Rails: How do I create a default value for attributes in Rails activerecord"s model?
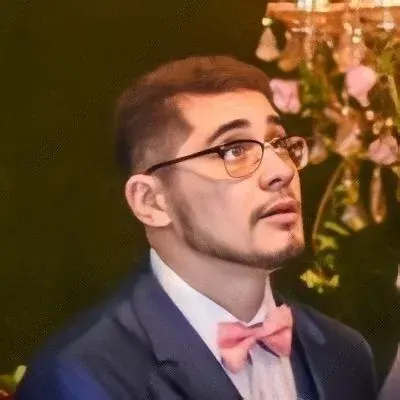
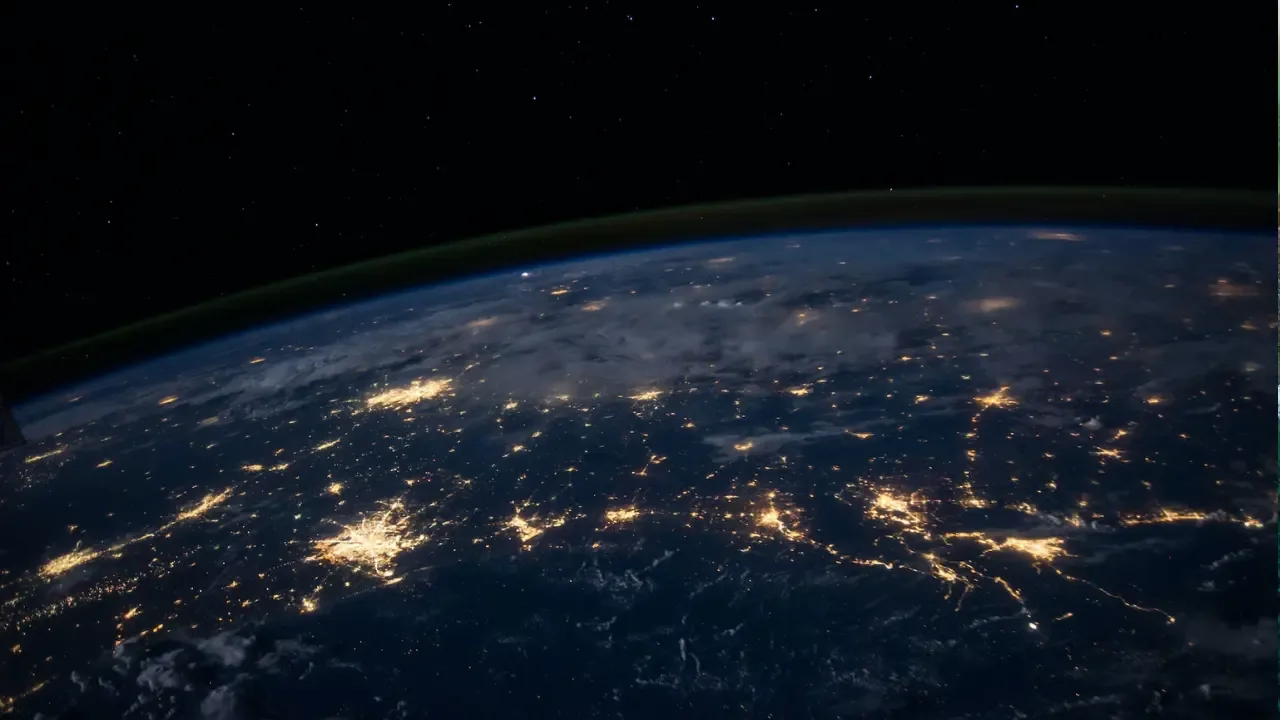
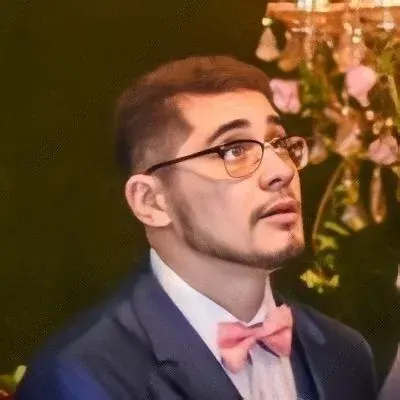
Creating a Default Value for Attributes in Rails ActiveRecord's Model
š Hey there, fellow Rails enthusiasts! Have you ever wondered how to set a default value for an attribute in Rails ActiveRecord's model? Look no further because we've got you covered! In this guide, we'll address the common issue faced by developers and provide easy solutions to create a default value for attributes. Let's dive right in! šŖ
The Common Problem
The question at hand is to set a default value for an attribute called :status
in an ActiveRecord model, such as Task
. The initial attempt by our fellow developer was to define a custom writer method in the model:
class Task < ActiveRecord::Base
def status=(status)
status = 'P'
write_attribute(:status, status)
end
end
However, upon creating a new record, an error was encountered:
ActiveRecord::StatementInvalid: Mysql::Error: Column 'status' cannot be null
š¤ The error suggests that the value was not applied to the :status
attribute. So, what's the elegant way to tackle this scenario? Let's explore some solutions! š
Solution 1: Using default
Option
The simplest way to set a default value is by utilizing the default
option provided by ActiveRecord. You can specify the default value directly in the migration file when creating the corresponding column. Here's an example:
class CreateTasks < ActiveRecord::Migration[6.1]
def change
create_table :tasks do |t|
t.string :status, default: 'P'
# Other attribute columns...
t.timestamps
end
end
end
š„ Voila! The :status
attribute now has a default value of 'P'
whenever a new record is created. No need for any custom writer methods. Easy peasy, right?
Solution 2: Using after_initialize
Callback
Another approach to set a default value is by leveraging the after_initialize
callback in your ActiveRecord model. This callback method gets triggered every time an object is instantiated. Here's how you can use it:
class Task < ActiveRecord::Base
after_initialize :set_default_status
private
def set_default_status
self.status ||= 'P'
end
end
In the above example, we're using the ||=
operator to set the default value only if the status
attribute is nil
or false
. This way, we ensure that the default value is assigned when a new record is created. š
Time to Create Your Default Value!
š We've explored two elegant solutions for creating default values for attributes in Rails ActiveRecord models. Whether you prefer using the default
option in your migration file or the after_initialize
callback in your model, both approaches will help you achieve the desired outcome.
Now it's time for you to implement these techniques in your own Rails projects. Give them a try and see which solution works best for you! Remember, creating default values for attributes can save you time and effort in the long run.
š Do you have any other tricks up your sleeve for handling default values in Rails ActiveRecord models? Let's continue the conversation in the comments section below! Share your thoughts, challenges, or even your own creative solutions. We'd love to hear from you and learn together.
Happy coding! š»š