Ruby: Calling class method from instance
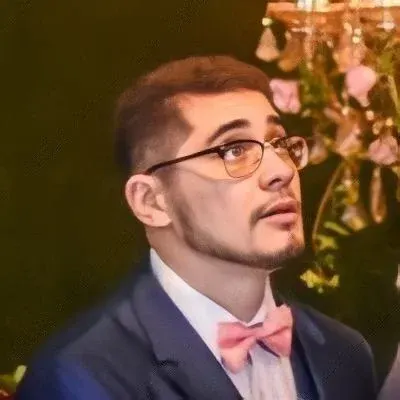
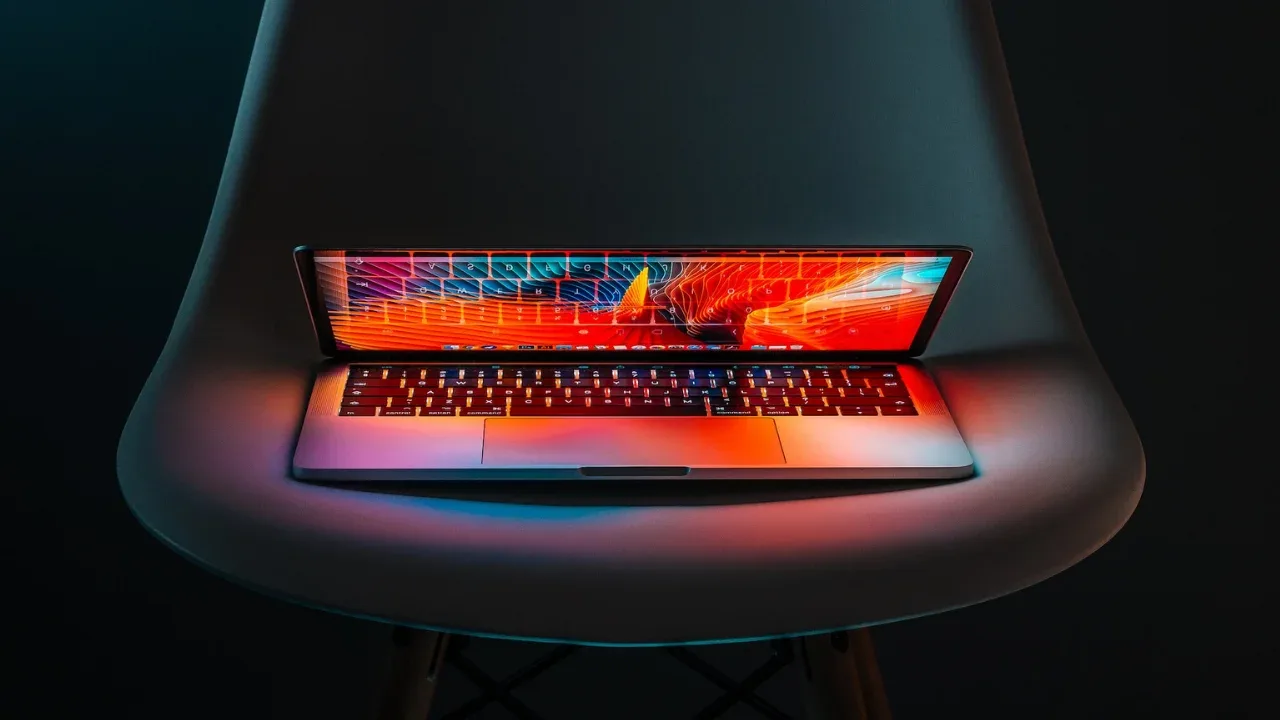
How to Call a Class Method from an Instance in Ruby
Have you ever found yourself needing to call a class method from one of its instances in Ruby? It can be a bit confusing at first, but fear not! In this blog post, we will explore the common issues surrounding this question and provide you with easy solutions.
The Problem
Let's take a look at the code snippet provided:
class Truck
def self.default_make
# Class method.
"mac"
end
def initialize
# Instance method.
Truck.default_make # gets the default via the class's method.
