OO Design in Rails: Where to put stuff
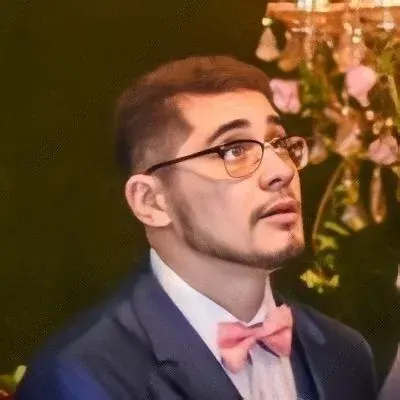
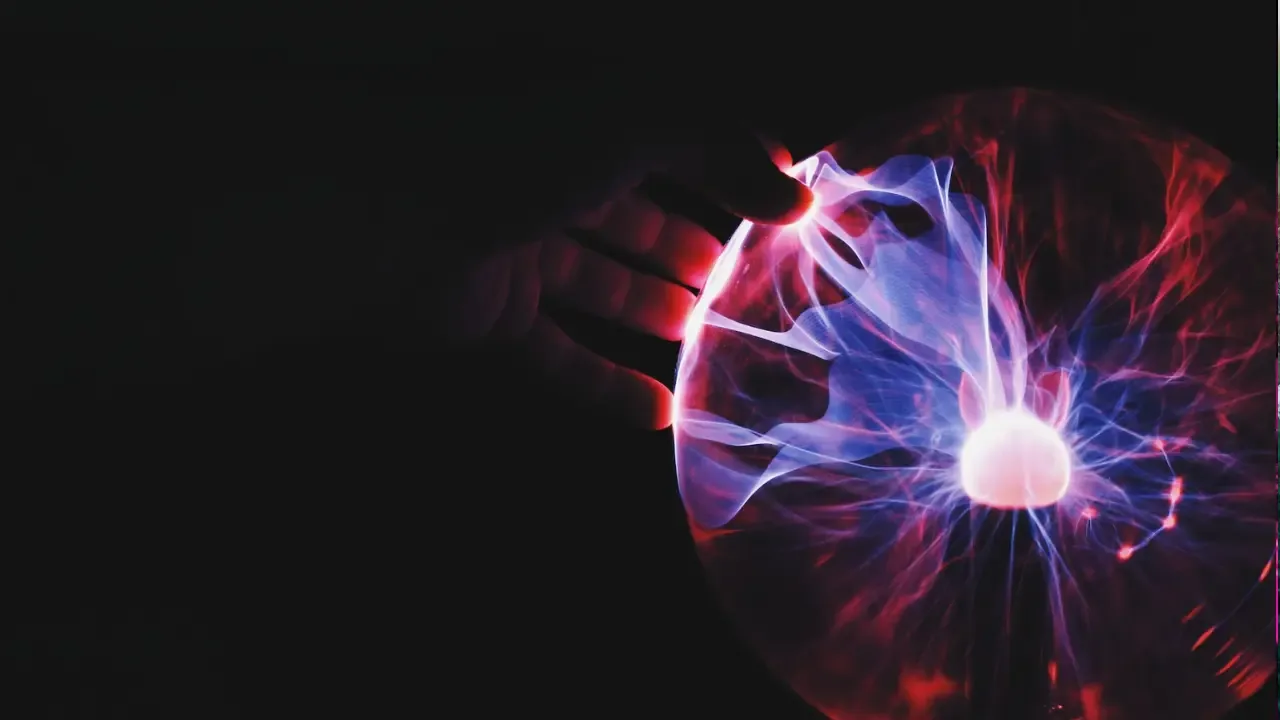
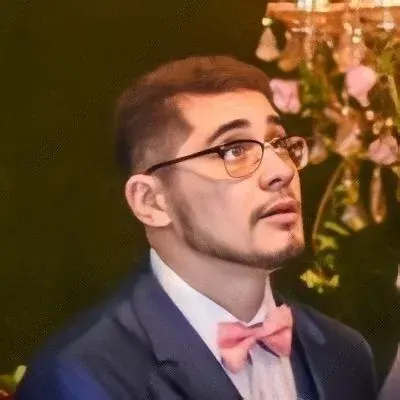
๐ Title: Rails Design Dilemma: Where to Put Stuff?
๐ Hey there, fellow Rails enthusiasts! ๐
I hear you're loving Rails and its object-orientedness, but you're facing a common conundrum: where do you put all the stuff to keep your codebase organized? ๐ค Don't worry, you're not alone! Many developers have grappled with this same question. In this blog post, I'll share some easy solutions to this problem, so you can keep your classes from growing out of control. Let's dive in! ๐โโ๏ธ
๐ 1. Controllers: One Resource, One Controller
One common pitfall is ending up with huge controllers that handle multiple resources. ๐ To avoid this, follow the Rails convention of having one controller per resource. For example, if you have models for users, posts, and comments, create separate controllers for each:
app/controllers/
users_controller.rb
posts_controller.rb
comments_controller.rb
This approach keeps your controllers focused and easy to maintain. ๐งน
๐ 2. Models: Embrace Associations and Concerns
When it comes to your models, ๐ฅ associations and concerns are your best friends. ๐ค Associations allow you to define relationships between different models, ensuring that responsibilities are divided efficiently.
For example, consider a blog application with users and posts:
class User < ApplicationRecord
has_many :posts
end
class Post < ApplicationRecord
belongs_to :user
end
By setting up this association, you can access a user's posts easily: user.posts
. โจ
To further organize your models, leverage concerns. ๐คฒ Concerns allow you to extract common functionality into separate modules, which can be included in multiple models. For instance, if both User and Post models have similar validation logic, you can create a Validatable
concern:
module Validatable
extend ActiveSupport::Concern
included do
validates_presence_of :title
validates_length_of :body, maximum: 500
end
end
class User < ApplicationRecord
include Validatable
end
class Post < ApplicationRecord
include Validatable
end
Voila! ๐ฉ Your code is now DRY and organized.
๐ 3. Views: Leverage Helpers and Partials
