What do helper and helper_method do?
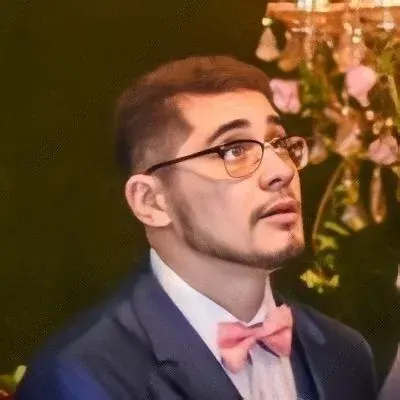
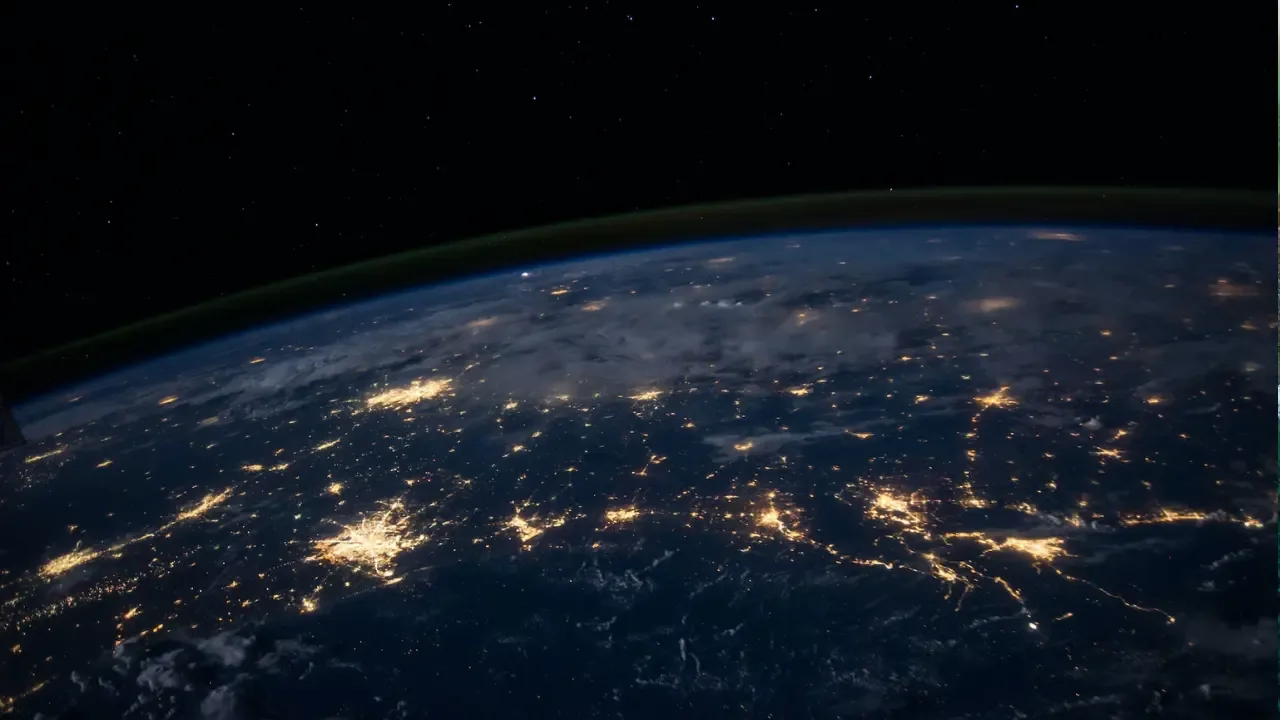
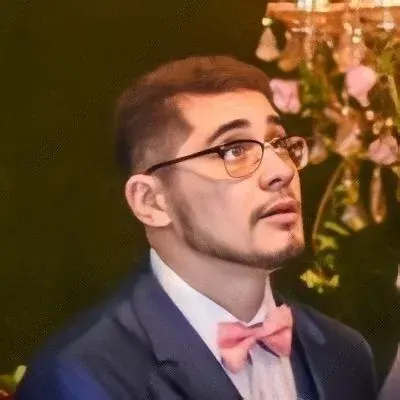
What do helper
and helper_method
do?
When working with Ruby on Rails, you may come across the terms helper
and helper_method
. These two methods are used to share functionality between controllers and views, making your code more DRY (Don't Repeat Yourself) and easier to maintain. In this blog post, we will explore what these methods do, their differences, and how to use them effectively.
Understanding helper
The helper
method in Rails is used to import helper methods into a particular file or a module. These helper methods can be defined in the ApplicationHelper
module or any other custom helper modules that you create within your Rails application.
The purpose of using a helper method is to encapsulate logic and reusable code that can be used across multiple views. By importing these methods using the helper
method, you make them available to the specific view or views where you need them.
Let's say you have a DateHelper
module with a method called format_date
, which formats a date string in a specific format. In your view, you can import this method using the helper
method like this:
class UsersController < ApplicationController
helper DateHelper
def show
# ...
end
end
Now, the format_date
method from the DateHelper
module is accessible within the show
view of the UsersController
. You can simply call format_date
without explicitly referencing the module name.
Exploring helper_method
On the other hand, the purpose of the helper_method
in Rails is slightly different from the helper
method. Instead of importing helper methods into a specific file or module, the helper_method
makes some or all of the controller's methods available to the view.
In other words, the helper_method
is used to expose controller methods to the view layer, enabling you to call these methods directly within your view templates. This is especially useful when you have some complex logic or calculations in the controller that you want to access from the view.
To define a helper method in a controller, you can use the helper_method
macro like this:
class UsersController < ApplicationController
helper_method :current_user
def show
# ...
end
private
def current_user
@current_user ||= User.find(params[:id])
end
end
In the above example, the current_user
method is defined as a helper method using helper_method
. Now, you can call current_user
directly within your view templates, without having to go through the controller explicitly.
Simplicity in Naming
You may wonder why these methods are named helper
and helper_method
. Indeed, the names can be a bit confusing and may lead to misconceptions. A more intuitive naming could possibly be share_methods_with_view
and import_methods_from_view
, respectively.
While it is essential to understand their functionality, the naming convention provides a hint about their purpose and usage in the Rails framework.
Conclusion
In this blog post, we have explored the differences between helper
and helper_method
in Ruby on Rails. While helper
imports helper methods into a file or a module, helper_method
exposes controller methods to the view. Understanding when to use each method is crucial for writing cleaner code and improving code reusability.
By leveraging these methods effectively, you can make your code more readable, maintainable, and DRY. So go ahead, experiment with them, and let us know how they have helped you in your Rails projects.
If you still have any questions or want to share your thoughts, leave a comment below. Happy coding! 💻🚀
References: