Ruby on Rails Callback, what is difference between :before_save and :before_create?
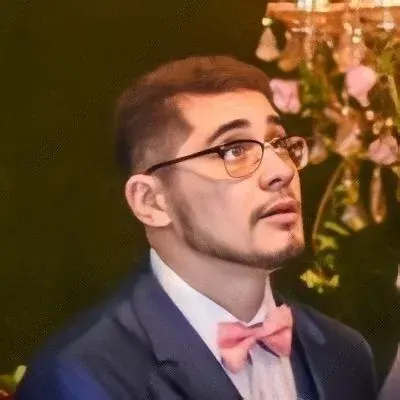
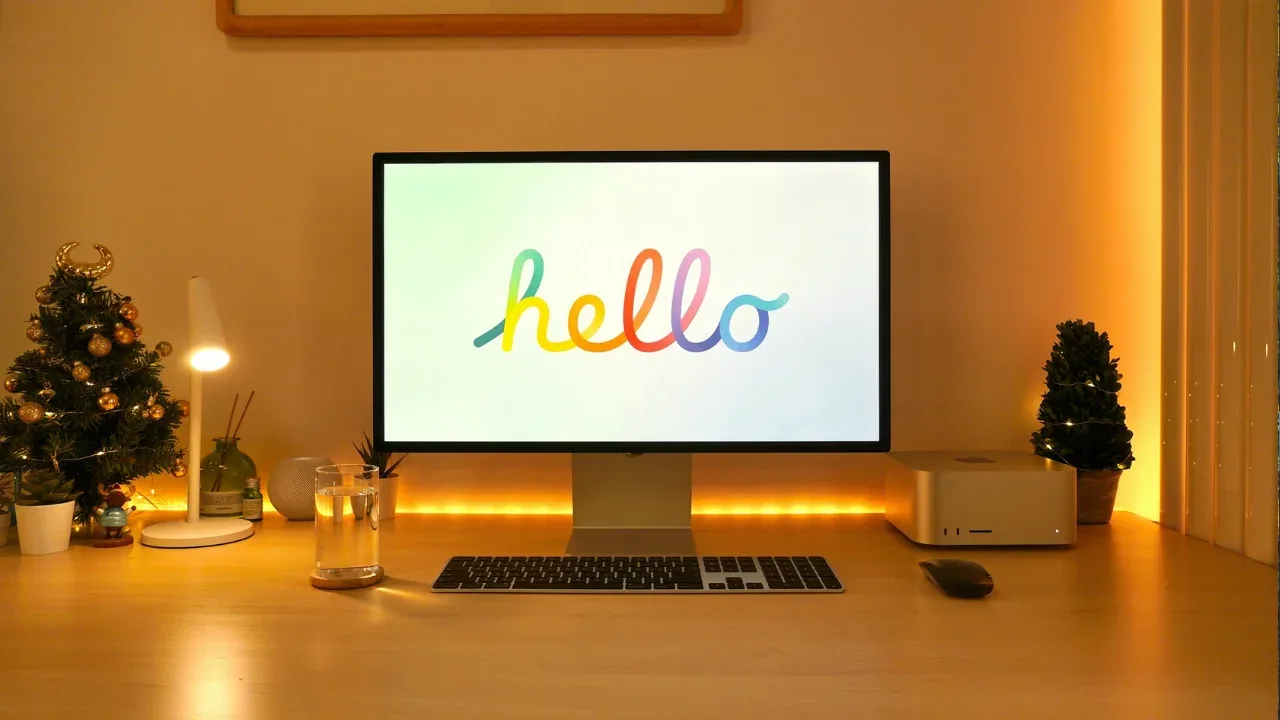
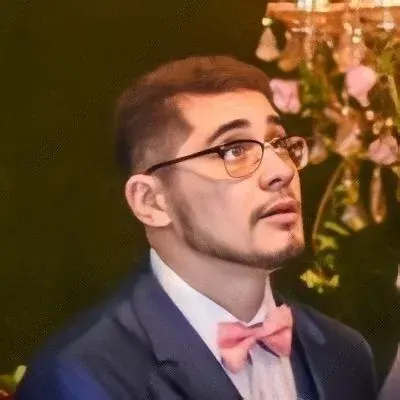
📢 Get Ready to Rock with Ruby on Rails Callbacks! 🚀
If you're a Ruby on Rails developer, you've probably encountered the mighty power of callbacks. 🎵 But what's the difference between the 🤔 :before_save
and :before_create
callbacks? And how do they play with Rails validations? 🌟
🔀 Callbacks in a Nutshell Before we dive into the specific callbacks, let's quickly grasp the concept of callbacks in Ruby on Rails. Callbacks are methods that get triggered at certain points during the lifecycle of an object. They allow you to add extra functionality before or after specific events, such as saving an object to the database.
🎯 :before_save
– The Pre-Saving Dance
The :before_save
callback is triggered just before an object is saved to the database, regardless of whether it's a new record or an existing one. 🔒 This callback provides a perfect opportunity to modify attributes or perform validations before the save operation occurs. 🛠️
🎯 :before_create
– The Prelude to Birth
On the other hand, the :before_create
callback is only triggered before creating a new record. 🧩 This means it will run when you're saving an object for the first time but not when updating an existing record. 🚧
🎓 Validations and Callback Order Now, let's address the question about validations. Do they occur before or after these callbacks? 🤔
🔃 The Save Laundering
When saving an object, validations come into play after both :before_save
and :before_create
callbacks are executed. So, if you have some validations defined, they will validate the object after any changes triggered by these callbacks. 🕺
👀 Here's an example to give you a better sense of the flow:
class Article < ApplicationRecord
before_save :do_something
before_create :do_something_else
validates :title, presence: true
validates :body, length: { minimum: 10 }
private
def do_something
# some fancy code here
end
def do_something_else
# some even fancier code here
end
end
In this example, the :do_something
method will be executed before both saving and creating an article, while :do_something_else
runs only before creating an article. After these callbacks, the validations will take the stage, validating the title
presence and the body
length.
🤩 Reader Engagement Time!
So, what have we learned? We now know the difference between :before_save
and :before_create
callbacks and how they relate to Rails validations. It's time to apply this knowledge to your own Rails projects and unleash the full potential of callbacks! 💪
🎉 Share your thoughts and experiences in the comments below. Have you run into any tricky situations with callbacks? Let's discuss and help each other out! 👯♀️
Happy coding! 💻✨