What is the right way to override a setter method in Ruby on Rails?
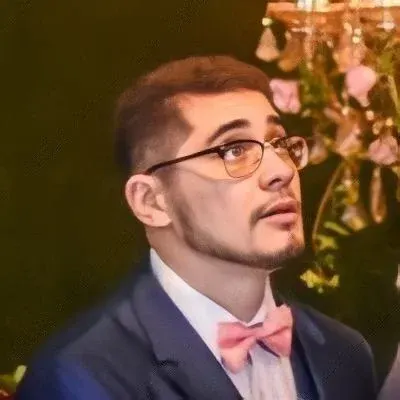
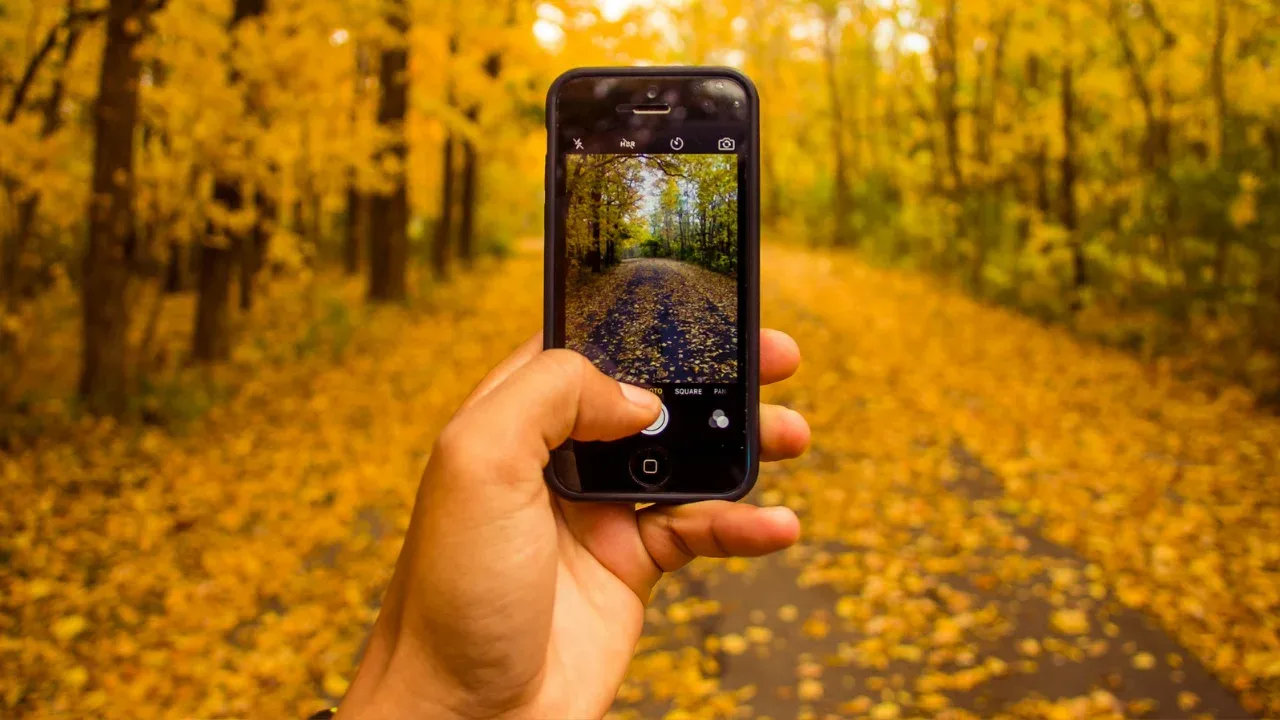
📝 Blog Post: What is the right way to override a setter method in Ruby on Rails?
Are you a Ruby on Rails developer looking for the right way to override a setter method? Look no further! In this blog post, we'll discuss the common issues, easy solutions, and provide you with the proper way to override a setter method in Ruby on Rails. Let's dive right in!
