Rails params explained?
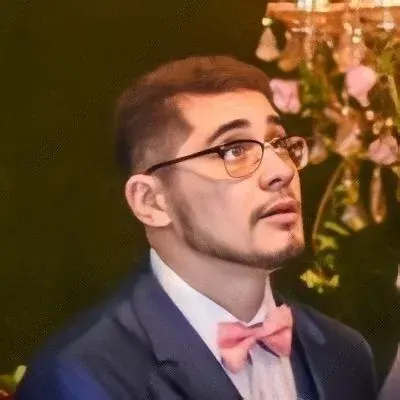
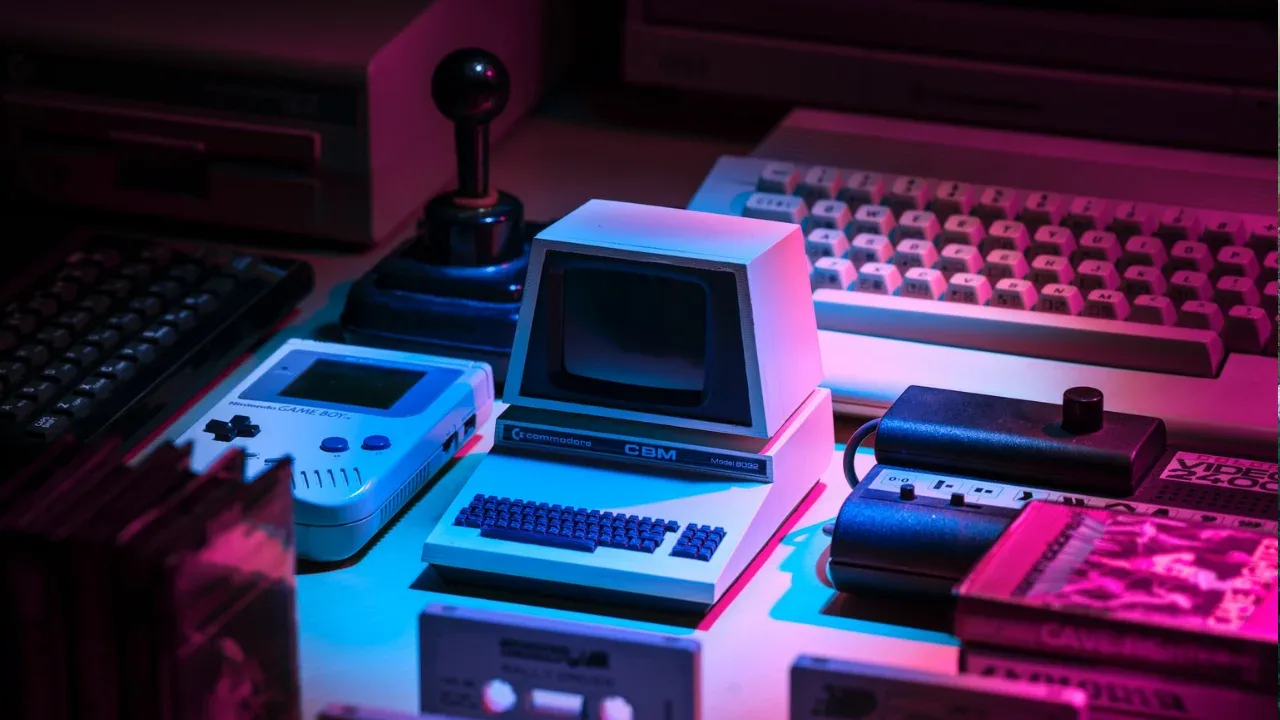
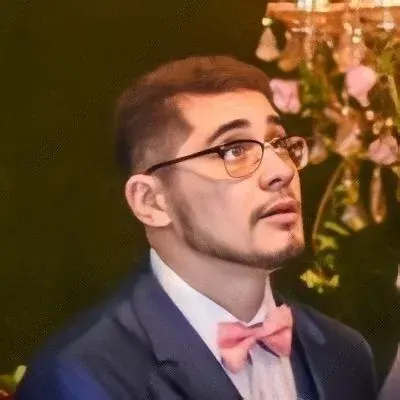
A Beginner's Guide to Understanding Rails params
š
Rails developers often come across the term params
in their controller code. It's essential to understand what params
are and how to use them effectively to handle user input. In this guide, we will explain the purpose of params
, where they come from, and how to reference them. By the end of this post, you'll be able to read and understand code that involves params
with ease.
What are params
in Rails?
params
is a shorthand for "parameters" and refers to the data sent by a client to a server. In the context of Rails, params
represent the user input captured through forms or sent as query parameters in the URL.
In the example code provided, params[:vote]
represents a hash containing the user's voting data. It includes the item_id
and user_id
, which are accessed as params[:vote][:item_id]
and params[:vote][:user_id]
respectively.
Where do params
come from?
When a user interacts with a Rails application by submitting a form, the form data is automatically captured and sent to the server as part of an HTTP request. Rails collects this data and stores it in the params
hash, making it accessible within controller actions.
For instance, in the create
action of the provided code snippet, the params[:vote]
hash contains the voting data submitted by the user.
Understanding the provided code
Now, let's break down the code snippet and understand what it does:
def create
@vote = Vote.new(params[:vote])
item = params[:vote][:item_id]
uid = params[:vote][:user_id]
@extant = Vote.find(:last, conditions: ["item_id = ? AND user_id = ?", item, uid])
last_vote_time = @extant.created_at unless @extant.blank?
curr_time = Time.now
end
The first line instantiates a new
Vote
object usingparams[:vote]
, which contains the voting data submitted by the user.The
item
variable is assigned the value ofparams[:vote][:item_id]
, representing the ID of the item being voted on.Similarly, the
uid
variable is assigned the value ofparams[:vote][:user_id]
, representing the ID of the user casting the vote.The next line fetches the last vote (
Vote
) recorded for the given item and user usingVote.find
with appropriate conditions.The
last_vote_time
variable is assigned the value of@extant.created_at
(timestamp of the last vote), provided@extant
is not blank.The
curr_time
variable is assigned the current timestamp usingTime.now
.
Easy Solutions for Common params
Issues
Using params
in Rails can sometimes lead to issues or unexpected behavior. Here are a few common problems you might encounter and their solutions:
Missing Parameters: When accessing nested parameters like
params[:vote][:item_id]
, you might encounter errors if any of the keys are missing. To handle this, utilize Rails'dig
method, which returnsnil
instead of raising an error:item = params.dig(:vote, :item_id) uid = params.dig(:vote, :user_id)
Strong Parameters: In recent versions of Rails, it's necessary to use strong parameters to permit only the desired attributes from
params
for security reasons. To do this, define a private method in your controller:private def vote_params params.require(:vote).permit(:item_id, :user_id) end
Then, use
vote_params
instead ofparams[:vote]
when creating or updating objects.
Engage with us and share your experience!
We hope this guide helped you understand how params
work in Rails and how to handle common issues that may arise. š
Have you encountered any challenges with params
in your Rails projects? Let us know in the comments below! Feel free to share your own tips and tricks too. Let's help each other out! š
Also, make sure to subscribe to our newsletter to receive more helpful Rails tips and tricks straight to your inbox. Together, let's #CodeRailsLikeAPro! š»š
Your turn! Do you have any questions about params
or any other topic you'd like us to cover in a future blog post? Leave a comment or reach out to us on Twitter at @OurAwesomeTechBlog. We love hearing from our readers and will do our best to address your queries. š¢š¬
Happy coding! šāØ