Ruby on Rails - Import Data from a CSV file
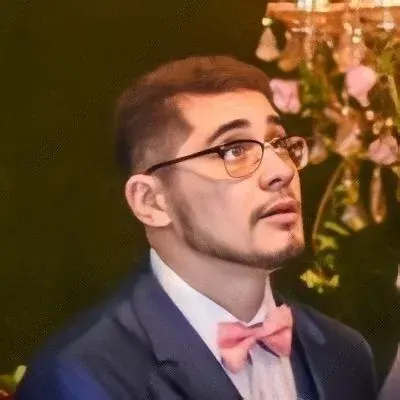
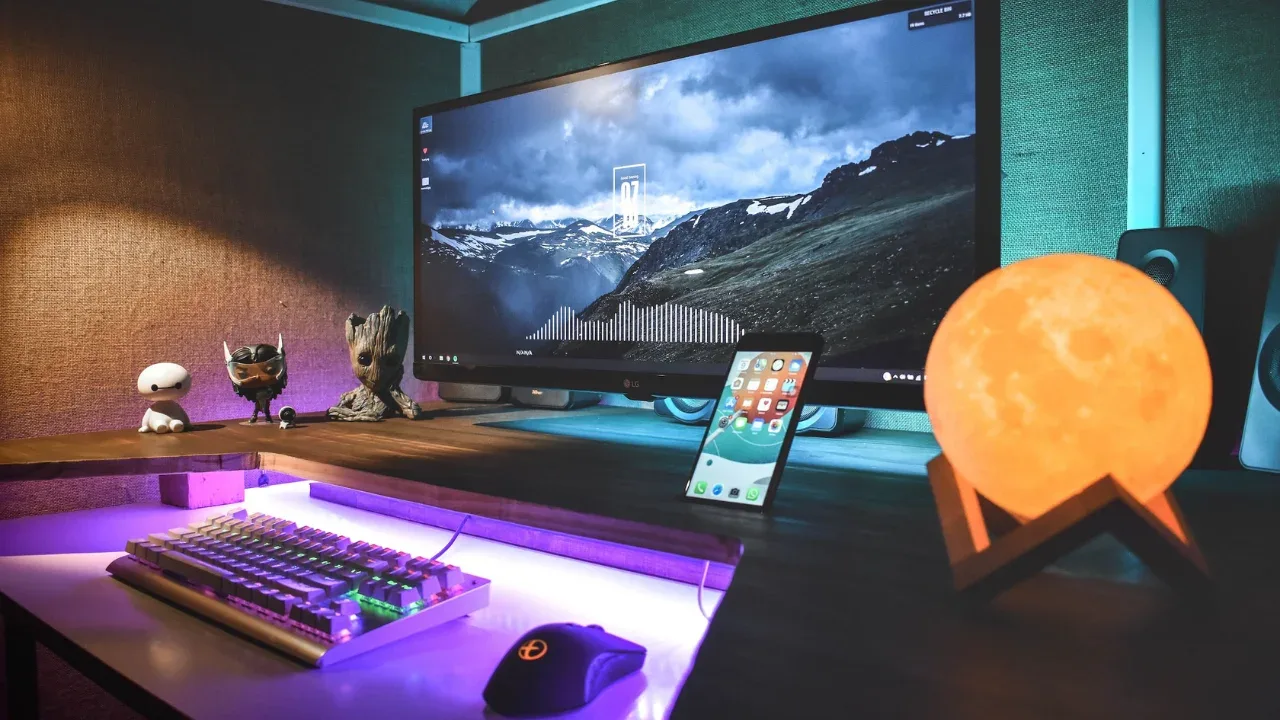
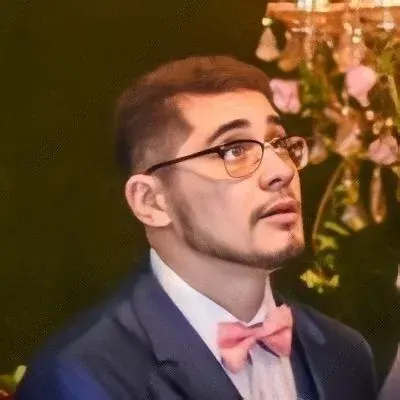
📝 Blog Post: Importing Data from a CSV file to Ruby on Rails
Are you looking for a way to effortlessly import data from a CSV file into your existing Ruby on Rails database table? Look no further! In this guide, we'll walk you through common issues, provide easy solutions, and empower you to handle this task like a pro. Let's dive in! 💪🚀
Understanding the Problem You want to take the data from a CSV file and populate an existing table in your database without saving the CSV file itself. Cool beans! Here's a simple breakdown of the problem:
Ruby version: 1.9.2
Rails version: 3
Existing table name:
mouldings
Here's a sneak peek at what the mouldings
table structure looks like:
create_table "mouldings", :force => true do |t|
t.string "suppliers_code"
t.datetime "created_at"
t.datetime "updated_at"
t.string "name"
t.integer "supplier_id"
t.decimal "length", :precision => 3, :scale => 2
t.decimal "cost", :precision => 4, :scale => 2
t.integer "width"
t.integer "depth"
end
Finding the Solution
Thankfully, Ruby on Rails provides an efficient way to handle CSV imports with the help of the csv
and activerecord-import
gems. Here's how you can accomplish this task:
Install the required gems by adding them to your
Gemfile
:
gem 'csv'
gem 'activerecord-import'
Run
bundle install
to install the newly added gems.In your Rails application, create a new file, such as
csv_importer.rb
, within thelib
directory.Open
csv_importer.rb
and add the following code:
require 'csv'
class CSVImporter
def self.import_data(file_path)
CSV.foreach(file_path, headers: true) do |row|
Moulding.create!(row.to_hash)
end
end
end
Now, whenever you want to import data from a CSV file, simply call the
import_data
method from theCSVImporter
class and pass the file path as an argument. For example:
CSVImporter.import_data('/path/to/your/file.csv')
🎉 That's it! Your Ruby on Rails application will now seamlessly import data from the CSV file and populate the mouldings
table with the respective records. How cool is that? 🙌
📣 Call-to-Action We hope this guide has helped you tackle the challenge of importing data from a CSV file effortlessly. Now that you have the skills, go forth and conquer! If you found this guide helpful, don't forget to share it with your fellow developers and leave a comment below. We'd love to hear about your experiences and any other topics you'd like us to cover. Happy coding! 😊👩💻👨💻
P.S. Remember to always sanitize and validate the CSV data before importing it into your production database to ensure the integrity of your application's data. Safety first! 👮♂️🔒
By the way, if you're interested, you can find this article on our blog at www.example.com/blog/post-importing-csv-ruby-on-rails. Make sure to follow us for more tech tips, tricks, and tutorials! 🌟