Rails new vs create
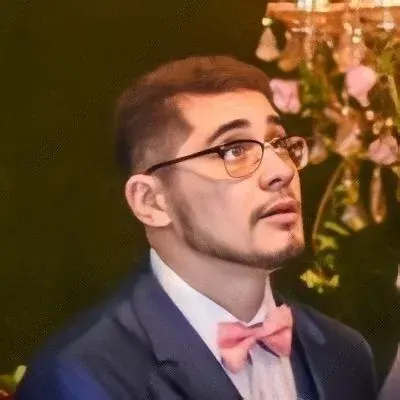
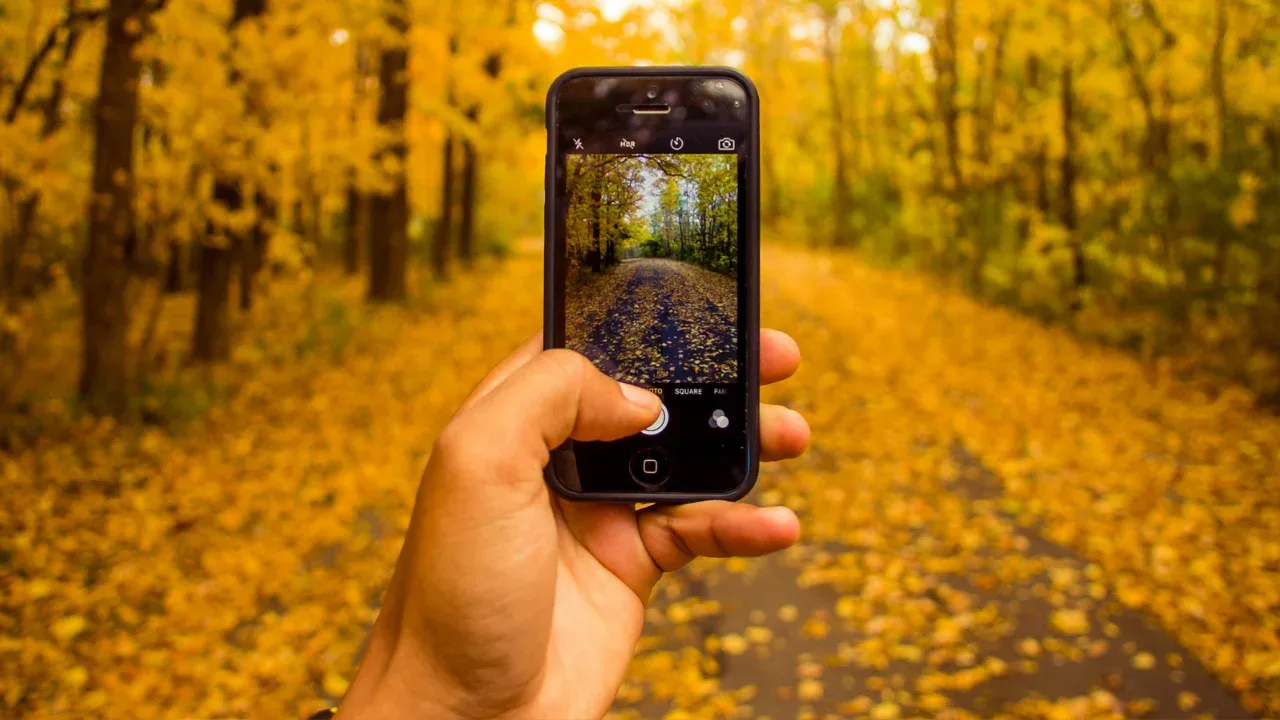
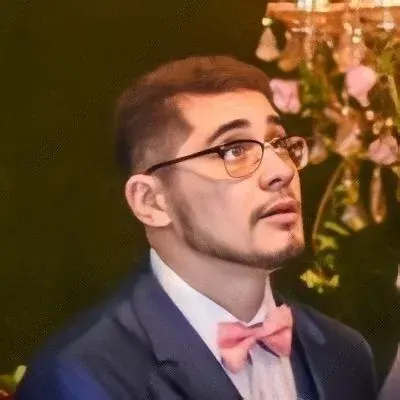
Understanding the Difference: Rails new vs create 🚀💡
Introduction
Are you new to Rails development and confused about the difference between the new
and create
methods in RESTful controllers? You're not alone! This is a common question that many developers encounter. 😕 But fear not, in this blog post, we will demystify this topic and provide you with easy solutions to understand and utilize these methods effectively. Let's dive in! 💪
The Question at Hand 🤔
"Why is there a need to define a new method in RESTful controller, followed by a create method?" This was the perplexing query that our reader, who had conducted a Google search without success, asked us. While understanding the difference between new
and create
is one thing, it's crucial to comprehend why they are used in the way they are. 🤷♀️
What's the Difference? 🔄
In Rails, the new
method is used to instantiate a new instance of an object, while the create
method is used to save this new instance to the database. Let's break it down further with an example: 🏗️📚
# app/controllers/books_controller.rb
class BooksController < ApplicationController
def new
@book = Book.new # Instantiates a new Book object
end
def create
@book = Book.new(book_params) # Instantiates a new Book object with the provided parameters
if @book.save
redirect_to books_path
else
render 'new'
end
end
private
def book_params
params.require(:book).permit(:title, :author, :genre)
end
end
In the code snippet above, the new
method is responsible for instantiating a new instance of the Book
model, while the create
method takes the parameters from the form and creates a new record in the database when the save
method is successful. 📝💾
Why the Two-Step Process? 🤷♂️
You might wonder why we go through this two-step process of first creating a new instance and then saving it to the database. The answer lies in separation of concerns and adhering to the principles of RESTful architecture. 📏🏗️
When a user visits the "new book" page, they need a form to enter the book details. By using the new
method, we provide a blank form where users can input the required information. This form is then submitted to the create
method, which handles the record creation logic. This clear separation ensures that our code is clean and adheres to the Single Responsibility Principle. 🌟🔒
Easy Solutions for Easier Understanding 🎯
Now that we've clarified the difference and the rationale behind it, here are some easy solutions to help you better understand and utilize new
and create
effectively in your Rails applications: 🚀
Read the Documentation: Rails provides comprehensive documentation that explains the purpose and usage of these methods in detail. Take the time to read through them, as they can provide valuable insights. 📖
Follow Tutorials: There are numerous online tutorials that walk you through building Rails applications. These tutorials often cover the usage of
new
andcreate
within the context of a practical example, helping you understand their purpose more effectively. 🌐Ask the Community: Don't be afraid to ask questions! Join online developer communities, such as Stack Overflow or Rails forums, where experienced developers are always eager to help. Learning from the community can provide you with real-life examples and additional tips and tricks. 💬
Conclusion and Call to Action 📢👏
Congratulations! 🎉 You have successfully grasped the difference between the new
and create
methods in Rails. Remember, the new
method is used for instantiating new instances, while the create
method saves these instances to the database. By understanding the underlying principles and following our easy solutions, you'll be able to enhance your Rails development skills and build even more robust applications. 💪💻
If you found this blog post helpful, share it with your fellow developers and spread the knowledge! 💙 And don't hesitate to leave a comment below if you have any questions or want to share your experience with new
and create
methods. Let's keep the conversation going! 🚀🗣️