Is Ruby pass by reference or by value?
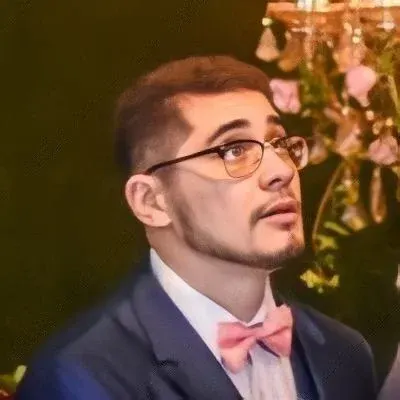
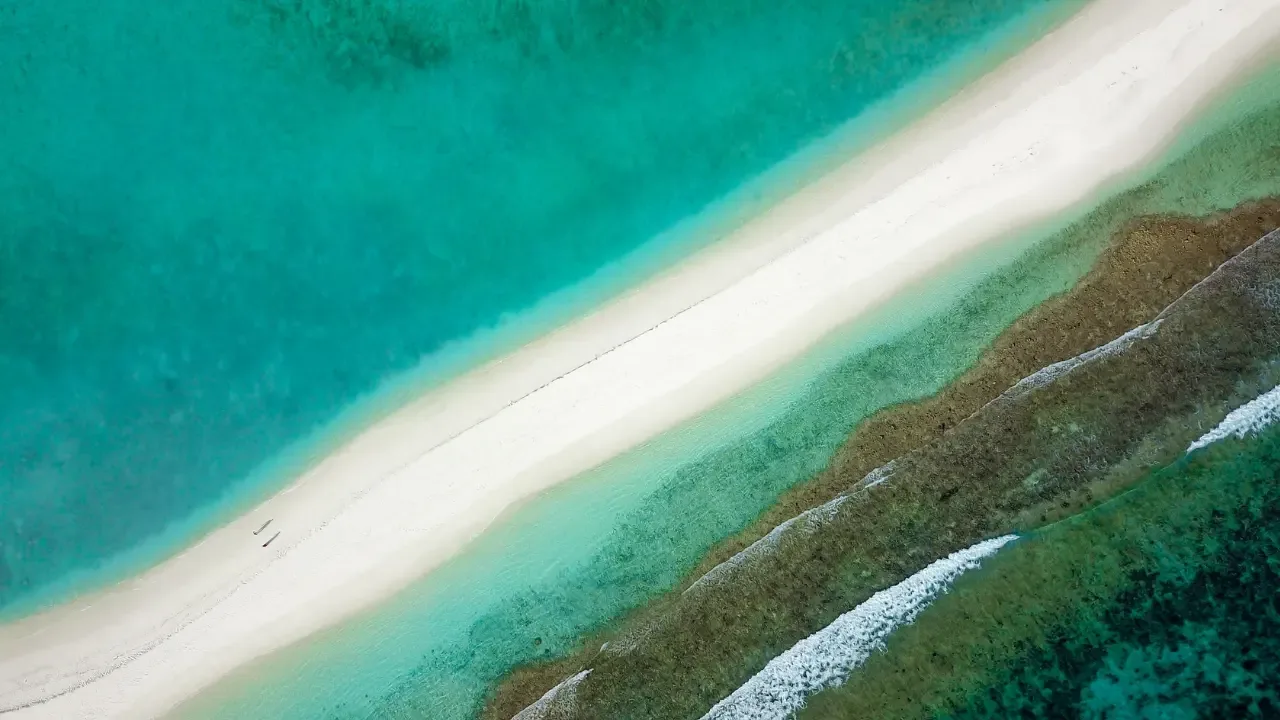
Is Ruby pass by reference or by value? 🤔
One of the most common questions among Ruby developers is whether Ruby is pass by reference or pass by value. Understanding how Ruby handles variable assignment and method arguments can be tricky, but fear not! In this post, we'll dive deep into this topic and provide easy-to-understand explanations and solutions to common issues. So, grab your favorite beverage ☕️ and let's get started!
The Context: Understanding the Problem 🤔
Let's begin by analyzing the provided code snippet. Here's what's going on:
@user.update_languages(params[:language][:language1],
params[:language][:language2],
params[:language][:language3])
lang_errors = @user.errors
logger.debug "--------------------LANG_ERRORS----------101-------------"
+ lang_errors.full_messages.inspect
if params[:user]
@user.state = params[:user][:state]
success = success & @user.save
end
logger.debug "--------------------LANG_ERRORS-------------102----------"
+ lang_errors.full_messages.inspect
if lang_errors.full_messages.empty?
It seems that the @user
object is adding errors to the lang_errors
variable in the update_languages
method. However, when performing a save on the @user
object, the errors initially stored in lang_errors
seem to disappear. 😞
Understanding Ruby's Pass by Reference/Value 🔄
To understand the behavior observed in the code snippet, we need to clarify Ruby's approach to pass by reference/value. 🤓
In Ruby, all variables are references to objects in memory. When passing a variable as an argument to a method, Ruby creates a copy of the reference to the same object. This leads to the misconception that Ruby is pass by value. However, if the method modifies the object itself, the changes will be visible outside the method, as both the original reference and the copied reference point to the same object.
