Remove duplicate elements from array in Ruby
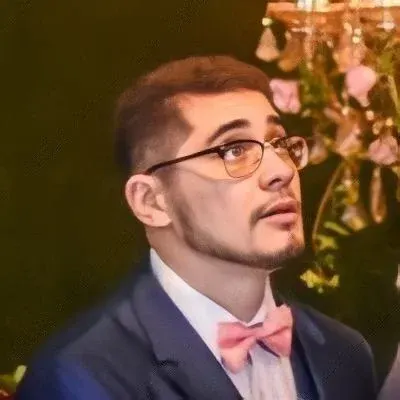
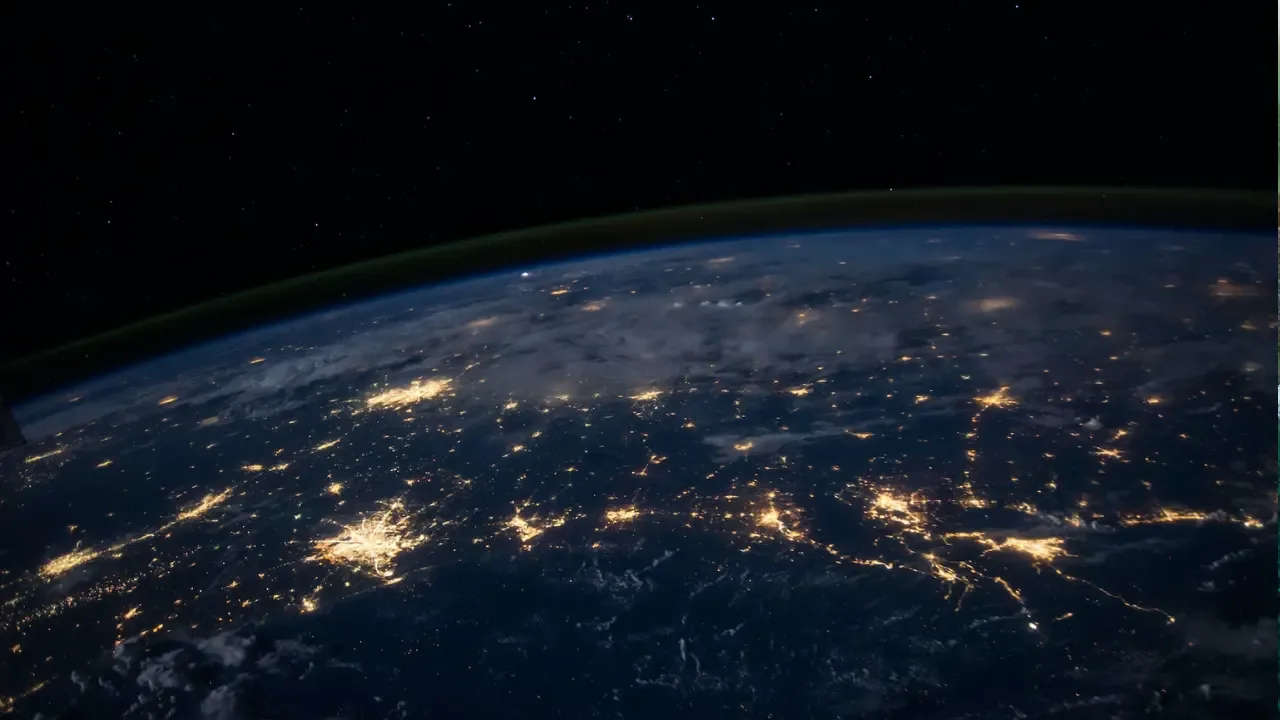
Removing Duplicate Elements from an Array in Ruby
Are you facing the challenge of removing duplicate elements from a Ruby array? Look no further! In this guide, we'll explore a simple and efficient solution that doesn't require for-loops or iteration. Let's dive right in!
The Problem
Consider the following Ruby array containing duplicate elements:
array = [1, 2, 2, 1, 4, 4, 5, 6, 7, 8, 5, 6]
The goal is to remove all the duplicate elements while retaining only the unique ones.
The Solution
Luckily, Ruby provides a nifty method called Array#uniq
that exactly fits our needs. This method returns a new array by removing duplicate elements from the original array. Here's how we can use it:
array = [1, 2, 2, 1, 4, 4, 5, 6, 7, 8, 5, 6]
unique_array = array.uniq
After executing the code above, the unique_array
will now contain all the unique elements present in the original array, effectively removing any duplicates. In our case, the resulting unique_array
will be:
[1, 2, 4, 5, 6, 7, 8]
It's as simple as that! By utilizing the power of Array#uniq
, we can remove duplicates in a single line of code.
A Note on Order
It's important to note that the Array#uniq
method preserves the order of elements in the original array. This means that the resulting array will maintain the same relative order of unique elements as in the original array. So, if order matters to your specific use case, you're in luck!
But Wait, There's More!
If you're dealing with a large array and performance is a concern, you can take advantage of the Array#uniq!
method. This method modifies the array in-place, removing duplicate elements and returning nil
if no changes were made. Here's an example:
array = [1, 2, 2, 1, 4, 4, 5, 6, 7, 8, 5, 6]
array.uniq!
After executing the code above, the array
will be modified to contain only the unique elements. The resulting array
will be:
[1, 2, 4, 5, 6, 7, 8]
By using Array#uniq!
, you can optimize your code and avoid unnecessary memory allocation for a new array.
🎉 Engage with the Community!
I hope this guide helped you remove duplicate elements from your Ruby array effortlessly. If you have any other questions or faced a different array-related challenge, feel free to leave a comment below. Let's help each other and learn together!
🔗 Do you want to explore more Ruby tips and tricks? Visit our Tech Blog for more exciting content.
Now, it's your turn! Have you ever encountered any interesting array manipulation challenges? Share your experiences and insights in the comments section. Let's keep the conversation going!
Happy coding! 💻🚀
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
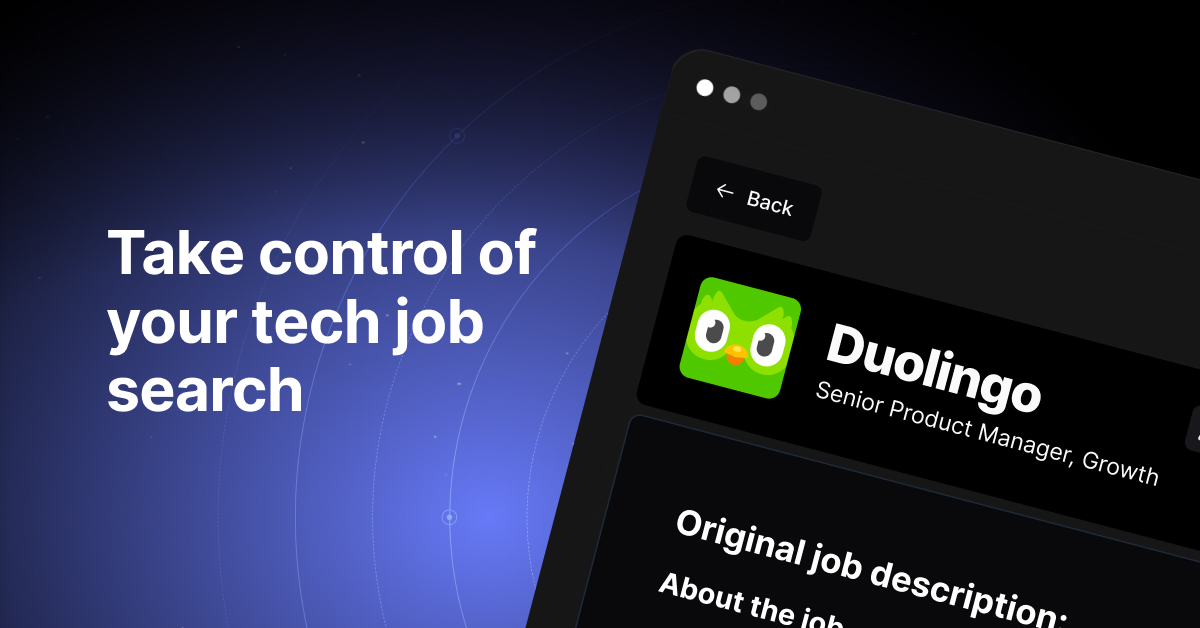