In Ruby on Rails, how do I format a date with the "th" suffix, as in, "Sun Oct 5th"?
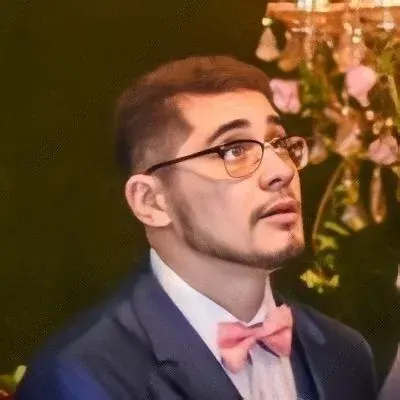
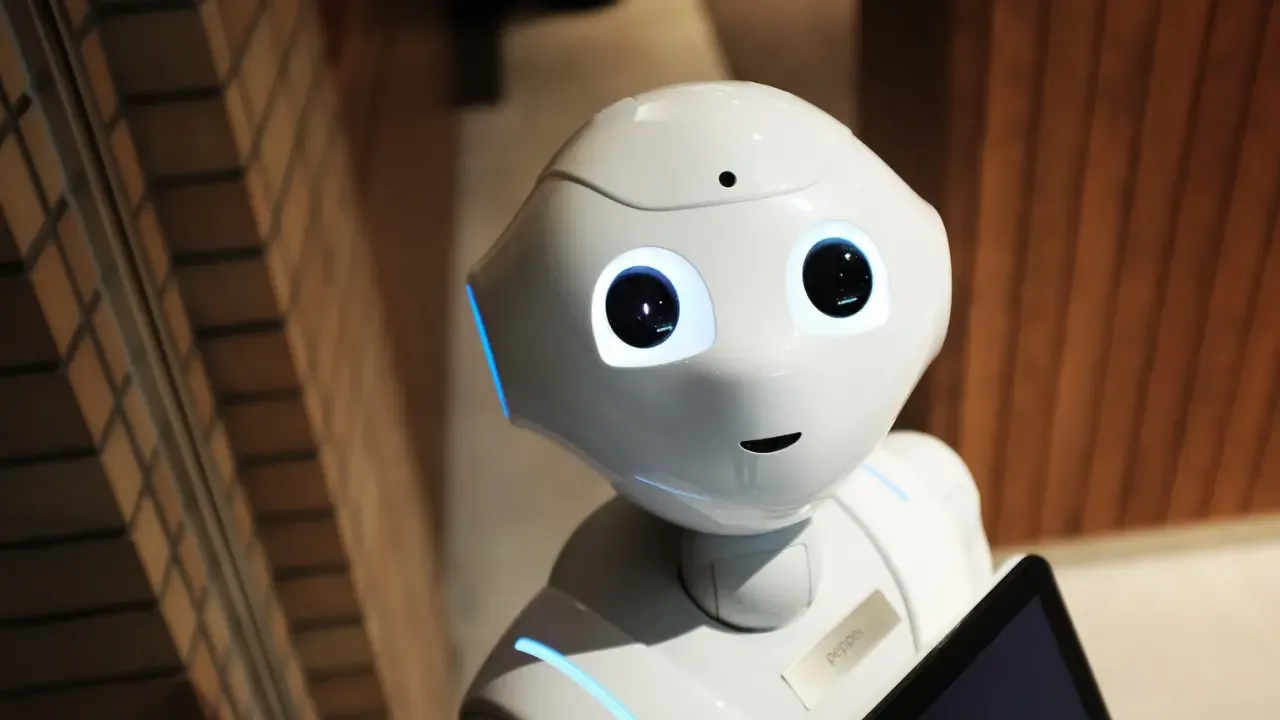
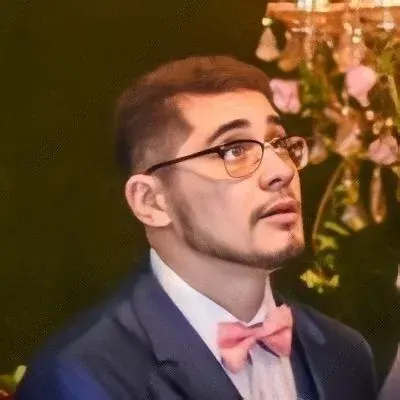
How to Format Dates with "th" Suffix in Ruby on Rails 📆💎
Have you ever wondered how to display dates with the "th" suffix in Ruby on Rails? You know, like "Sun Oct 5th" or "Thu Oct 2nd"? Look no further! In this blog post, we'll explore a common issue faced by Ruby on Rails developers and provide you with an easy solution. Let's dive right in! 💪🚀
The Problem 🤔
The challenge at hand is to format a date in the following format: short day of the week, short month, day of the month without leading zero, but including the "th", "st", "nd", or "rd" suffix. For example, "Thu Oct 2nd".
Unfortunately, the Time.strftime
method provided by Ruby's standard library doesn't directly support this requirement. This leaves us with the need for an alternative solution that achieves the desired output.
The Solution 💡
To accomplish this, we can make use of the strftime
method in conjunction with a custom format string. Here's how you can do it:
def formatted_date(date)
ord = date.day % 10 == 1 && date.day != 11 ? 'st' : date.day % 10 == 2 && date.day != 12 ? 'nd' : date.day % 10 == 3 && date.day != 13 ? 'rd' : 'th'
date.strftime("%a %b %-d") + ord
end
Let's break down how this solution works:
We define a method called
formatted_date
that takes adate
parameter, which represents the date we want to format.Inside the method, we determine the appropriate ordinal suffix for the day of the month using a ternary operator. We check if the day is not
11
,12
, or13
, and whether the day's remainder when divided by10
is equal to1
,2
, or3
, respectively.Next, we call
strftime
on thedate
object and pass in a format string. The%a
directive gives us the abbreviated day of the week (e.g., "Sun"),%b
gives us the abbreviated month (e.g., "Oct"), and%d
gives us the day of the month with a leading zero (e.g., "02").Finally, we concatenate the ordinal suffix determined earlier to the formatted date. The use of
-
befored
in the format string removes the leading zero from the day of the month.
With this solution, you can now easily format dates with the desired "th", "st", "nd", or "rd" suffix in Ruby on Rails! 🎉
Recap ⚡️
Here's a quick recap of the steps involved in formatting dates with the "th" suffix in Ruby on Rails:
Define a method that takes a
date
parameter.Determine the appropriate ordinal suffix for the day of the month.
Use
strftime
with a custom format string to format the date, including the day of the week, month, and day of the month without leading zero.Concatenate the ordinal suffix to the formatted date.
Take it to the Next Level! 💪🌟
Now that you've learned how to format dates with the "th" suffix, why not experiment further with date formatting options in Ruby on Rails? You can explore different directives and format strings provided by the strftime
method to customize and enhance your date display capabilities. Share your discoveries and creations in the comments below! We'd love to see what you come up with. 😄
Happy coding! 💻✨