What is the difference between include and require in Ruby?
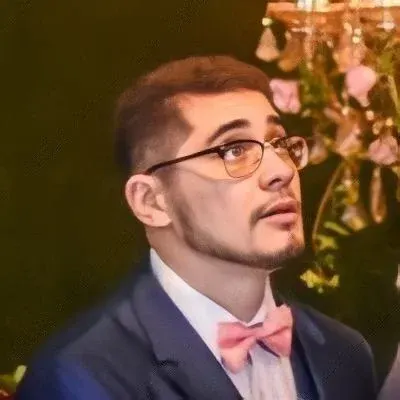
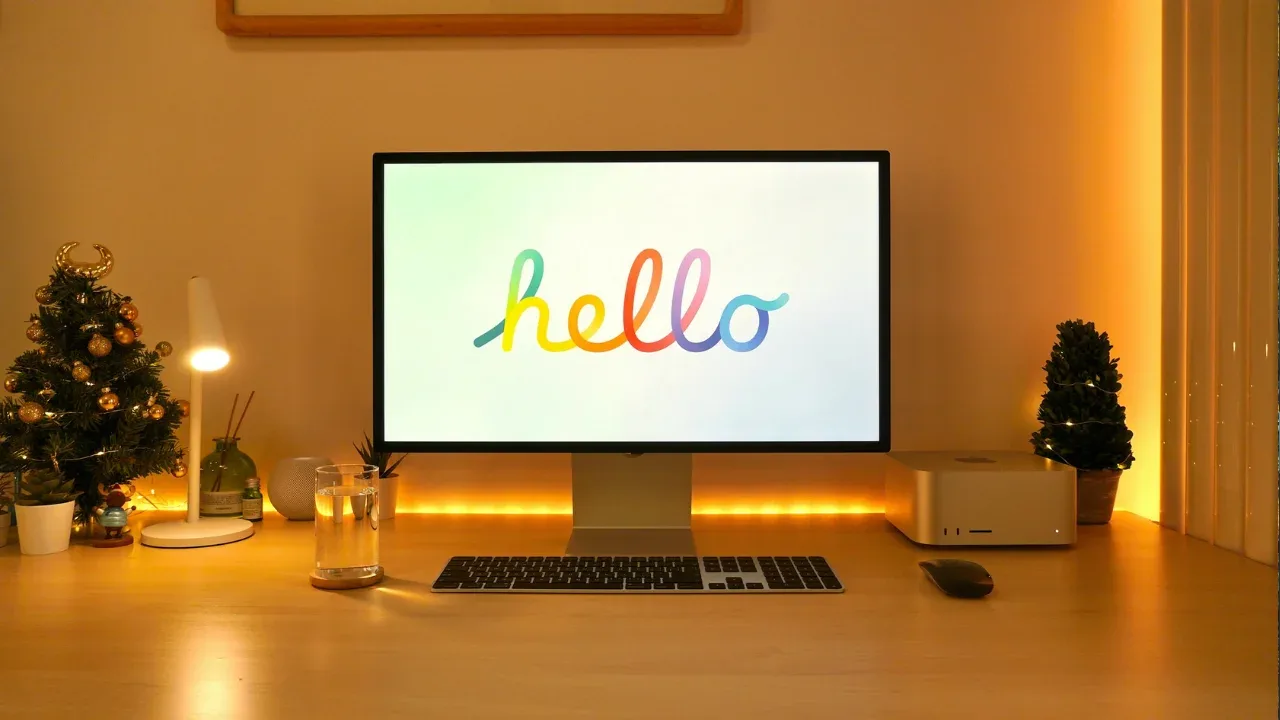
Understanding the Difference between include
and require
in Ruby
š¤ So you want to know the difference between include
and require
in Ruby? Trust me, you're not alone! It's a common question that often confuses even experienced Ruby developers. š But fret not, my fellow tech enthusiasts, because I'm here to break it down for you in a way that's easy to understand. š¤
The Basics: What Do include
and require
Do?
Both include
and require
are used in Ruby to make code from external files or modules available in your current file. However, they work in slightly different ways and have distinct purposes. Let's take a closer look at each of them.
require
: Load External Libraries and Files
The require
keyword is primarily used to load external files or libraries into your Ruby program. Think of it as bringing in an external resource that your code needs to function properly. š
When you require
something, Ruby looks for the specified file or library and loads it into memory. This way, you can access its functionality within your current file. It's like inviting a friend over to your house so you can mooch off their skills and knowledge. š š¤š
Let's see an example:
require 'net/http'
response = Net::HTTP.get('example.com', '/index.html')
puts response
In this example, we're using require
to load the net/http
library, which provides HTTP functionality. Now, we can use the get
method to fetch the contents of a web page and print it out using puts
.