The Mystery of the Washed-Out Variable Values 💦
Now that we know how Ruby handles variable assignment and method arguments, let's investigate why the lang_errors
variable is losing its values. 🕵️♀️
In the provided code, the lang_errors
variable is assigned the value of @user.errors
. However, when the @user
object is saved, the errors seemingly disappear. This is because the @user
object's save
method modifies the errors
object itself, replacing it with a new empty errors object.
FizzBuzz to the Rescue! 🚀
To demonstrate this behavior, let's do a quick FizzBuzz test with Ruby:
def fizz_buzz(str)
str.upcase!
str.gsub!('BUZZ', 'FIZZ')
str.reverse!
end
message = "Buzz"
fizz_buzz(message)
puts message
In this example, we're passing the message
variable to the fizz_buzz
method. Inside the method, we modify the str
object by applying several string transformations. When we print the value of message
outside the method, it will reflect the changes made within the method. This is because str
and message
are references to the same object.
Solutions: Preventing the Washout Effect 💡
To preserve the original errors stored in the lang_errors
variable, we need to make a copy of the errors
object before saving the @user
object. Here's how it can be done:
@user.update_languages(params[:language][:language1],
params[:language][:language2],
params[:language][:language3])
lang_errors = @user.errors.dup # Create a duplicate of the errors object
logger.debug "--------------------LANG_ERRORS----------101-------------"
+ lang_errors.full_messages.inspect
if params[:user]
@user.state = params[:user][:state]
success = success & @user.save
end
logger.debug "--------------------LANG_ERRORS-------------102----------"
+ lang_errors.full_messages.inspect
if lang_errors.full_messages.empty?
# Do something here
end
By using the dup
method, we create a separate instance of the errors
object, ensuring that modifying the original object within the @user
object's save
method does not affect the lang_errors
variable.
Your Turn: Engage and Learn More! 💬
Now it's your turn to take action! Apply the solution provided and see if it solves the issue you were facing. Experiment with different scenarios to solidify your understanding of Ruby's pass by reference/value.
If you have any questions or want to share your experiences, we'd love to hear from you! Leave a comment below ⬇️ and let's start a conversation.
Happy coding! 💻✨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
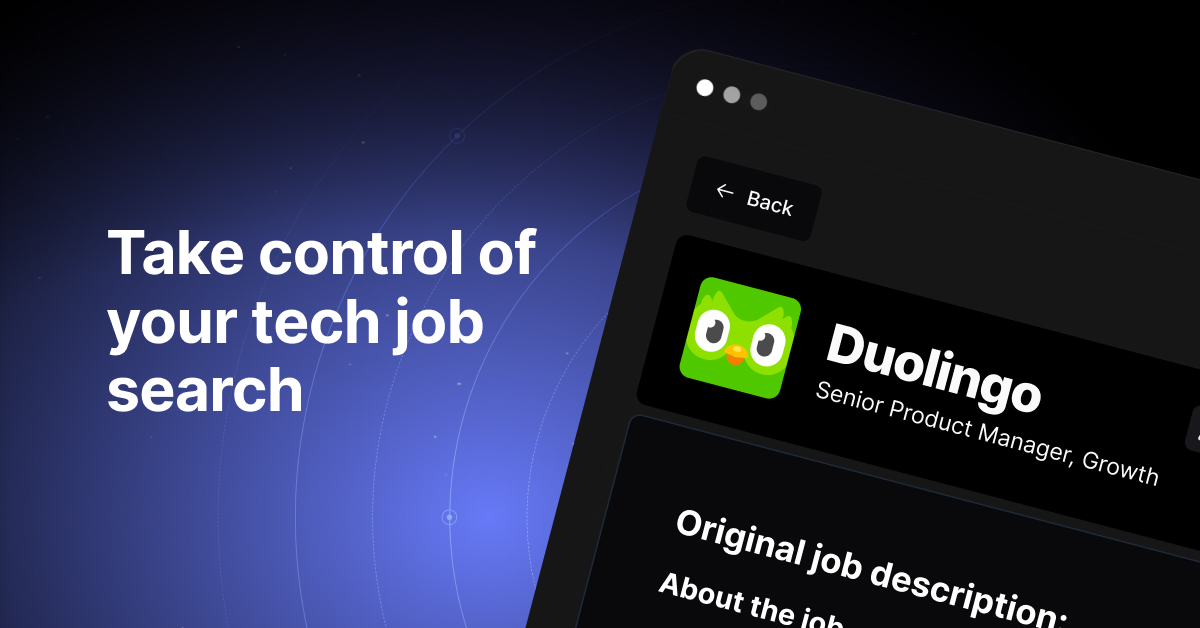