Rails - How to use a Helper Inside a Controller
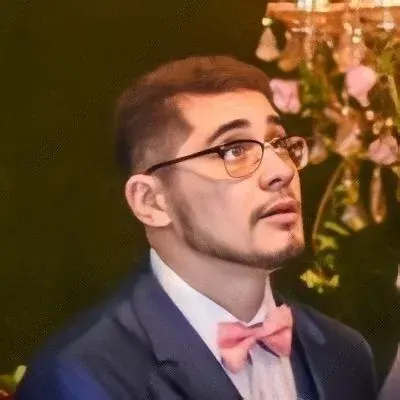
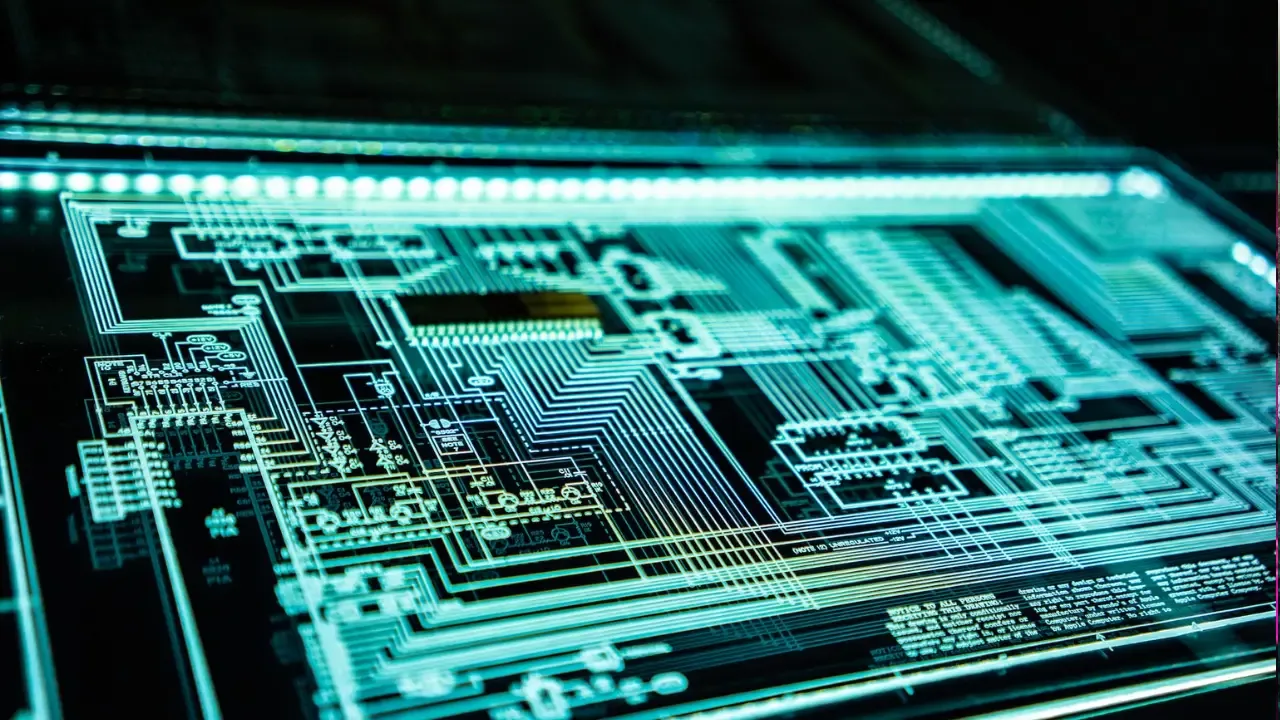
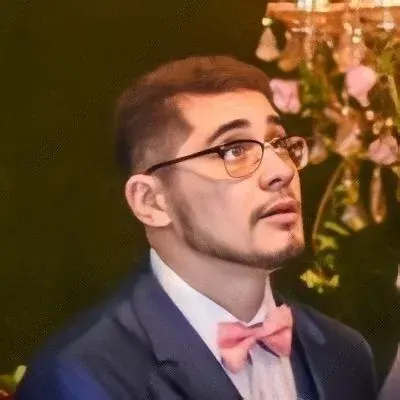
🚀 Rails - How to Use a Helper Inside a Controller
Do you find yourself in a situation where you need to use a helper inside a controller, like when building a JSON object to return? Look no further! In this guide, we'll explore different approaches to accessing a helper inside a Rails controller. 🤩
Understanding the Challenge
In Rails, helpers are primarily designed to be used within views and not intended to be directly accessed from controllers. However, there might be scenarios where using a helper within a controller becomes necessary, like when you need to format data for a JSON response. 🤔
In the example provided, the challenge is to access the html_format
helper inside the xxxxx
action of the controller to format the content before it's included in the JSON response.
Solution 1: Include the Helper
One of the simplest ways to use a helper inside a controller is by explicitly including the helper module. This approach allows you to access the helper methods within the controller actions. 😎
To include a helper module in a controller, follow these steps:
Open the controller file where you want to access the helper.
Add the following line at the top of the controller class:
include YourHelperModuleName
Replace YourHelperModuleName
with the actual module name of your helper.
In the given example, assuming the helper module is called HtmlFormattingHelper
, we can modify the controller code as follows:
class YourController < ApplicationController
include HtmlFormattingHelper
def xxxxx
@comments = Array.new
@c_comments.each do |comment|
@comments << {
:id => comment.id,
:content => html_format(comment.content)
}
end
render :json => @comments
end
end
💡 Note: Including a helper module gives access to all the helper methods, so make sure to consider any potential implications before using this approach.
Solution 2: Utilize a Concern
Another way to access a helper inside a controller is by utilizing Rails concerns. Concerns are reusable modules that can be shared across different classes, providing a clean and structured way to share common functionality. This approach helps to keep your code modular and promotes maintainability. 🧩
To use a concern to access a helper, follow these steps:
Create a new concern module. For example, let's call it
HelperAccess
.
module HelperAccess
extend ActiveSupport::Concern
included do
include YourHelperModuleName
end
end
Import the concern module inside your controller:
class YourController < ApplicationController
include HelperAccess
# Your other controller code...
end
With this approach, the helper methods are accessible through the included module, making them available within the controller actions.
Conclusion & Takeaways
While it is generally recommended to keep helper methods within views and not directly access them from controllers, there are situations where accessing a helper from a controller becomes necessary. In those cases, we explored two solutions: including the helper directly or utilizing a concern.
Keep in mind that using a helper inside a controller may not always be the best approach, and it's important to weigh the pros and cons before implementing it. Remember, Rails conventions are there for a reason! 🙌
Do you have any other questions or challenges in your Rails journey? Share them with us in the comments below or reach out to us on social media. Happy coding! 🔥
{CTA:} Don't forget to 👍 like, 💬 comment, and 📢 share this article to help other Rails developers who may face similar challenges!