Ruby on Rails: Where to define global constants?
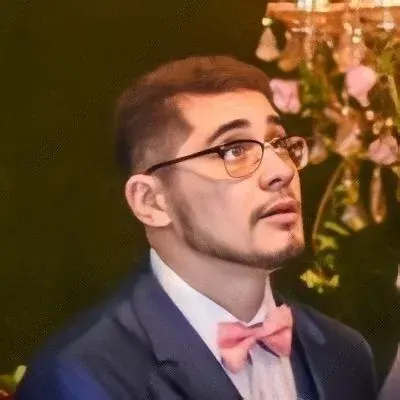
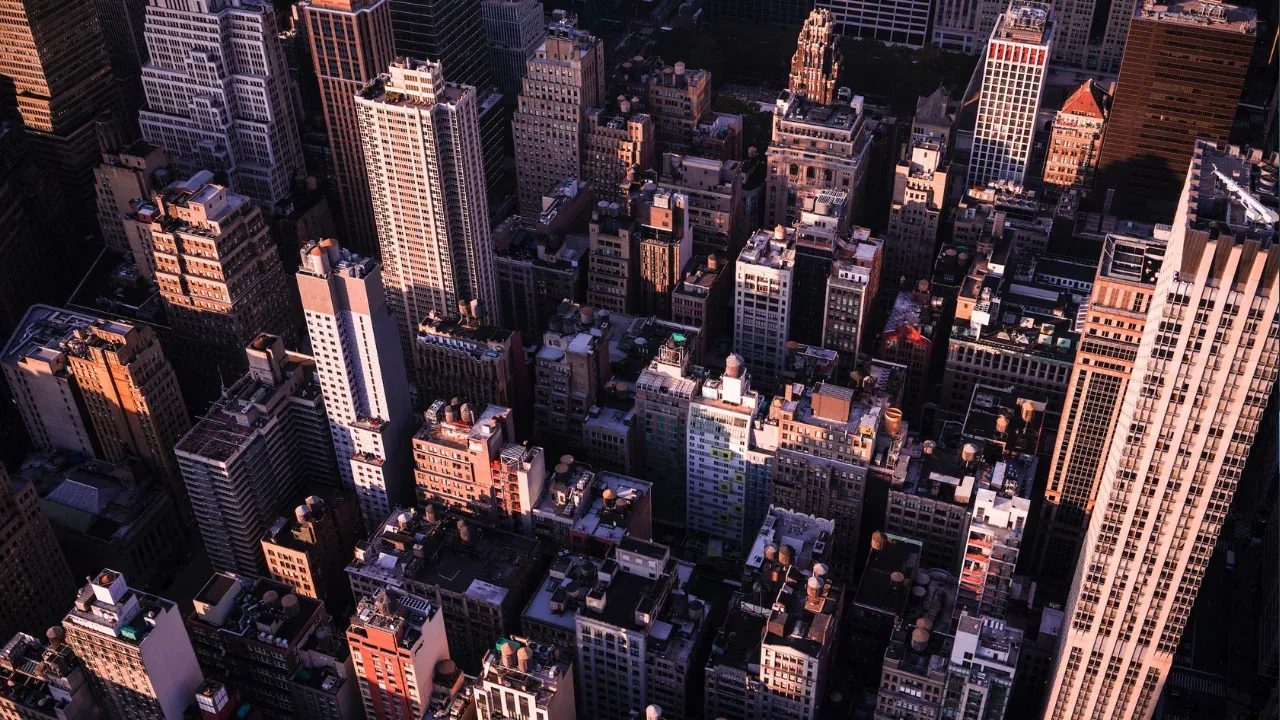
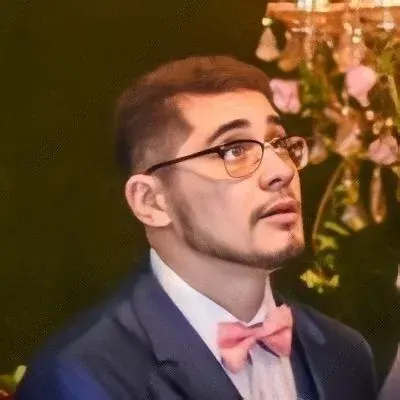
📢 Ruby on Rails: Where to define global constants? 🌐
So you're diving into the world of Ruby on Rails and you've run into a little conundrum - where to define global constants that can be accessed across your entire app? 🤔 Don't worry, we've got you covered! In this blog post, we'll explore common issues and provide easy solutions to help you out. Let's get started! 💪
The Scenario 🏢
Imagine you have a Ruby on Rails web app with various models, views, and controllers. You need to define global constants that are applicable throughout your whole application, both in the logic of your models and the decisions made in your views. For instance, let's say you want to define a constant called COLOURS
with values 'white', 'blue', 'black', 'red', and 'green'.
The Challenge ⚔️
You've tried a few approaches, but nothing seems to be working seamlessly. Here's what you've attempted so far:
1️⃣ Constant class variables in the corresponding model (e.g., @@COLOURS = [...]
), but accessing them in views requires a kludgy syntax like Card.first.COLOURS
.
2️⃣ A method on the model (e.g., def colours ['white',...] end
), but you encountered the same issue.
3️⃣ A method in application_helper.rb
- which has worked for views, but unfortunately, it's inaccessible in models.
4️⃣ You may have even tinkered with application.rb
or environment.rb
, but those solutions didn't seem right, and they didn't resolve the problem either.
Finding the Sweet Spot 🎯
Believe it or not, there is a way to define global constants accessible from both models and views without sacrificing the separation between them. Here's what you can do:
1️⃣ Go to your config/initializers
directory and create a new file, let's call it constants.rb
. This folder is specifically designed for initializing constants and configurations.
2️⃣ Open constants.rb
and define your global constant using the Ruby ::
scope resolution operator. For example:
module AppConstants
COLOURS = ['white', 'blue', 'black', 'red', 'green']
end
3️⃣ Save the file and restart your Rails server for the changes to take effect.
4️⃣ Now, you can access the COLOURS
constant from both models and views using the AppConstants::COLOURS
syntax. 🌈
# Example usage in a model:
class Card < ApplicationRecord
def some_method
colors = AppConstants::COLOURS
# your code here
end
end
# Example usage in a view:
<% AppConstants::COLOURS.each do |color| %>
<div style="background-color: <%= color %>">
<!-- your code here -->
</div>
<% end %>
See? Easy-peasy! Now you have a DRY and accessible solution for your global constants. 🔥
The Importance of Separation 🚧
While it's tempting to mix models and views when they share domain-specific knowledge, it's crucial to maintain separation for the sake of code maintainability and modularity. By utilizing the constants.rb
file, you maintain the separation and keep your codebase organized.
Let's Recap 🔍
Defining global constants in Ruby on Rails can be a bit tricky, especially when you want them accessible from both models and views.
Avoid using class variables, methods, or helpers that are limited to either models or views.
Instead, create a
constants.rb
file in theconfig/initializers
directory.Define your global constants using the
::
scope resolution operator within a module.Access the constants using the
ModuleName::CONSTANT_NAME
syntax.
Engage and Share! 📣
We hope this blog post has shed some light on the best practices for defining global constants in Ruby on Rails. If you have any questions or alternative solutions, we'd love to hear from you in the comments section below. Share this post with your fellow RoR enthusiasts who might be facing the same challenge.
Happy coding! 💻✨