Ruby/Rails: converting a Date to a UNIX timestamp
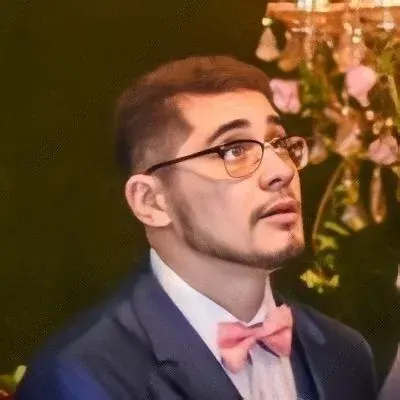
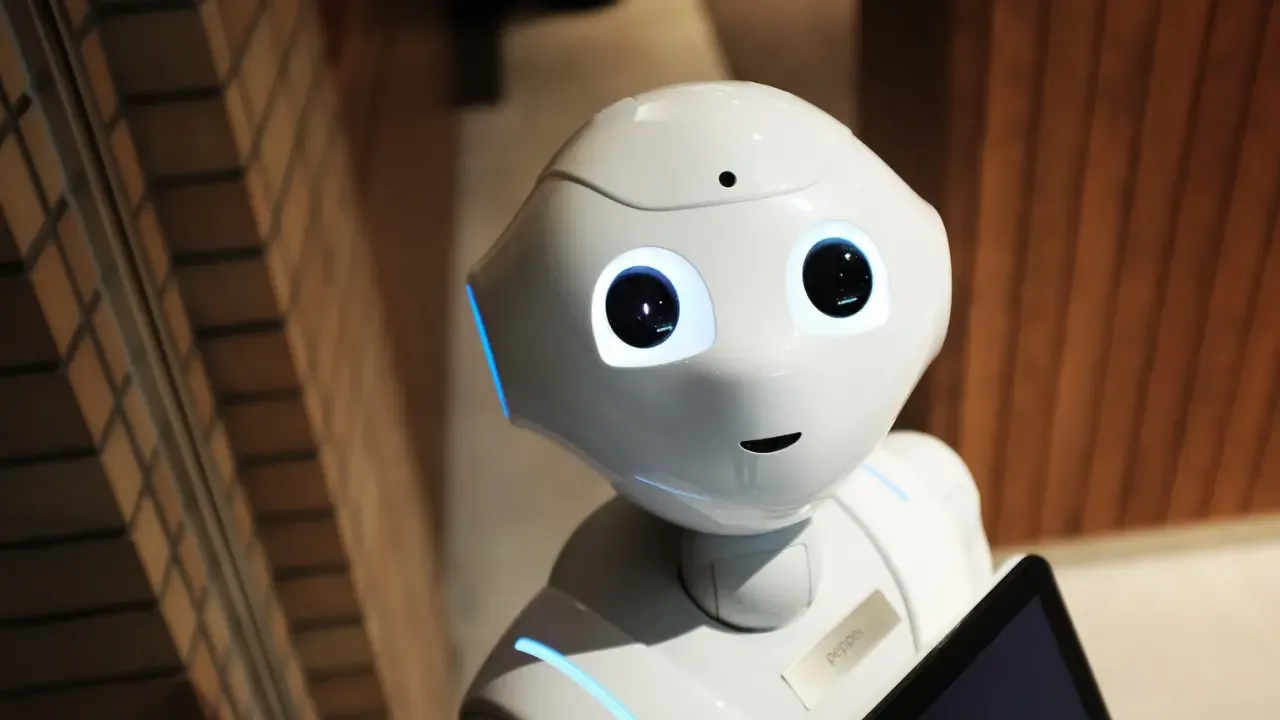
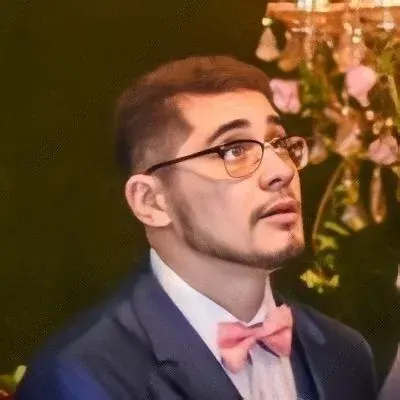
🎉📝 Converting a Date to a UNIX Timestamp in Ruby/Rails: Issue and Easy Solutions 🖥🚀
Are you looking to convert a Date object into a UNIX timestamp in your Ruby on Rails app? You've come to the right place! In this blog post, we'll address the common issue faced by many developers and provide you with easy solutions to tackle it. Let's dive in! 💡👇
The Problem: Off by About a Month 📅❌
One user on a Rails forum shared that they were experiencing an issue when trying to obtain a UNIX timestamp from a Date object. They noticed that using Time#to_i
didn't give them the expected result. Instead, when they used Date#to_time
to convert the Date object to a Time object and then obtained the timestamp, it was off by approximately a month. 😮
Understanding the Issue 🕵️♂️
The problem lies in a time zone mismatch that occurs when converting the Date object to a Time object using Date#to_time
. This mismatch can sometimes cause the timestamp to be calculated incorrectly, resulting in a discrepancy of about a month.
Easy Solution: Accounting for Time Zone Mismatch ✅🌐
If you want to stick with using Date#to_time
for the conversion and adjust for the time zone mismatch, you can specify the desired time zone by using the in_time_zone
method. Here's an example:
date = Date.parse("2022-01-01")
time = date.to_time.in_time_zone("UTC")
timestamp = time.to_i
By specifying the time zone as "UTC" using in_time_zone
, you ensure that the timestamp remains accurate regardless of any time zone mismatches that may occur during the conversion. Problem solved! 🎉
Alternative Solution: Leveraging DateTime 💪✨
If you prefer to avoid relying on Date#to_time
, you can also use the DateTime class to achieve the desired result. Here's how you can do it:
require 'date'
date = Date.parse("2022-01-01")
timestamp = DateTime.new(date.year, date.month, date.day).to_time.to_i
By using the DateTime class and manually specifying the year, month, and day, you bypass the potential time zone mismatch issue and obtain an accurate UNIX timestamp. Voila! 🚀
Join the Conversation and Share Your Experience! 💬🔗
Do you have any other tips or tricks for converting a Date to a UNIX timestamp in Ruby/Rails? We'd love to hear from you! Leave a comment below and share your insights with our community. Let's help each other grow as developers! 🌟
Remember, no problem is too big when we tackle it together! Happy coding! 💻💪