Rails migrations: Undo default setting for a column
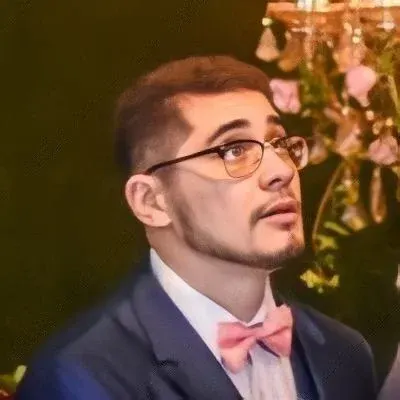
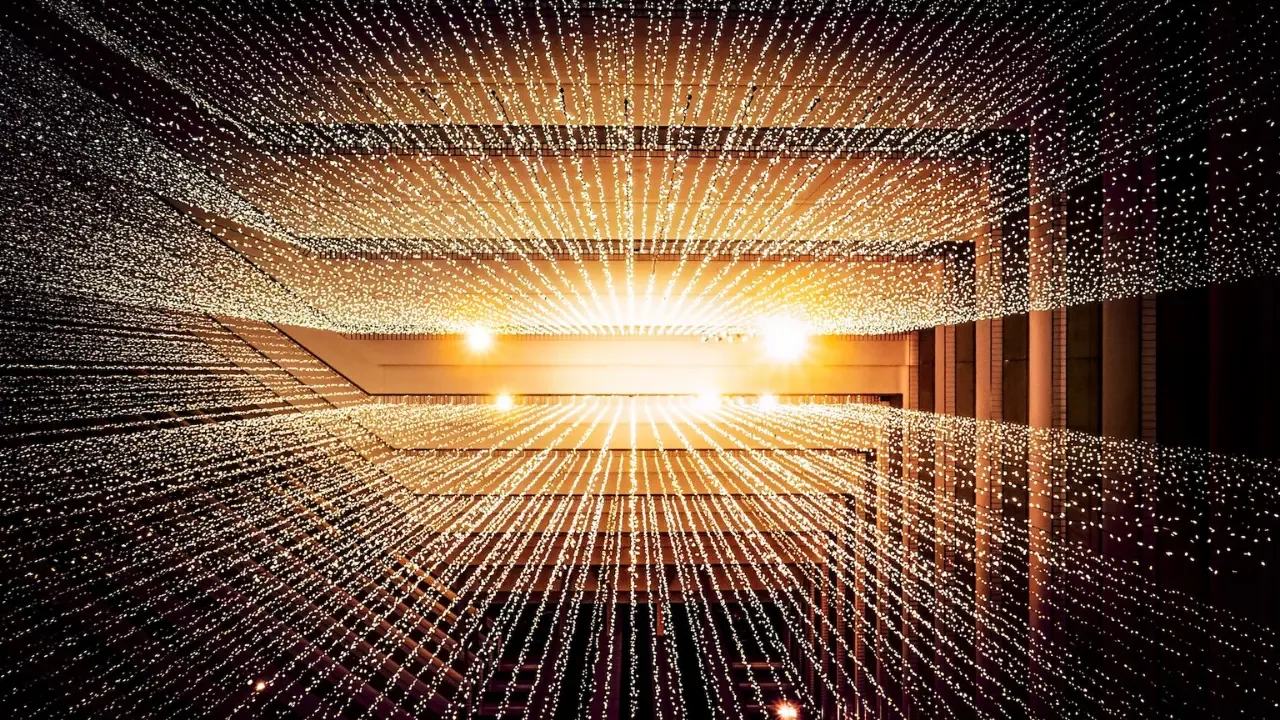
Undo Default Setting for a Column in Rails Migrations
So, you're working on a Rails project and you find yourself in a situation where you need to undo the default setting for a column in one of your migrations. Don't worry, I've got you covered! In this blog post, I'll explain common issues and provide easy solutions to help you overcome this challenge.
