What is the Ruby <=> (spaceship) operator?
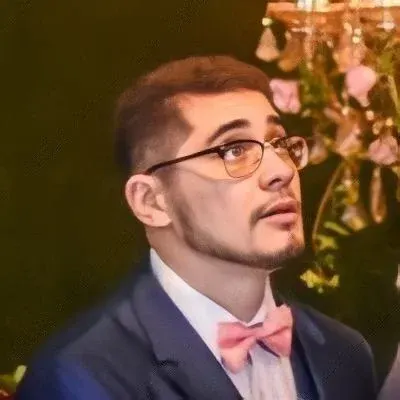
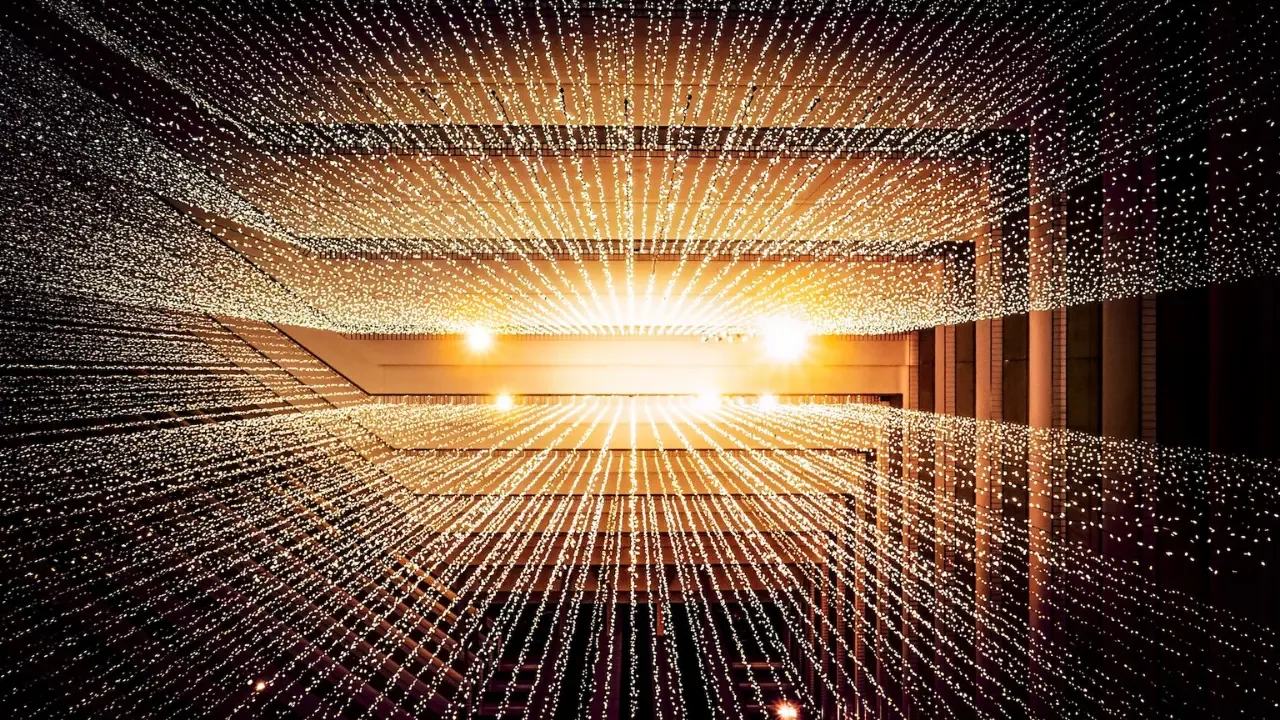
Ruby <=> (spaceship) operator: Unlocking the Mystery š½
š Hey there, tech enthusiasts! Have you ever come across the confusing Ruby <=>
operator? š¤ Don't worry, you're not alone! This operator, often called the "spaceship" operator, may seem alien at first glance, but fear not! We're here to demystify it and make it your new best friend in Ruby programming. š
What's the deal with the spaceship operator?
The <=>
operator is a powerful tool that allows you to perform a three-way comparison between two values. It returns 0
if the two values are equal, 1
if the left value is greater, and -1
if the right value is greater. Sounds handy, right? š
How does it work in Ruby?
Here's an example where we use the spaceship operator to compare two numbers:
number1 = 10
number2 = 5
puts number1 <=> number2
In this case, the output will be 1
because number1
is greater than number2
. š
You can also use the spaceship operator with other data types, such as strings:
string1 = "apple"
string2 = "banana"
puts string1 <=> string2
In this scenario, the output will be -1
because "apple" comes before "banana" in alphabetical order. šā¤ļøš
Does any other language use this operator?
