Rails: validate uniqueness of two columns (together)
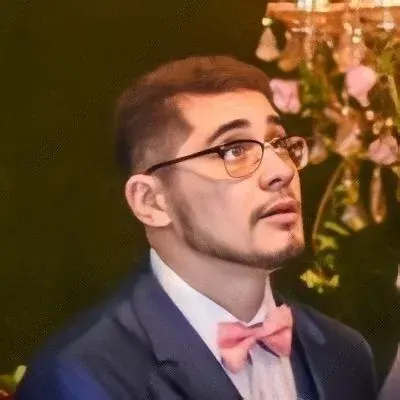
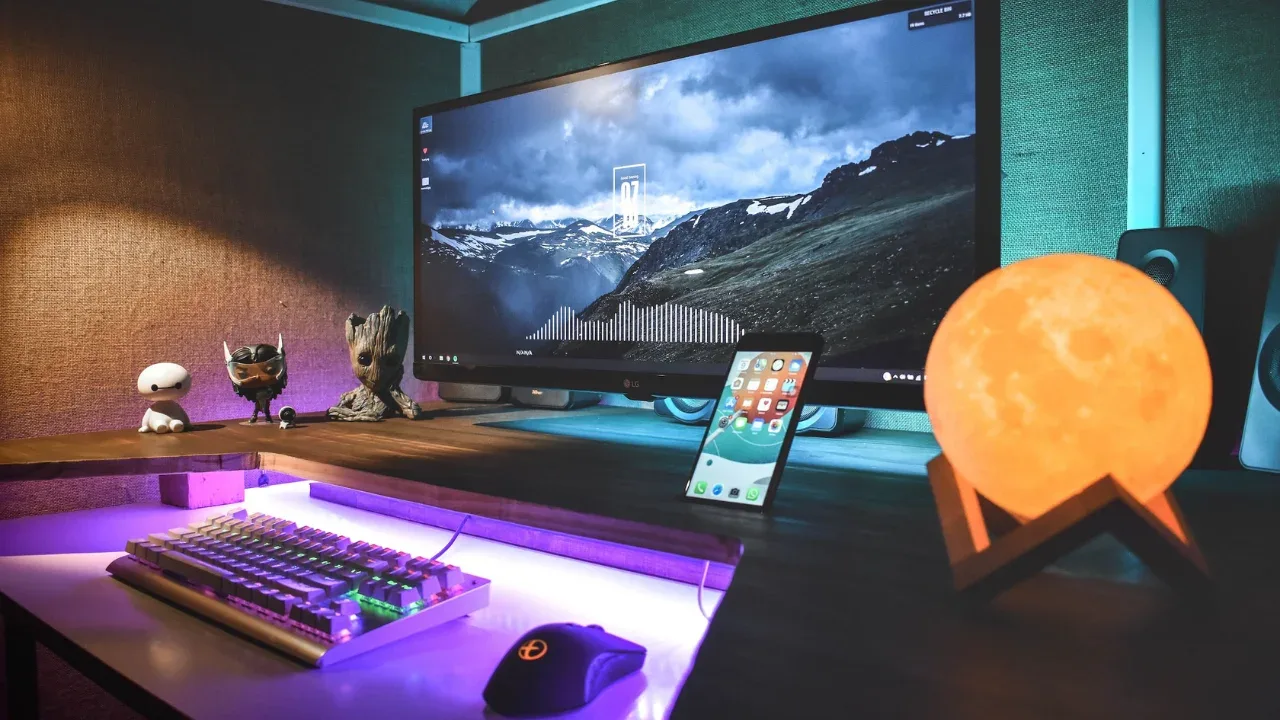
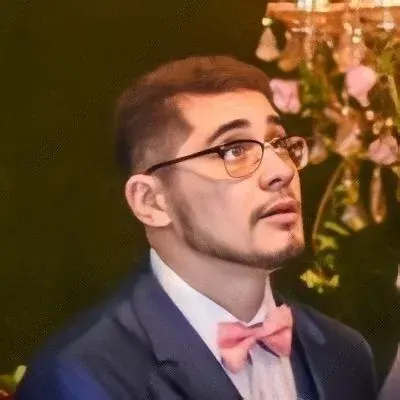
š¢šļø Uniqueness Validation for Multiple Columns in Rails: Master the Art! šāØ
š Hey there, fellow Rails enthusiasts! Today, we're going to address a common issue that often pops up when working with the Rails framework: validating the uniqueness of two columns together. Specifically, we'll tackle the scenario where we want to ensure that there are no duplicate combinations of values in two particular columns.
š” To give you some context, imagine you have a Release
model with various attributes, including medium
and country
. In this case, you want to prevent any releases
from sharing the exact same medium
and country
values. Let's dive into finding the perfect solution for this challenge!
š¤ Identifying the Problem
The main issue here is figuring out how to write a Rails validation that enforces the uniqueness of two columns together. Rails provides several built-in validation methods like uniqueness
, but by default, they only handle one column at a time. So, how can we approach this problem? Fear not, dear reader, for we have a couple of elegant solutions at hand! š
š” Solution 1: Using a Custom Validation Method
One way to tackle this is by using a custom validation method in your Release
model. Let's take a look at an example implementation:
class Release < ApplicationRecord
validate :check_uniqueness_of_medium_and_country
private
def check_uniqueness_of_medium_and_country
if self.class.exists?(medium: medium, country: country)
errors.add(:base, "This combination of medium and country already exists!")
end
end
end
In this solution, we define a custom validation method called check_uniqueness_of_medium_and_country
. Inside the method, we use ActiveRecord's exists?
method to check if any releases
with the same medium
and country
already exist. If a duplicate combination is found, we add an error message to the errors
object.
š Solution 2: Combining Columns with Unique Index
Another approach involves using the power of unique indexes in your database. By creating a unique index on the combination of the medium
and country
columns, we can rely on the database itself to enforce uniqueness.
Here's how we can achieve this:
class AddUniqueIndexToReleases < ActiveRecord::Migration[6.1]
def change
add_index :releases, [:medium, :country], unique: true
end
end
By running this migration, you create a unique index on the medium
and country
columns of the releases
table. This ensures that the combination of these two columns remains unique, addressing the problem directly at the database level.
š£ Call-to-Action: Share Your Thoughts!
There you have it, folks! Two effective solutions to validate the uniqueness of two columns together in Rails. Whether you prefer the flexibility of a custom validation method or the power of unique indexes, you now possess the tools to conquer this challenge. šŖāØ
š Now, it's your turn! Which approach resonates with you the most? Have you encountered any other unique validation scenarios in Rails? Share your thoughts and experiences in the comments section below. Let's learn and grow together as a vibrant Rails community! š¤š
š¢ Keep Exploring, Keep Coding! šš„