The Problem
Let's start by understanding the problem at hand. Suppose you have a migration that sets up a default value for a column in your database table, like this:
def self.up
add_column :column_name, :bought_at, :datetime, :default => Time.now
end
Now, imagine you want to remove or drop that default setting in a later migration. You might be tempted to execute a custom SQL command in your Rails migration, like this:
def self.up
execute 'alter table column_name alter bought_at drop default'
end
While this workaround might do the job, it's not an ideal solution. You become dependent on how the underlying database interprets this command. If there's a change in the database, this query may not work anymore, and your migration could break. But fear not, my fellow developer! There's a better way to handle this situation.
The Solution
Rails migrations provide a cleaner and more reliable solution for undoing default settings on columns. Instead of relying on raw SQL commands, you can use Rails' own migration methods to revert the default setting.
To undo the default setting for your column, you can modify your migration code like this:
def self.up
change_column_default :column_name, :bought_at, nil
end
By passing nil
as the new default value, you effectively remove the default setting from the column. This method is database agnostic and ensures that your migration will work regardless of the underlying database engine.
A Friendly Reminder
Remember to consider the impact of this change. If your application relies on the default value for this column, removing it might lead to unexpected behavior. Make sure to assess the implications and update your code accordingly.
Conclusion
Undoing default settings for a column in Rails migrations can be a common challenge. But with the change_column_default
method, you now have an easy and database-agnostic solution at your disposal.
No more worrying about compatibility issues or broken migrations due to raw SQL commands. Embrace the Rails way and leverage the power of built-in methods to keep your migrations clean and reliable.
If you found this guide helpful, let me know by leaving a comment below. I'd love to hear your thoughts and any other topics you'd like me to cover in future blog posts. Happy coding! 💻🚀
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
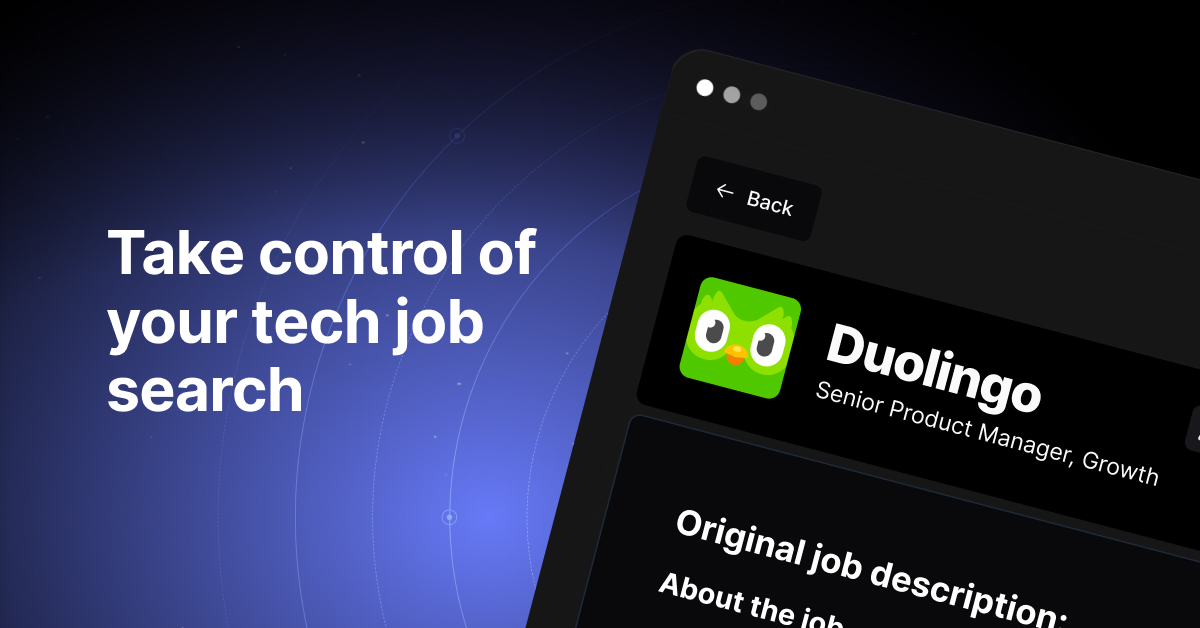