🔍 Understanding the Problem
To better understand the problem, let's take a look at the code snippet provided by the user:
attr_accessible :attribute_name
def attribute_name=(value)
... # Some custom operation.
self[:attribute_name] = value
end
The above code seems to work as expected. However, the user wants to ensure if this is the right way to override a setter method and if any future problems might arise. Great question!
🐛 Potential Issues
By using the code snippet above, you may encounter the following potential issues:
1️⃣ SystemStackError: If you attempt to use self.attribute_name = value
instead of self[:attribute_name] = value
, you might face a SystemStackError (stack level too deep) as mentioned in the user's note.
🛠️ The Right Way to Override a Setter Method
To override a setter method correctly, you can use the write_attribute
method instead of directly assigning the value to self[:attribute_name]
. Here's how you can do it:
def attribute_name=(value)
... # Some custom operation.
write_attribute(:attribute_name, value)
end
By using write_attribute
, you ensure that the setter method is properly overridden, and you avoid potential issues such as the SystemStackError.
📣 Take Action and Engage!
Now that you know the right way to override a setter method, it's time to put your knowledge into action! Try implementing the suggested code snippet in your Ruby on Rails project and see the difference it makes.
We'd love to hear about your experience and any further questions you might have. Share your thoughts in the comments section below! Let's engage and learn from each other.
Happy coding! 💻✨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
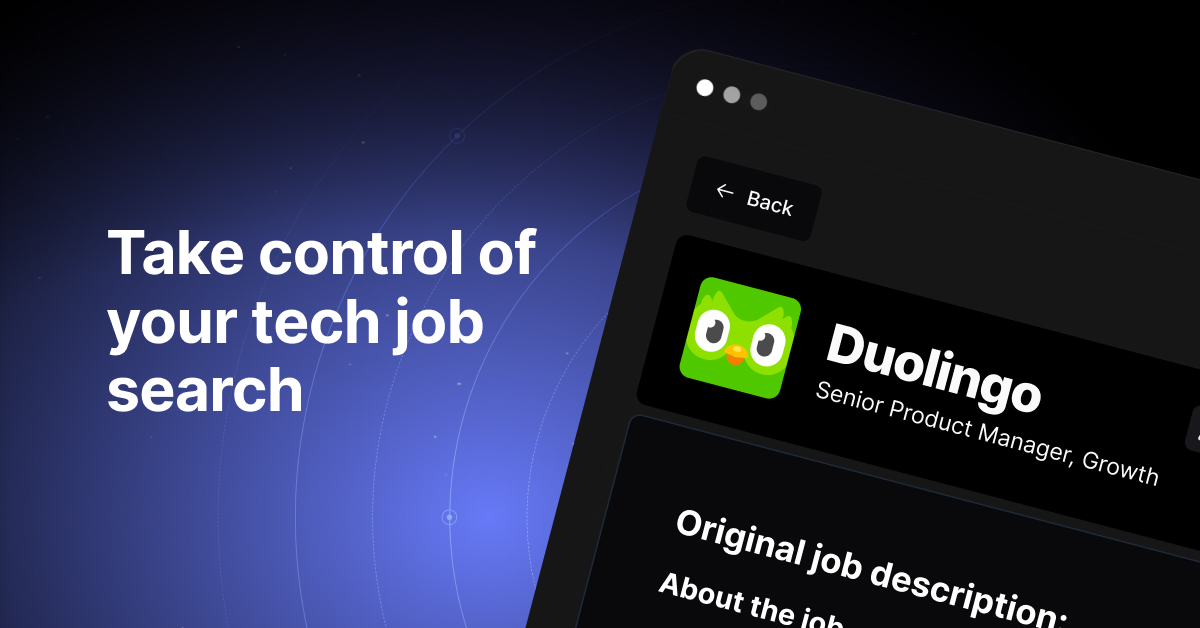