# But: I wish to avoid mentioning Truck. Seems I'm repeating myself.
end
end
As you can see, the line Truck.default_make
is used to retrieve the default make of the truck. But the question is, can we achieve this without explicitly mentioning Truck
? It feels like there should be a more elegant solution.
The Solution
Fortunately, Ruby provides us with a simple solution to this problem. Instead of directly referencing the class name, we can use the class
method available to all objects in Ruby. Let's modify the code to reflect this solution:
class Truck
def self.default_make
# Class method.
"mac"
end
def initialize
# Instance method.
self.class.default_make # gets the default via the class's method using `self.class`.
end
end
By using self.class
, we are able to reference the class of the current instance without explicitly mentioning the class name. This way, you avoid repeating yourself while still achieving the desired result.
Why Does This Work?
In Ruby, every object is an instance of a class. The self
keyword refers to the current instance, and the class
method returns the class of the object. By combining self.class
, we can access the class's methods within an instance method without explicitly mentioning the class name.
Conclusion
Calling a class method from an instance in Ruby doesn't have to be complicated. By utilizing the self.class
approach, you can easily achieve the desired result without repeating yourself. So the next time you find yourself needing to call a class method from an instance, remember this handy solution!
If you found this blog post helpful, why not share it with your fellow Ruby developers? Let them benefit from this time-saving technique as well. And don't forget to leave a comment below if you have any questions or further insights on this topic!
Happy coding! 👩💻🚀
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
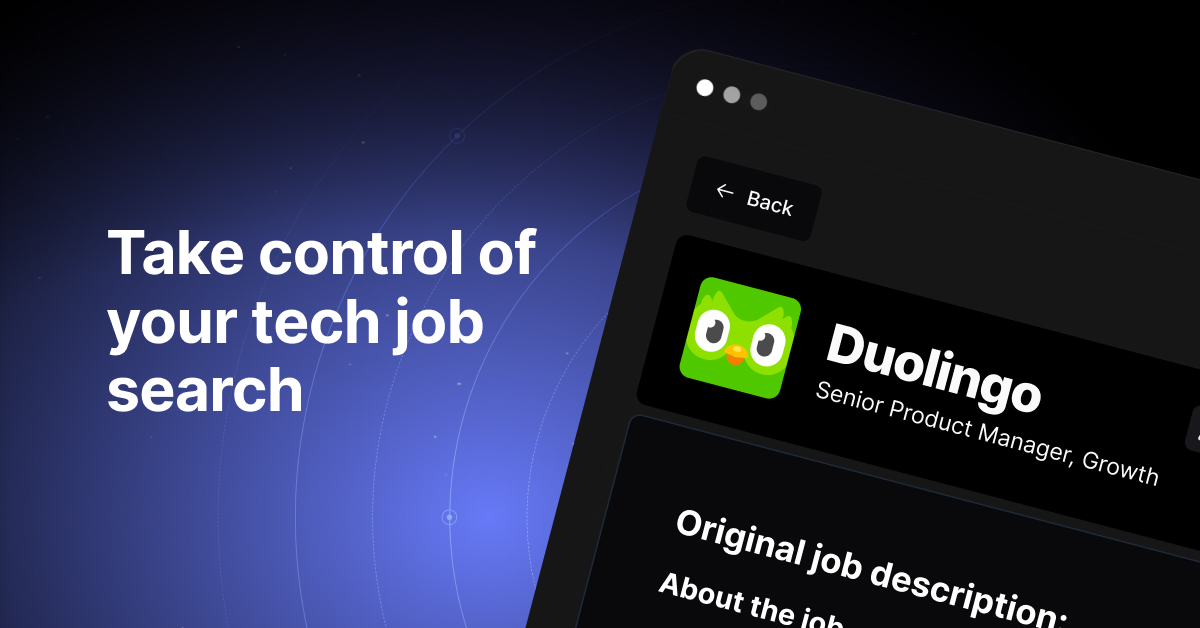