Parsing a JSON string in Ruby
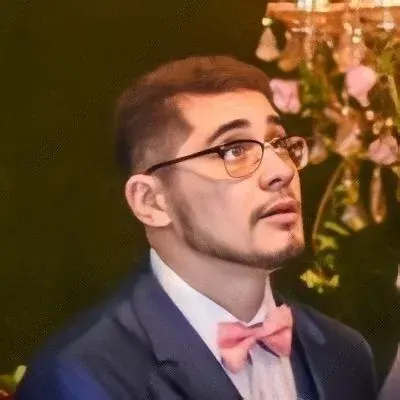
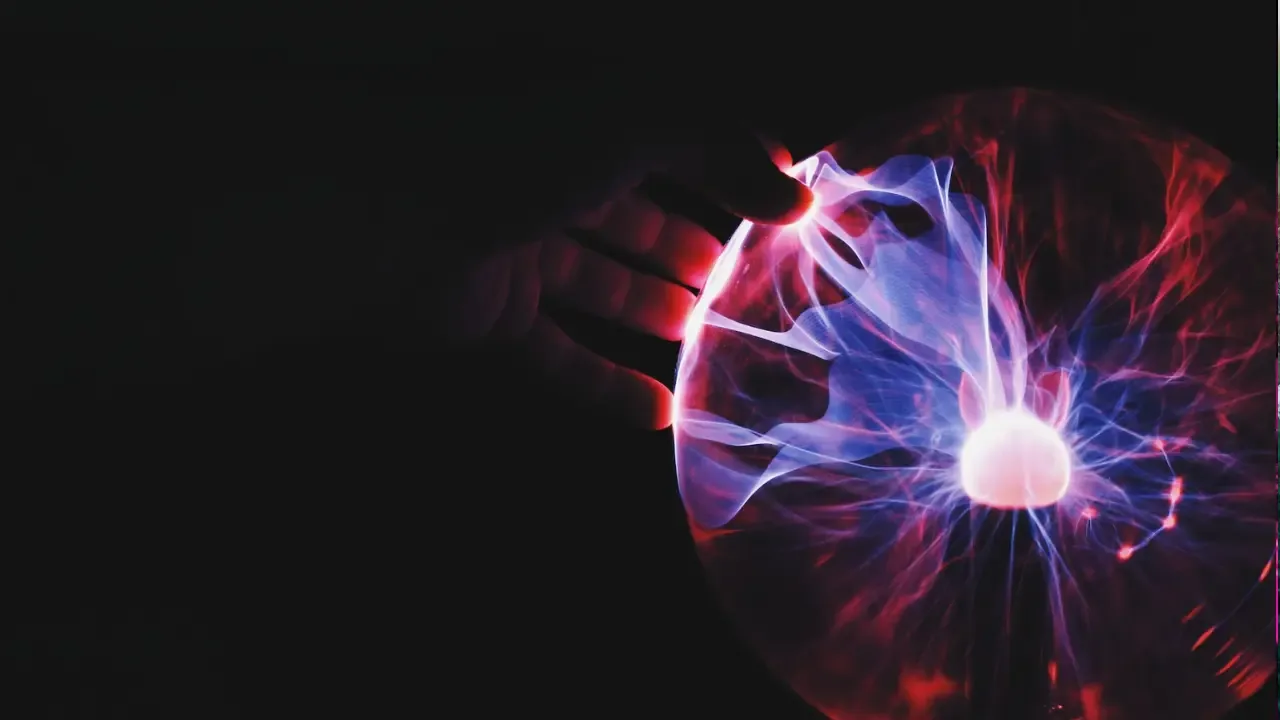
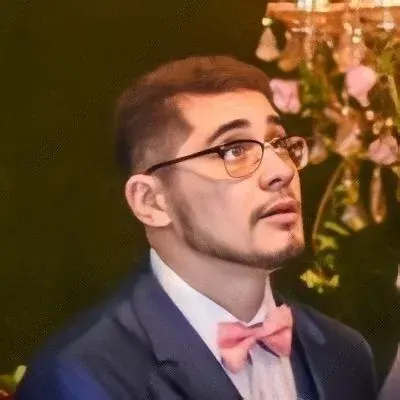
How to Parse a JSON string in Ruby
Parsing a JSON string in Ruby can seem like a daunting task, but fear not! We're here to guide you through the process step-by-step. 🚀
The Problem
Let's say you have a string containing a JSON object, and you want to extract the data from it. In our case, the string looks like this:
string = '{"desc":{"someKey":"someValue","anotherKey":"value"},"main_item":{"stats":{"a":8,"b":12,"c":10}}}'
The question is, how can we easily parse this string to access the desired data?
The Solution
Ruby provides a built-in library called JSON
that allows us to parse JSON strings effortlessly. Here's how you can do it:
require 'json'
json_object = JSON.parse(string)
The JSON.parse
method converts the JSON string into a Ruby hash, making it incredibly easy to access the data. 🙌
Extracting the Data
Now that we have parsed our JSON string, we can easily navigate through the resulting hash to extract the desired data. In our example, let's say we want to get the value of the "someKey"
:
value = json_object['desc']['someKey']
That's it! You've successfully extracted the data from the JSON string. 💪
Common Issues
Invalid JSON
One common issue you might encounter is trying to parse an invalid JSON string. If the string is not properly formatted, the JSON.parse
method will raise a JSON::ParserError
. To handle this gracefully, you can wrap the parsing code in a begin...rescue
block:
begin
json_object = JSON.parse(string)
# The rest of your code goes here
rescue JSON::ParserError => e
puts "Invalid JSON: #{e.message}"
end
Missing Keys
Another common issue is trying to access keys that don't exist in the JSON string. If you attempt to access a non-existent key, Ruby will return nil
. To handle this situation, you can use the safe navigation operator (&.
) to avoid NoMethodError
:
value = json_object&.dig('desc', 'someMissingKey')
Take it to the Next Level!
Now that you know how to parse a JSON string in Ruby, why not take it a step further? Use this knowledge to build web applications that consume APIs, process data, and much more. The possibilities are endless! 🌈
Conclusion
Parsing a JSON string in Ruby is a common task, but with the help of the JSON
library, it becomes a breeze. Remember to handle invalid JSON and missing keys gracefully, and you'll be well on your way to extracting data like a pro.
So go ahead, give it a try! Share your thoughts and experiences with us in the comments below. Happy coding! 💻✨