š” Pro Tip: If you need to require
a file relative to your current file, use the require_relative
keyword instead. It's handy when working with local project files.
include
: Mix in Module Methods
Now, let's talk about include
. Unlike require
, which loads external files, include
is used to bring in methods and functionality from modules directly into your class or module. It's like having a personal assistant who knows all the cool tricks and shares them with you. š©āØ
When you include
a module, its methods become accessible to the class or module where you call include
. Pretty neat, huh? š
Let's take a look at an example to see include
in action:
module HelperMethods
def greet(name)
puts "Hello, #{name}!"
end
end
class Person
include HelperMethods
end
person = Person.new
person.greet('Alice')
In this example, we define a module called HelperMethods
with a single method, greet
. Then, in our Person
class, we include
the HelperMethods
module. Voila! Now, instances of the Person
class have access to the greet
method.
š” Pro Tip: Remember that include
is used for instance methods, while extend
is used for class methods. If you want to learn more about extend
, check out my previous blog post linked in the question context. š
So, require
or include
? Which One Should You Use?
Ah, the big question! When do you use require
, and when do you use include
?
The answer lies in understanding their purposes:
Use
require
when you need to load an external library or file that your code depends on, like when you want to use functionality from Ruby's standard or third-party libraries.Use
include
when you want to bring in methods or functionality from a module directly into your class. It helps you keep your code organized and modular.
š” Pro Tip: Just a small heads up! If you try to use include
with a file instead of a module, Ruby will throw an error. So remember, modules are best friends with include
, external files love require
. Keep that in mind! š¤
Conclusion and Call-to-Action
Now that you know the difference between include
and require
in Ruby, it's time to put that knowledge to good use! Remember to pick the right tool for the job:
Use
require
to load external files and libraries into your program.Use
include
to bring in methods and functionality from modules.
Feel free to experiment, play around, and discover the power of both require
and include
in your Ruby projects. And if you have any more questions or need further clarification, drop a comment below or share your thoughts on social media using the hashtag #RubyRequireVsInclude. Let's keep the conversation going! š¬āØ
Happy coding! šš©āš»šØāš»
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
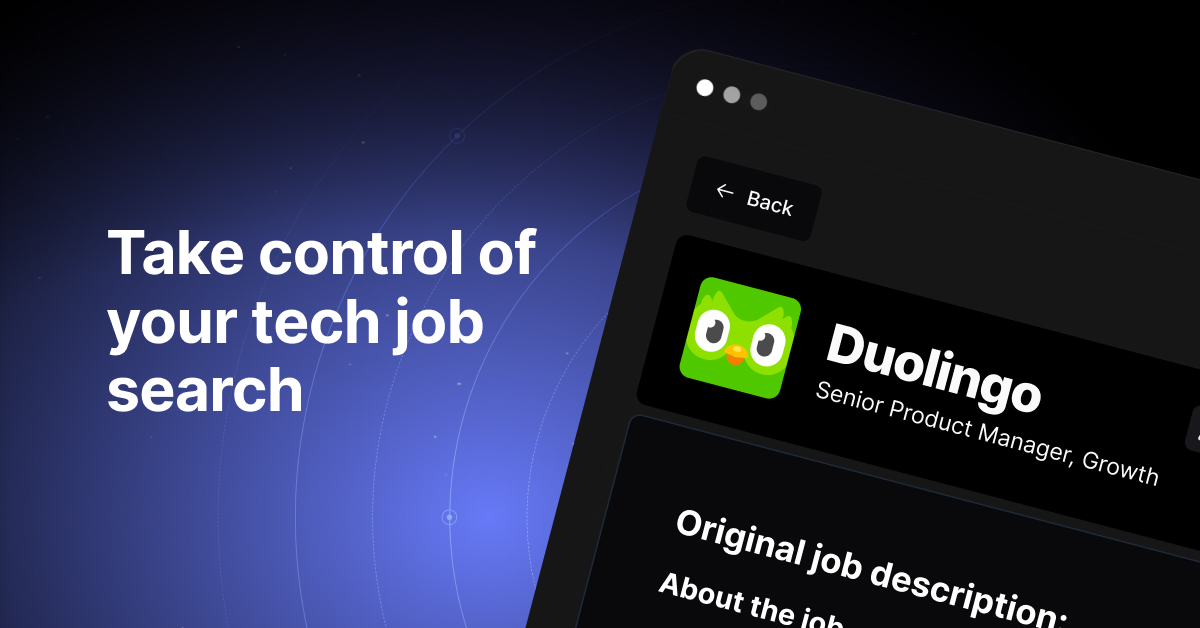