Ruby on Rails form_for select field with class
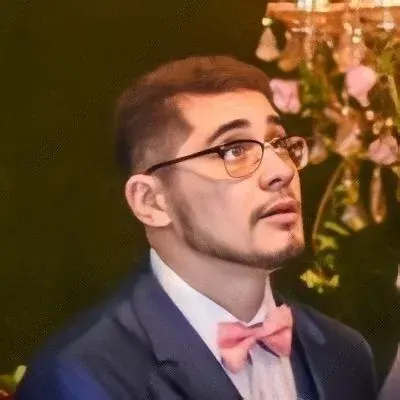
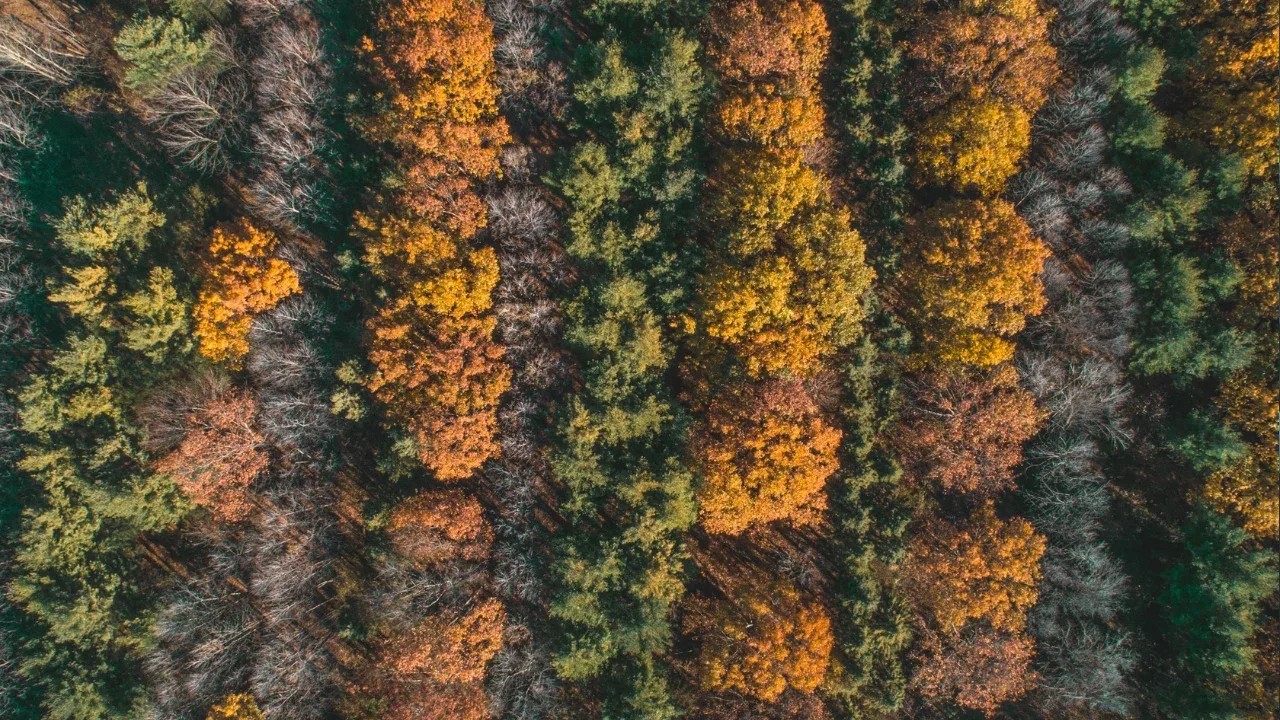
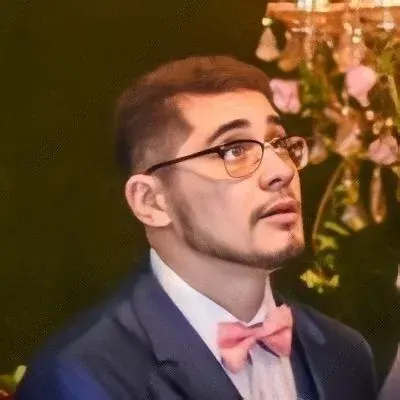
🌟 How to Add a Class to a select field with Ruby on Rails form_for 🌟
Have you ever found yourself struggling to add a class to a select field using the f.select
tag in a Ruby on Rails form? You're not alone! Many developers have faced this issue and have been left scratching their heads 🤔.
The Problem
Let's take a look at the code snippet provided by our fellow coder:
<%= f.select(:object_field, ['Item 1', 'Item 2', 'Item 3', 'Item 4', 'Item 4'], :class => 'my_style_class')%>
This should be a simple select tag, right? But something's missing! Our friend here wants to add a class to the select field using the :class
option, but no matter what they try, it just doesn't seem to work.
The Solution
Fear not, dear developer! I'm here to guide you through this frustrating hurdle. Here are a couple of solutions to try:
Solution 1: Use the html_options
Parameter
One way to add a class to the select field is by using the html_options
parameter. Instead of passing the class directly to the f.select
method, you can pass it as an additional parameter:
<%= f.select(:object_field, ['Item 1', 'Item 2', 'Item 3', 'Item 4', 'Item 4'], {}, {:class => 'my_style_class'})%>
In this solution, we pass an empty hash {}
as the third parameter, with the class options as the fourth parameter enclosed in curly braces. This ensures that the class
attribute gets properly assigned to the select field.
Solution 2: Use the select_tag
Method
If the first solution doesn't work for you, don't worry! We have another trick up our sleeves. You can use the select_tag
method instead of f.select
to have more control over the HTML output:
<%= select_tag(:object_field, options_for_select(['Item 1', 'Item 2', 'Item 3', 'Item 4', 'Item 4']), class: 'my_style_class') %>
In this solution, we use the select_tag
method to create the select field, passing the options using the options_for_select
helper method. We can then directly assign the class with the class
option.
Conclusion
And that's it! You now have two solutions at your disposal to overcome the challenge of adding a class to a select field when using the f.select
tag in Ruby on Rails.
I hope this guide has helped you navigate through this frustrating issue. Feel free to try out these solutions and let us know in the comments which one worked best for you, or if you have any other questions or suggestions.
Now go forth and conquer that form styling! 🚀