When it comes to views, the key is to keep them as lightweight as possible. ๐จ Utilize helper methods and partials to encapsulate reusable view logic.
For example, if your application needs to display a formatted timestamp, you can define a helper method in app/helpers/datetime_helper.rb
:
module DatetimeHelper
def format_timestamp(datetime)
datetime.strftime("%A, %B %e, %Y")
end
end
Now, you can use this helper method in your views:
<%= format_timestamp(post.created_at) %>
By extracting such formatting and rendering logic into helpers and partials, you keep your views clean and focused on presentation. ๐
๐ก Pro Tip: If you find your helpers growing too large, consider organizing them into separate modules within the helpers/
directory. Just make sure to include them in the relevant controller or view.
๐ 4. Lib and Engines: Know Your Choices
Sometimes, the conventional Rails directory structure might not cut it for larger or complex projects. In such cases, you have a few additional options:
lib/
: This directory is meant for code that doesn't fit into the conventional MVC structure. You may create subdirectories withinlib/
to organize your code as needed.Engines: Rails engines allow you to encapsulate reusable functionality into separate modules that can be mounted into your application. You can think of them as miniaturized Rails applications.
To decide between lib/
and engines, consider the scope and level of reusability of your code. If the functionality is tightly coupled with your main application, opt for lib/
. If it can exist as a separate, reusable component, go for an engine. ๐
๐ Conclusion: Time to Organize!
Now that you know where to put stuff in your Rails application, it's time to refactor and organize your code like a pro! ๐ Remember the key takeaways:
Controllers: One resource, one controller.
Models: Utilize associations and concerns.
Views: Leverage helpers and partials.
lib/
and Engines: Choose the right option based on the scope and reusability of your code.
Unleash the power of organization to keep your codebase manageable, maintainable, and most importantly, enjoyable to work with! ๐โจ
Got any tips or tricks of your own? Share your thoughts in the comments below. Let's level up our Rails game together! ๐
๐ฃ So, where do you put your code in Rails? Share your approach in the comments! ๐ฃ