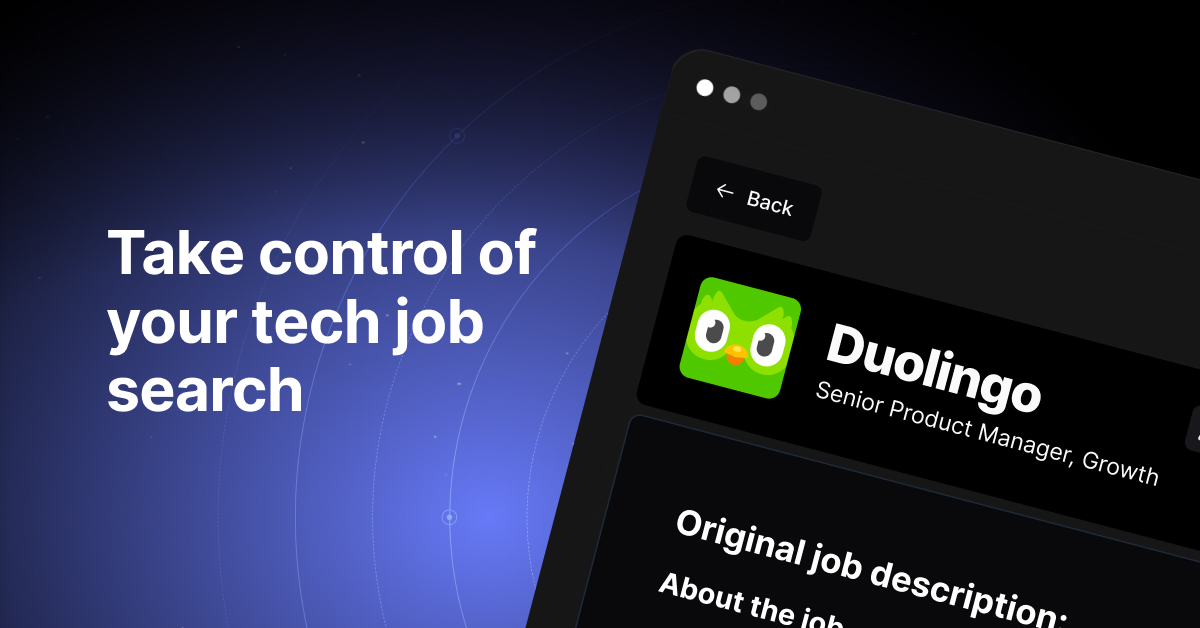