Ruby: How to turn a hash into HTTP parameters?
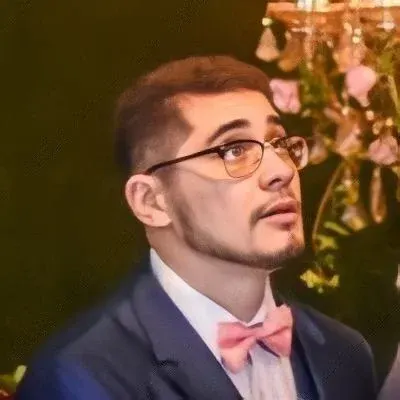
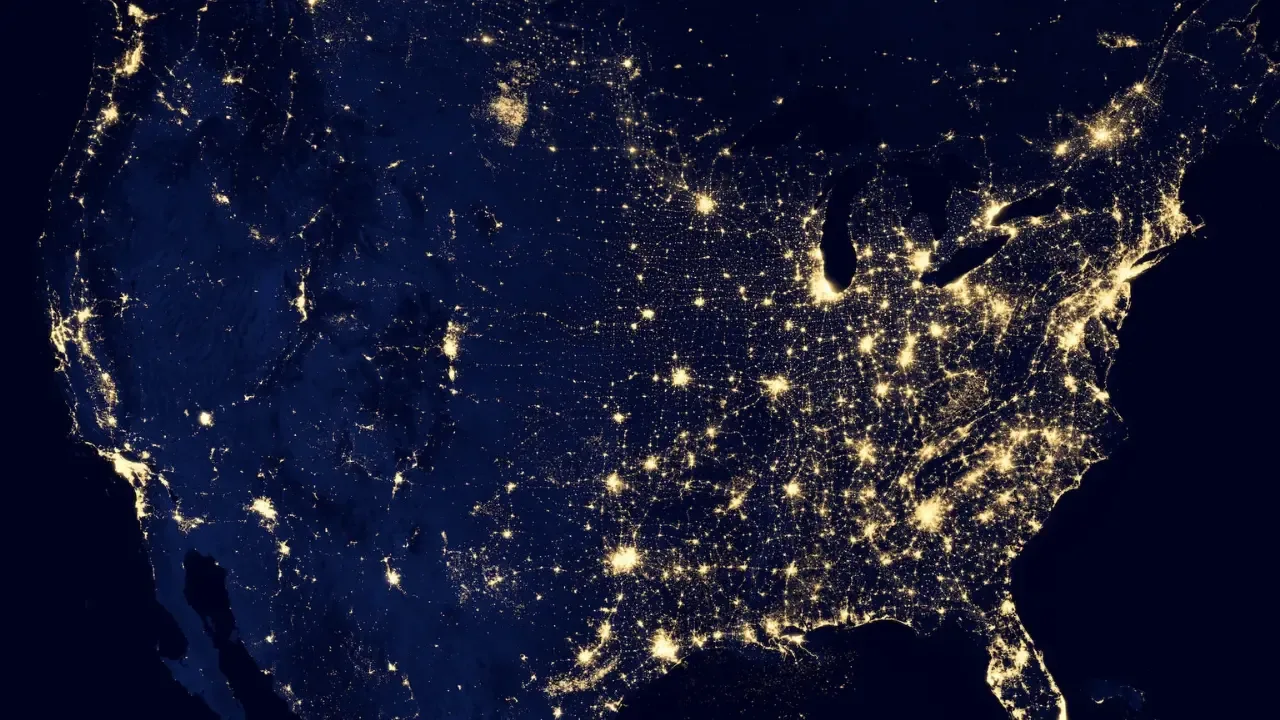
Ruby: Turn a Hash into HTTP Parameters Made Easy! β¨π
Do you find yourself in the tangled web of turning a complex hash into HTTP parameters? Fear not, fellow Rubyist! I am here to guide you through this confusing maze and provide you with easy solutions. Let's dig in! π©βπ»πͺ
The Simple Case: A Plain Hash πΊοΈ
Let's start with a simple scenario. Suppose you have a plain hash like this:
{:a => "a", :b => "b"}
To convert this hash into HTTP parameters, you need to transform it into a string format like this:
"a=a&b=b"
To achieve this, you can use the URI.encode_www_form
method, which is built into Ruby. Here's how you can do it:
require 'uri'
params = {:a => "a", :b => "b"}
encoded_params = URI.encode_www_form(params)
And voila! You have your HTTP parameters string ready to go. π
Handling Arrays in Hashes π
Things get a bit trickier when you have arrays as values in your hash. Consider the following example:
{:a => "a", :b => ["c", "d", "e"]}
In this case, you want to convert it into the following HTTP parameters string:
"a=a&b[0]=c&b[1]=d&b[2]=e"
To accomplish this, you can utilize the to_query
method from the ActiveSupport gem, commonly used in Ruby on Rails applications. If you're not already using Rails, don't worry! You can manually create a helper method to achieve the same functionality:
def array_to_query(key, values)
values.each_with_index.map { |value, index| "#{key}[#{index}]=#{value}" }.join("&")
end
Here's how you can incorporate it into your code:
require 'uri'
params = {:a => "a", :b => ["c", "d", "e"]}
encoded_params = URI.encode_www_form(params.map { |key, value|
if value.is_a?(Array)
array_to_query(key, value)
else
[key, value]
end
})
By utilizing this approach, you can effectively handle arrays within your hash and generate the desired HTTP parameters string.
