Tell Ruby Program to Wait some amount of time
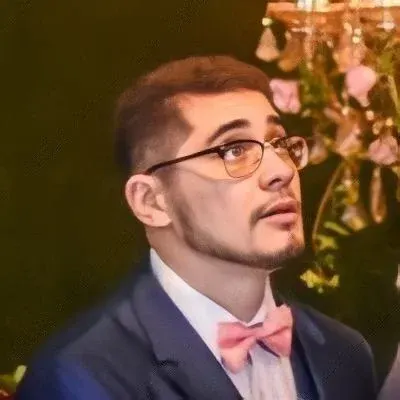
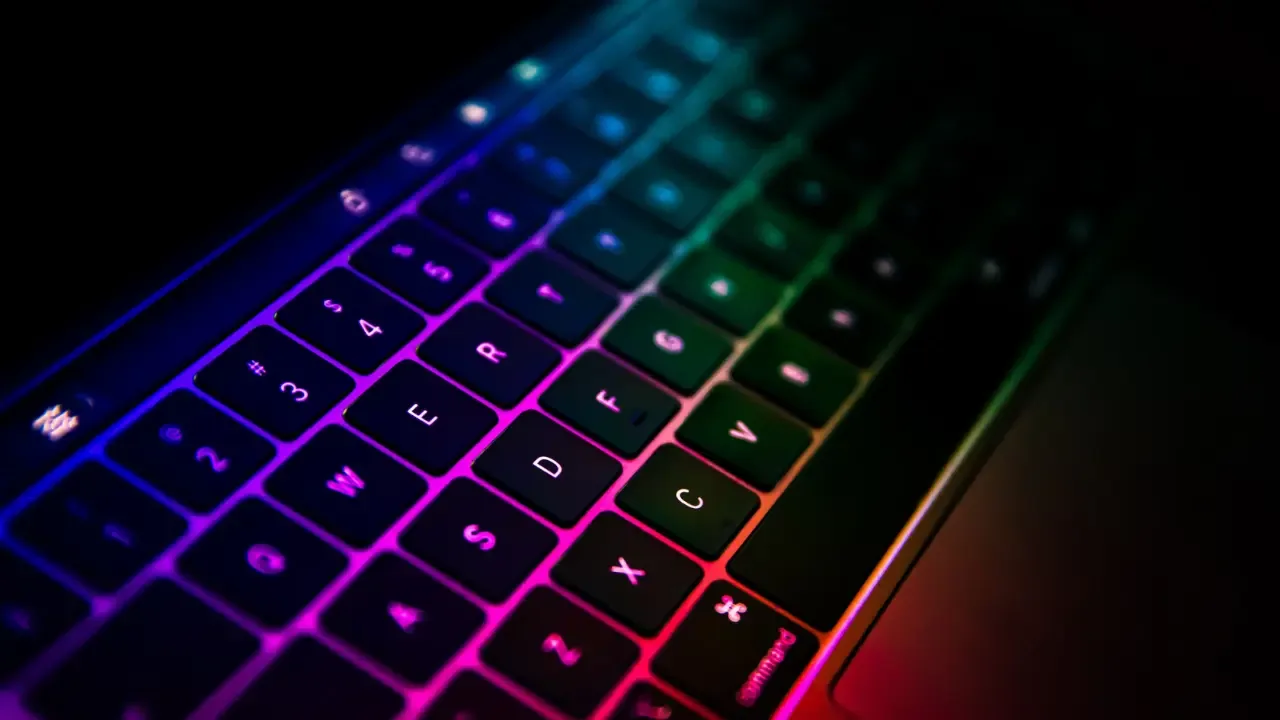
🕒 How to make your Ruby program wait 🕒
So, you want to make your Ruby program pause for a moment and catch its breath before moving on to the next line of code? Well, you've come to the right place! In this blog post, we'll explore some easy solutions to this common problem.
The Problem
Let's say you have a Ruby program and you want it to wait for a specific amount of time before executing the next line of code. Maybe you want to simulate a delay, create a timeout, or simply add a pause for dramatic effect. Whatever the reason, you need a way to pause your program without freezing it entirely.
