Validate uniqueness of multiple columns
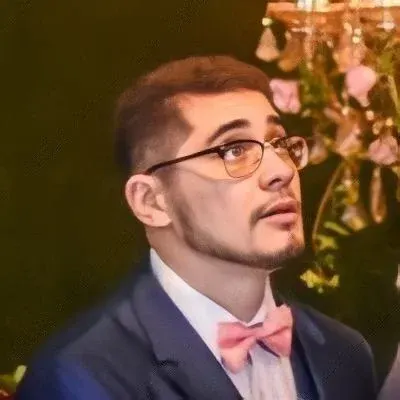

Validate Uniqueness of Multiple Columns: A Rails Solution 💎
Have you ever wondered how to ensure the uniqueness of multiple columns in your Rails application? 🤔 It's not uncommon to encounter situations where you need to validate that a record is unique based on more than just a single column. In this blog post, we'll tackle a specific problem related to validating the uniqueness of multiple columns in Rails and provide you with some easy solutions. So, let's get started! 🚀
The Challenge: Ensuring Unique Records with Multiple Columns 🔒
Consider a scenario where you have a friendship model/table in your Rails application. You want to validate that each friendship record is unique, taking into account both the user_id
and friend_id
columns. You don't want to allow duplicate records where both these columns have identical values.
For example, these records should not be allowed:
user_id: 10 | friend_id: 20
user_id: 10 | friend_id: 20
The Solution: Rails' Uniqueness Validation with a Twist ✨
Fortunately, Rails provides a built-in uniqueness
validation, which is commonly used to ensure the uniqueness of a single column. To make it work for multiple columns, we just need to add a small twist. 😉
Solution 1: Using scope
with validates_uniqueness_of
One way to achieve the desired uniqueness validation is by using the scope
option in conjunction with validates_uniqueness_of
in your friendship model.
class Friendship < ApplicationRecord
validates_uniqueness_of :user_id, scope: :friend_id
end
With this simple addition, Rails will take both the user_id
and friend_id
columns into account when checking for uniqueness. If an attempt is made to create a duplicate friendship record, Rails will automatically reject it, ensuring that each friendship is unique.
Solution 2: Using a Composite Unique Index
Another approach to tackling this problem is by utilizing a composite unique index at the database level. This ensures that the combination of user_id
and friend_id
values remains unique and avoids the need for additional validation in the application layer.
Assuming you're using PostgreSQL as your database, you can create a migration to add the composite unique index:
class AddUniqueIndexToFriendships < ActiveRecord::Migration
def change
add_index :friendships, [:user_id, :friend_id], unique: true
end
end
By adding this unique index, duplicate records with the same combination of user_id
and friend_id
will be prevented at the database level itself. This is a powerful solution that not only guarantees uniqueness but also improves performance by offloading the validation task to a lower level.
Time to Take Action: Share Your Thoughts! 💬
Now that you have two practical solutions to validate uniqueness across multiple columns in Rails, it's time to put them into action! Whether you decide to implement the validates_uniqueness_of
solution or utilize a composite unique index, we'd love to hear your thoughts and experiences with these approaches.
Have you encountered this challenge before? What other unique scenarios have you come across while developing in Rails? Share your insights and engage with our vibrant developer community in the comments section below! Let's keep the discussion going! 🎉
Happy coding! 😄✌️
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
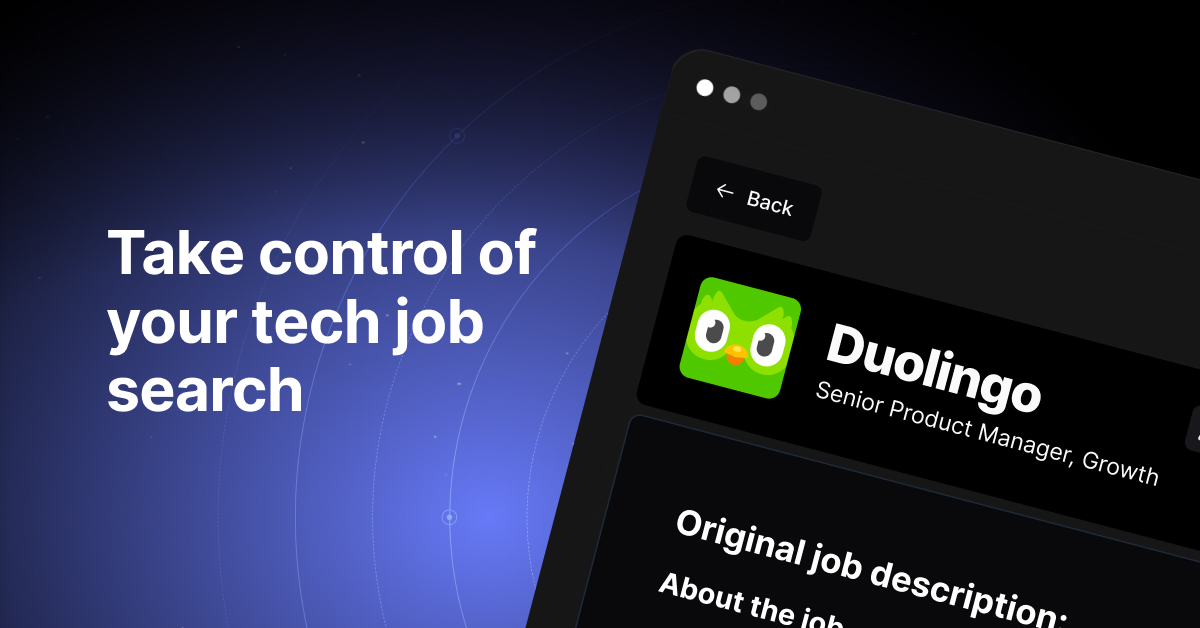