Override devise registrations controller
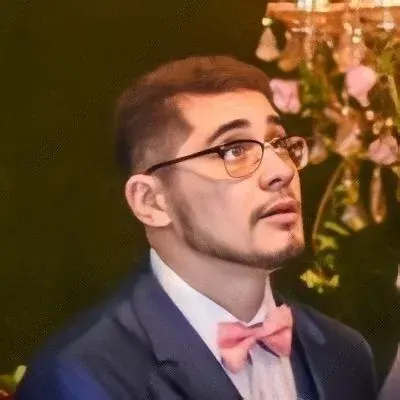
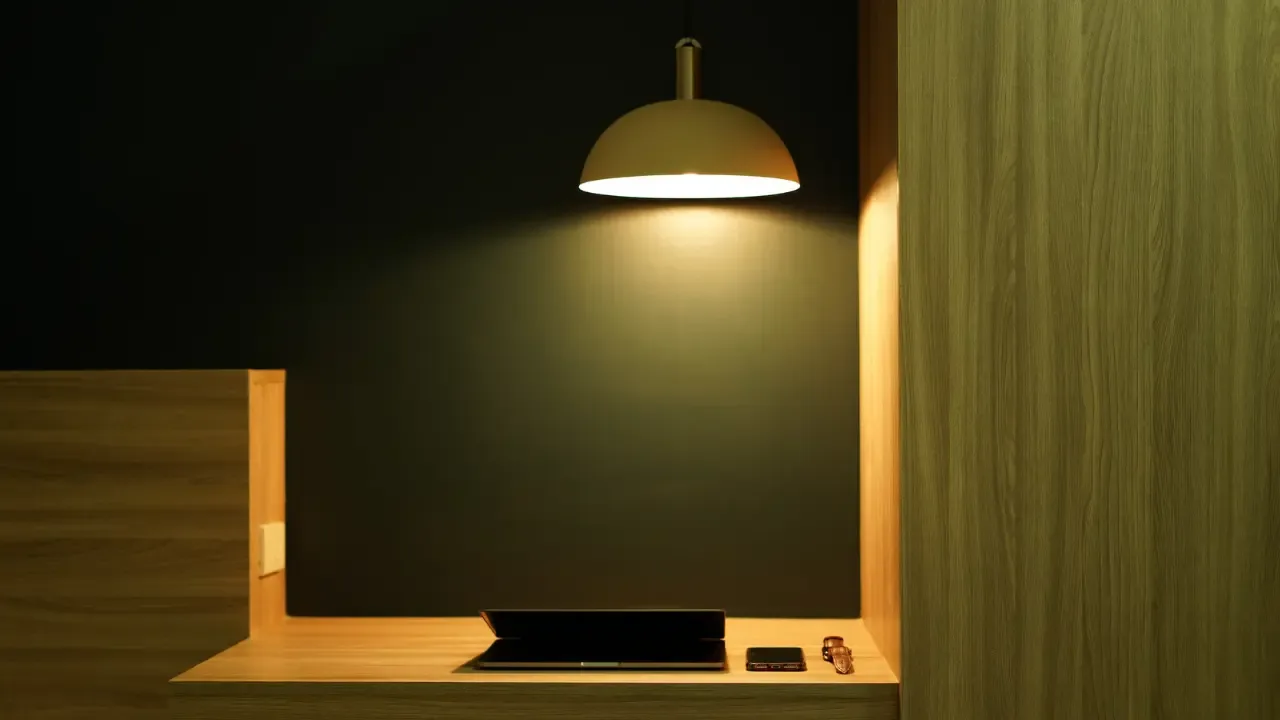
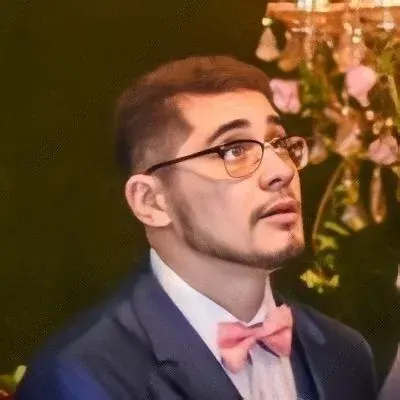
📝 Blog Post: Override Devise Registrations Controller
Hey there tech enthusiasts! Welcome back to another awesome blog post where we tackle tech dilemmas with style and ease. Today, we're diving into the world of Devise and overcoming the common issue of overriding the registrations controller. Let's get right into it!
🔎 Identifying the problem
So, you've added a fancy new field to your sign-up form, but things aren't going as smoothly as you'd hoped. Upon hitting that save button, an ominous Activerecord::UnknownAttributeError
pops up, pointing directly at the newly added field (in this case, "company"). Don't stress, we've got your back!
🔄 Why would you want to override the registrations controller?
Now, you may be wondering why on earth you would need to override the registrations controller. Well, in certain scenarios, like yours, where additional customizations are required, overriding the default controller becomes essential. It allows you to take control and tailor the sign-up process to your exact needs.
🛠️ Easy solution: Overriding the Devise Registrations Controller
To override the registrations controller, follow these steps:
Create a new file in your controllers directory (if it doesn't exist already) named
registrations_controller.rb
. This will be your customized registrations controller.Open the newly created file and define the class
RegistrationsController
which inherits fromDevise::RegistrationsController
. Your code should look something like this:class RegistrationsController < Devise::RegistrationsController # Your custom code goes here end
Now, we'll specifically address the issue with the "company" field causing the
UnknownAttributeError
. Override thesign_up_params
method inside yourRegistrationsController
and permit the "company" attribute. Here's an example:class RegistrationsController < Devise::RegistrationsController private def sign_up_params params.require(:user).permit(:email, :password, :password_confirmation, :company) end end
Almost there! We need to inform Devise to use our customized registrations controller instead of the default one. Add the following line at the bottom of your
routes.rb
file:devise_for :users, controllers: { registrations: 'registrations' }
📢 Time for action!
It's showtime, folks! Fire up your sign-up form, fill in all the required details, including the fantastic "company" field, and hit that glorious save button. 🚀
🔎 Pro-tip: Avoiding attribute errors with nested attributes
Remember the additional field that caused all this trouble in the first place? If it's based on a different model and you're using nested attributes, make sure to check out this helpful resource: How do I use nested attributes with the Devise model. It'll clear up any confusion and prevent further attribute-related headaches.
🙌 Engage with us!
Have you successfully overridden the registrations controller? Share your triumphs, experiences, or any hurdles you faced along the way in the comments section below. We'd love to hear from you and help out if needed.
That's a wrap, folks! We hope this guide has cleared up any confusion and empowered you to conquer the beastly task of overriding the Devise registrations controller. Happy coding! 😊✨