Want to find records with no associated records in Rails
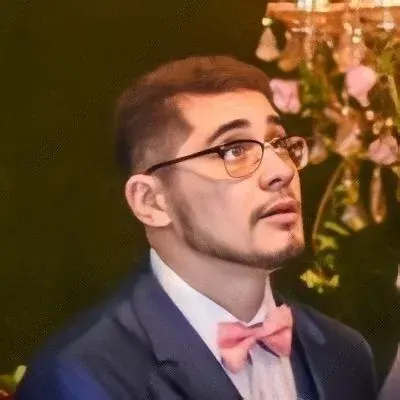
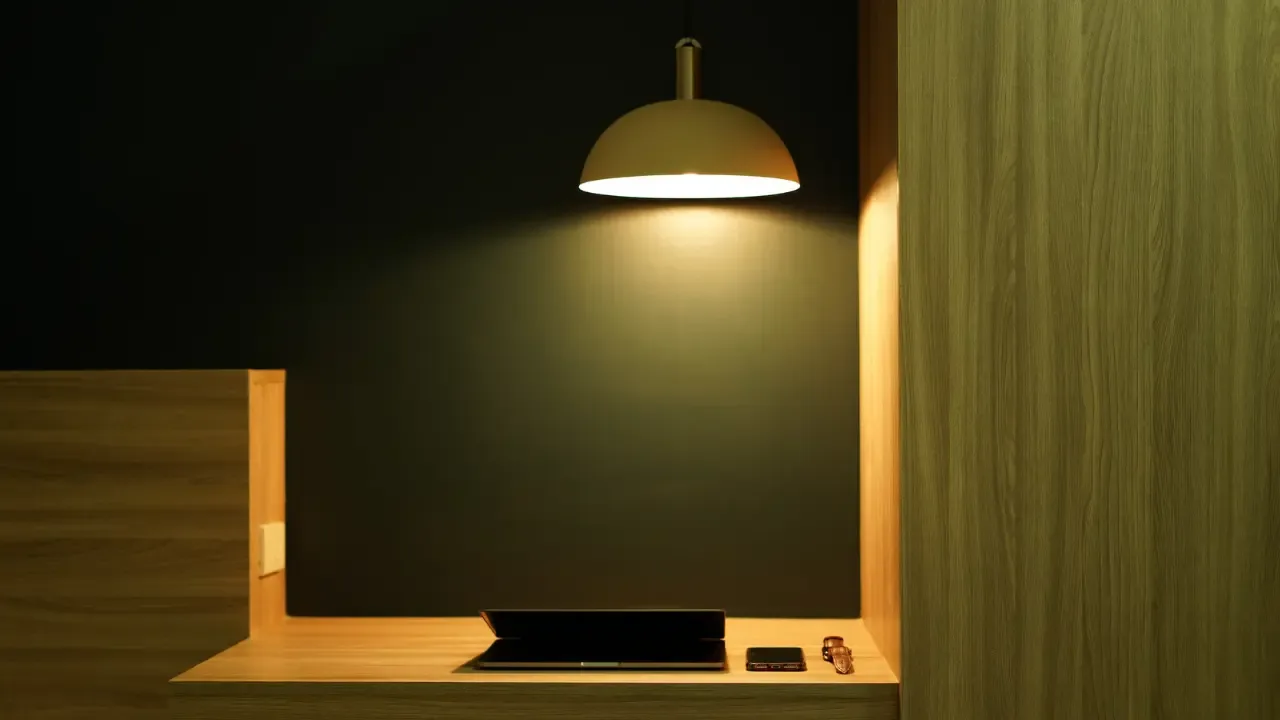
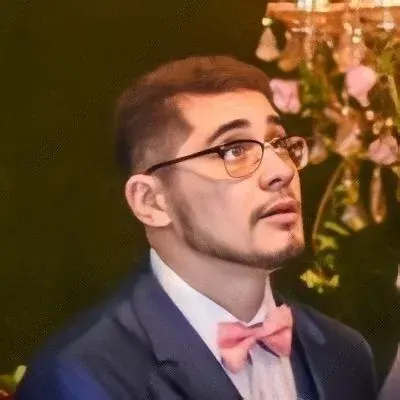
📝 Finding Records with No Associated Records in Rails 🎯
Are you struggling to find records in Rails that have no associated records? No worries, we've got you covered! In this post, we'll explore the cleanest and most efficient ways to accomplish this using ARel and/or meta_where. 🚀
The Context 👥
To set the stage, let's consider a simple association between the Person
and Friend
models. A Person
can have multiple friends, while a Friend
belongs to a single person. Here's a quick look at how these models are defined:
class Person
has_many :friends
end
class Friend
belongs_to :person
end
Finding Persons with No Friends 🤝
The first case we'll tackle is finding all the persons that have no friends. This can be achieved by leveraging the power of ActiveRecord queries. Let's dive into the cleanest solution using ARel! 💪
Person.where.not(id: Friend.select(:person_id))
In this query, we use a subquery to select all the person_id
values from the friends
table. The where.not
method then returns all the Person
records whose id
does not appear in the subquery result. Elegant and efficient! 🌟
But hey, what about a has_many :through
association? Don't worry, we've got a solution for that too! 🙌
Finding Persons with No Friends in a has_many :through
Association 🔄
Now, let's consider a more complex scenario. We'll introduce a Contact
model that acts as a join table between Person
and Friend
. Here's a glimpse of the updated models:
class Person
has_many :contacts
has_many :friends, through: :contacts, uniq: true
end
class Friend
has_many :contacts
has_many :people, through: :contacts, uniq: true
end
class Contact
belongs_to :friend
belongs_to :person
end
To find all the persons with no friends in this has_many :through
association, we can tweak our previous solution slightly:
Person.where.not(id: Contact.select(:person_id).map(&:id))
Similar to before, we extract the person_id
values from the contacts
table using a subquery. Then, we select all Person
records whose id
is not included in the extracted IDs. Beautifully simple and effective! 🎉
The Call to Action 📣
Now that you have learned how to find records with no associated records in Rails, it's time to put this newfound knowledge into action! Use these techniques to optimize your queries and efficiently filter your data. Share this blog post with your fellow Rails developers to spread the knowledge and help them solve this common problem. 💡
We hope this guide has been helpful in your journey to becoming a Rails pro! If you have any questions or suggestions, feel free to drop a comment below. Happy coding! 💻❤️
(Note: Remember to use the appropriate method syntax based on your Rails version and the query builder gem you are using, such as meta_search
. The examples provided here are based on ActiveRecord and may need slight adjustments to adapt to your specific setup.)