Nesting Madness: Handling Nested Hashes ππ
Now, let's take things up a notch. Consider a more complex scenario involving nested hashes:
{:a => "a", :b => [{:c => "c", :d => "d"}, {:e => "e", :f => "f"}]}
Converting this nested hash into appropriate HTTP parameters presents a new challenge. We need to flatten the nested structure and generate parameters like this:
"a=a&b[][c]=c&b[][d]=d&b[][e]=e&b[][f]=f"
To tackle this problem, we can enhance our helper method to handle nested hashes as well:
def hash_to_query(key, hash)
hash.map { |nested_key, value| array_to_query("#{key}[][#{nested_key}]", value) }.join("&")
end
Let's incorporate this into our code:
require 'uri'
def hash_to_query(key, hash)
hash.map { |nested_key, value| array_to_query("#{key}[][#{nested_key}]", value) }.join("&")
end
def array_to_query(key, values)
values.each_with_index.map { |value, index| "#{key}[#{index}]=#{value}" }.join("&")
end
params = {:a => "a", :b => [{:c => "c", :d => "d"}, {:e => "e", :f => "f"}]}
encoded_params = URI.encode_www_form(params.map { |key, value|
if value.is_a?(Array)
hash_to_query(key, value)
else
[key, value]
end
})
With this enhanced solution, you can flawlessly handle even the most convoluted nested hashes and generate the desired HTTP parameters string.
Your Turn to Shine! π‘π
Now that you have mastered the art of turning hashes into HTTP parameters in Ruby, go ahead and apply this newfound knowledge to your projects. Experiment and explore further possibilities!
Have you encountered any other challenges related to this topic? How did you solve them? Share your experiences and solutions in the comments below. Let's learn and grow together! ππ±
π’ Call to Action: Share Your Success! π
I hope this guide has helped you unravel the mysteries of turning a hash into HTTP parameters. If you found it useful, don't be shy β share it with your fellow Rubyists! Spread the knowledge by clicking the social media icons below and let the world bask in the glory of your newfound expertise. πβ¨
Until next time, happy coding! π»π
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
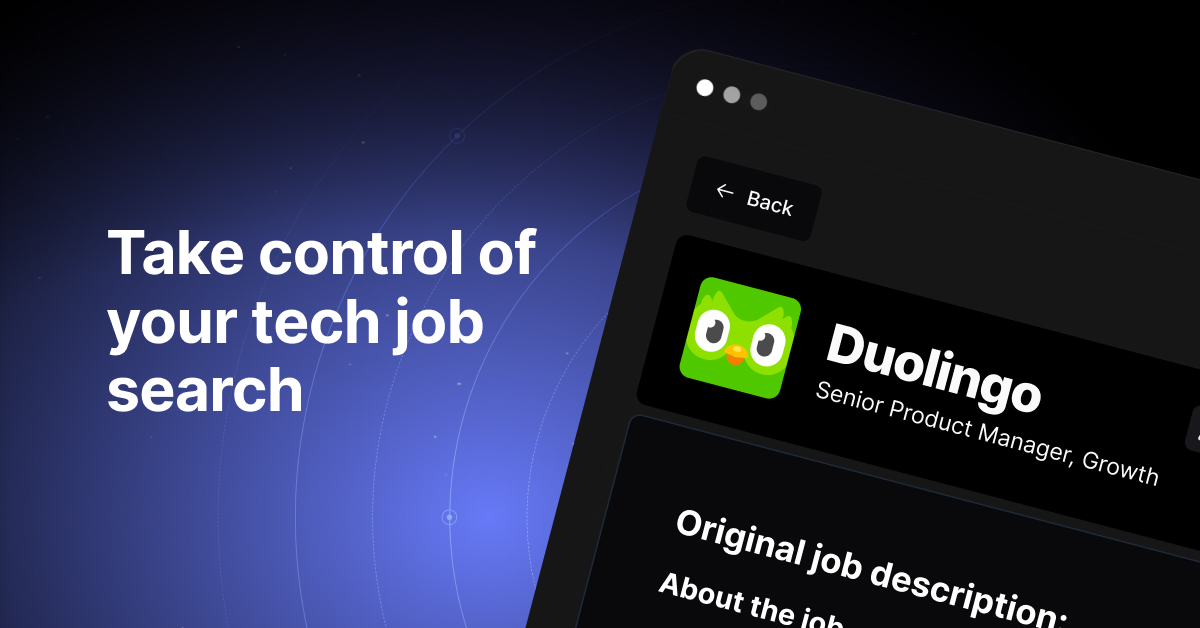