The Solutions
Fortunately, Ruby provides us with a few handy methods to make our program wait. Here are some of the most common approaches:
1. Sleep Method
The sleep
method is a simple and straightforward way to pause your Ruby program for a specified number of seconds. Just pass the desired delay as an argument, and voilà! Your program will patiently wait before continuing.
puts "Hello!"
sleep(3) # Pauses the program for 3 seconds
puts "Goodbye!"
Output:
Hello!
[3 seconds delay]
Goodbye!
2. Timeout Module
If you're looking to add some time restrictions to your program, the Timeout
module comes to the rescue. It allows you to set a maximum time limit for a particular block of code. If the code takes longer to execute, an exception will be raised, and you can gracefully handle it.
require 'timeout'
begin
Timeout.timeout(5) do
# Code that might take longer than 5 seconds
end
rescue Timeout::Error
puts "Sorry, it took too long!"
end
3. Event-Driven Delay
Sometimes, you need your program to pause until a certain event occurs. In that case, you can leverage the event-driven nature of Ruby to wait for a specific condition to be met. For example, if you're working with a web framework like Ruby on Rails, you can use AJAX requests to wait for data to be loaded before proceeding.
# Code that triggers an AJAX request
until data_loaded? # Wait until data is loaded
sleep(1) # Pause for 1 second before checking again
end
# Code that depends on the loaded data
The Call-to-Action
Now that you know how to make your Ruby program wait, it's time to put your newfound knowledge into practice! Experiment with the different methods we discussed and see how they can enhance the functionality of your programs.
Do you have any other clever ways to make Ruby wait? Share your thoughts and experiences in the comments below! Let's keep the conversation going and help each other become better Ruby developers.
✨ Happy coding! ✨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
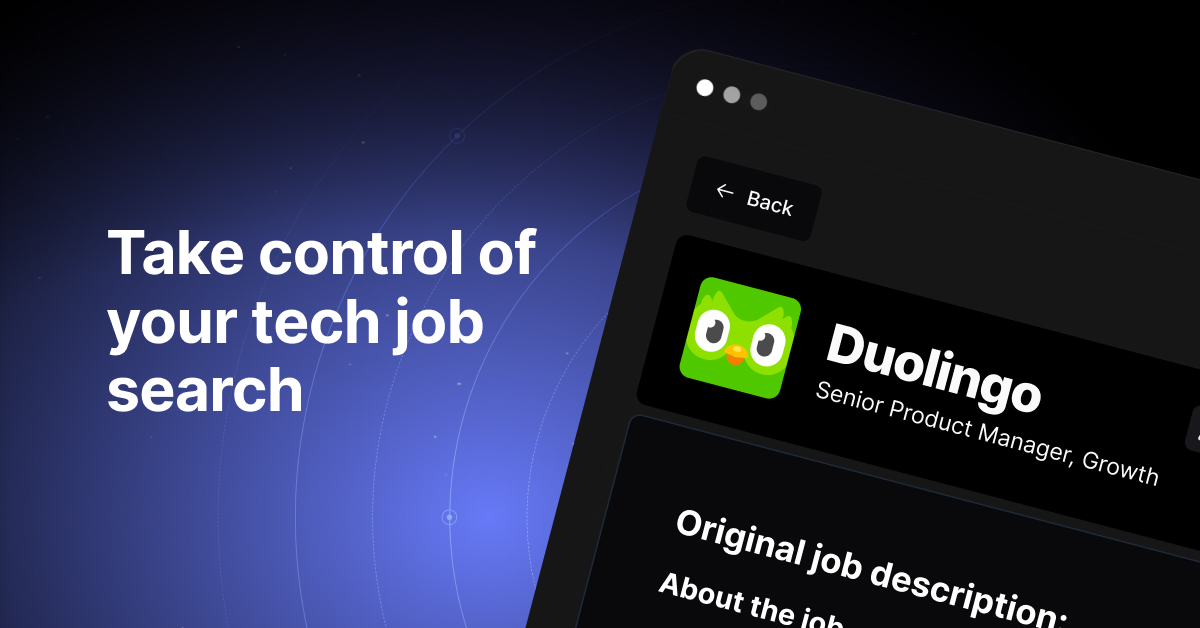