How to use RSpec"s should_raise with any kind of exception?
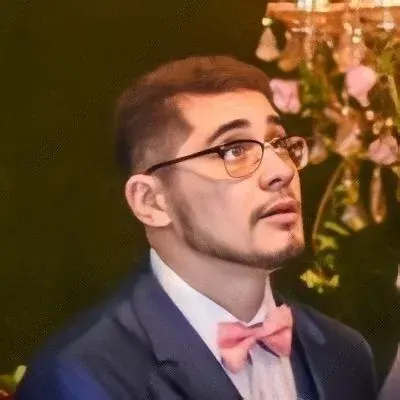
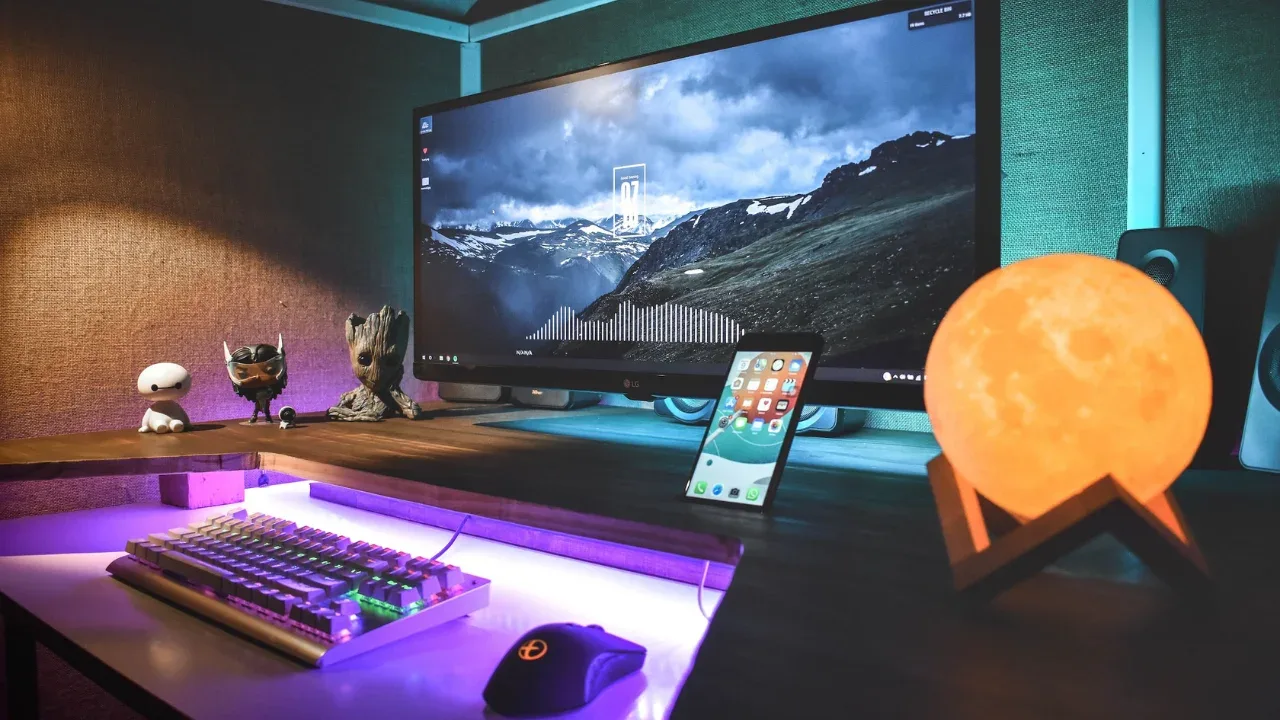
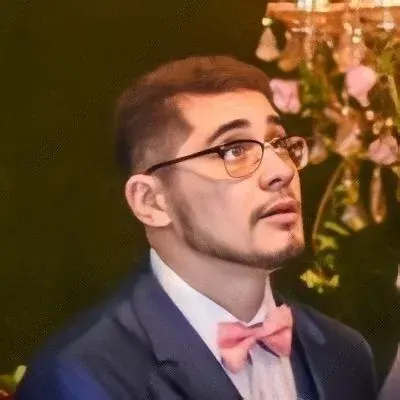
š Title: Mastering RSpec's should_raise: Handling Any Kind of Exception in Your Tests
š Hey there tech enthusiasts! Welcome back to our blog, where we share exciting tips and tricks to level up your coding skills. Today, we're diving into one of the common challenges that developers face when using RSpec's should_raise with any kind of exception. š¤
So, you're in a situation where you want to use RSpec's should_raise, but you don't really care about the specific exception being raised. You just need to ensure that an exception is raised in your tests. Here's how you can overcome this hurdle with ease. šŖ
š The Problem at Hand
You may have tried something like this:
some_method.should_raise <any kind of exception, I don't care>
But unfortunately, it doesn't work as expected. š
š ļø Easy Solution
But fear not, fellow developer! There's a straightforward solution to this problem. Instead of trying to match against any exception explicitly, we can leverage Ruby's StandardError
to achieve the desired behavior. š
Here's an updated snippet that will make your tests pass:
expect { some_method }.to raise_error(StandardError)
By using raise_error
matcher and specifying StandardError
as the expected error, you're essentially asserting that an exception of any kind, derived from StandardError
, should be raised.
š Example Usage
Let's see some practical examples to solidify our understanding.
# Example 1: Testing an exception is raised
expect { 1 / 0 }.to raise_error(StandardError)
# Example 2: Testing an exception is raised within a block
expect do
File.open('non_existent_file.txt')
end.to raise_error(StandardError)
# Example 3: Testing an exception is raised with custom message
expect { some_method }.to raise_error(StandardError, 'This is a custom error message')
Feel free to customize the code snippets according to your specific use cases.
š£ Take Action: Engage and Share!
Congratulations! š You've now mastered the art of using RSpec's should_raise with any kind of exception. Go ahead and give it a spin in your testing suite. We're confident that this technique will enhance your code coverage and help you catch those pesky bugs.
If you found this article helpful, don't forget to share it with your fellow developers. Let's spread the knowledge and empower the tech community together! šŖš
We'd love to hear your thoughts and experiences. Have you encountered any challenges while using should_raise? Or maybe you have an alternative approach? Share your thoughts in the comments below and let's start a conversation. Happy coding! šš