Absolutely! Ruby isn't the only language that has embraced this quirky spaceship operator. In fact, several other programming languages also implement the <=>
operator in similar ways. Some of these languages include Perl, PHP, and Swift, among others. š
Handling common issues with the spaceship operator
Now, let's address some common issues you may encounter when working with the spaceship operator and discuss how to overcome them:
Issue #1: Custom object comparisons
ā ļø When you're comparing custom objects, the spaceship operator might not work as expected by default. This happens because Ruby doesn't automatically know how you want your objects to be compared.
Solution: Implement the Comparable
module
āļø By including the Comparable
module and implementing the <=
method, you can define your own comparison logic for custom objects. This allows the spaceship operator to work its magic correctly. š
Here's a quick example:
class Person
include Comparable
attr_reader :age
def initialize(age)
@age = age
end
def <=>(other)
age <=> other.age
end
end
person1 = Person.new(25)
person2 = Person.new(30)
puts person1 <=> person2
In this case, we're comparing two instances of the Person
class based on their ages. The spaceship operator knows how to handle this thanks to the Comparable
module and our implementation of the <=>
method. š„
Issue #2: Complex data types
ā ļø The spaceship operator might not produce the desired results with complex data types, such as arrays or hashes. This happens because these data types lack a natural order by default.
Solution: Leverage the sort
method
āļø A handy solution is to use the sort
method, which internally utilizes the spaceship operator to perform accurate comparisons. By sorting your data before comparison, you'll get reliable results. š
Here's an example:
array1 = [3, 2, 1]
array2 = [5, 4, 6]
puts array1.sort <=> array2.sort
In this case, we sort both arrays before performing the comparison. The spaceship operator does the rest and gives us the correct result. š
It's time to embrace the spaceship operator! ššŗ
Congratulations, space cadets! You've unlocked the powers of the Ruby spaceship operator! ⨠We've discovered how it works, seen its usage in Ruby, explored other languages that utilize it, and even tackled common issues along the way. You're now equipped to use this operator effectively in your own code. š
So go out there, explore the depths of Ruby's spaceship operator, and let it guide you on your coding adventures! And remember, keep exploring, keep hacking! š©āš»šØāš»
š Do you have any exciting spaceship operator experiences to share? Drop a comment below and let's blast off into a discussion! š½šš¬
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
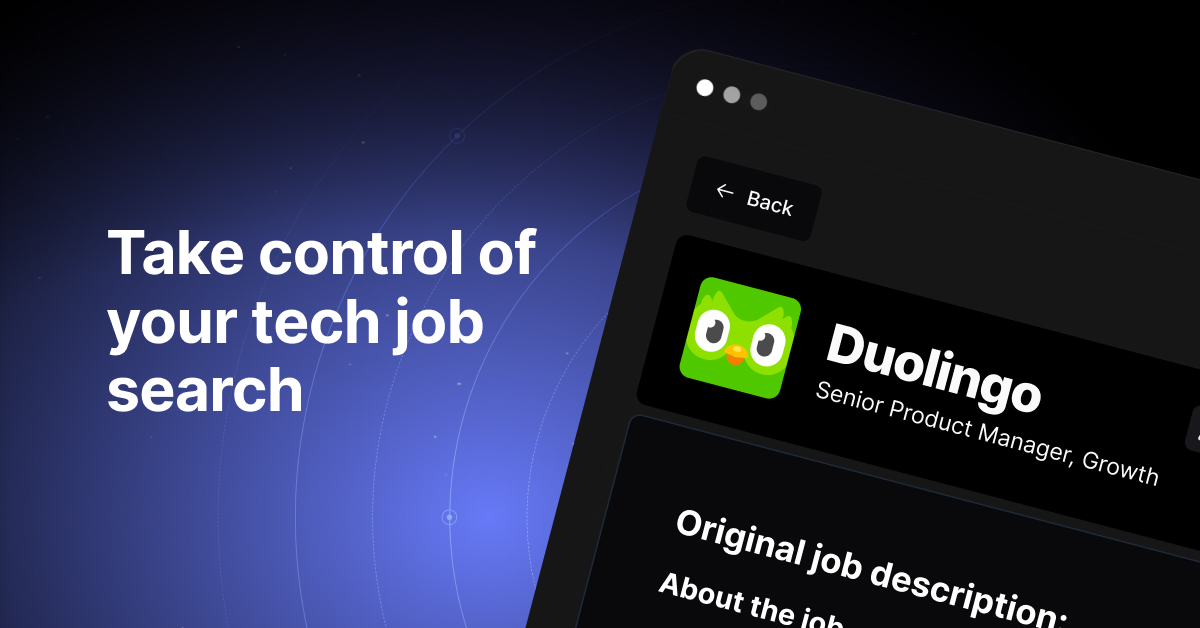