How to test if parameters exist in rails
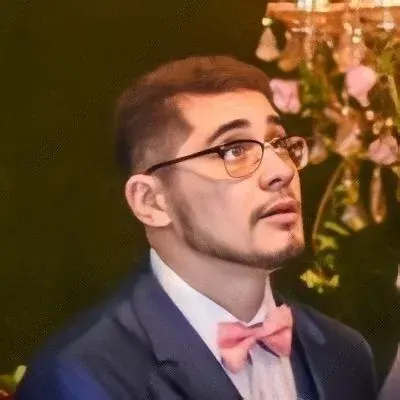
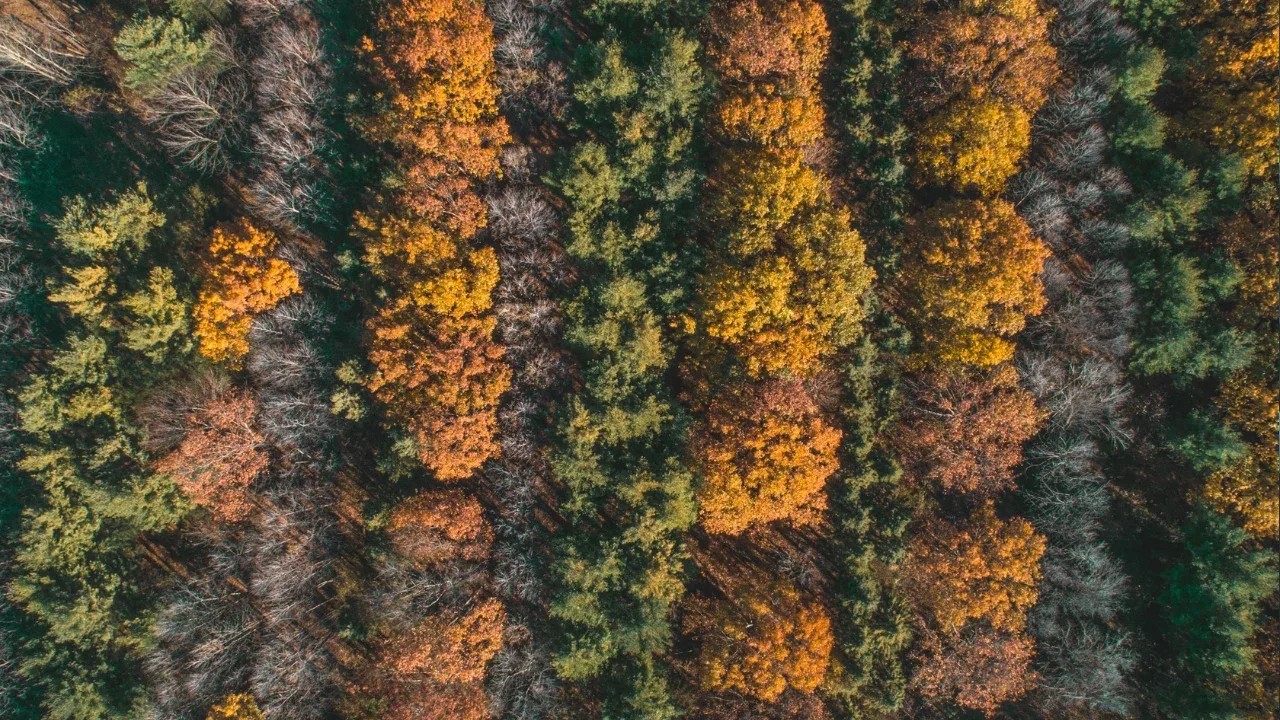
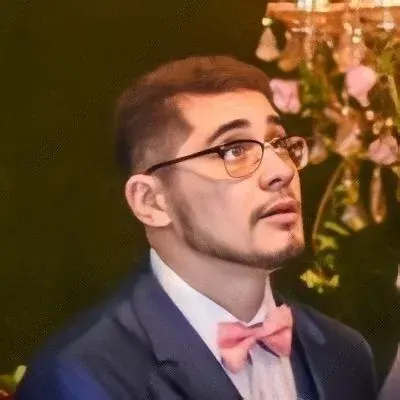
Testing if Parameters Exist in Rails: A Comprehensive Guide ๐๐ง
Are you struggling to test whether specific request parameters are set in your Ruby on Rails application? ๐ค Don't worry, you're not alone! This blog post aims to address this common issue and provide you with easy solutions to accurately determine if both params[:one] and params[:two] are set. Let's dive right in! ๐ป๐
The Problem: Misbehaving IF Statement ๐คจ
So, you have an IF statement in your Rails application, but regardless of whether both parameters are set, the first part of the block always gets triggered. This behavior can be quite frustrating, especially when you want to perform different actions based on the presence of both parameters. Let's take a look at the code snippet in question:
if (defined? params[:one]) && (defined? params[:two])
... do something ...
elsif (defined? params[:one])
... do something ...
end
Understanding the Issue ๐
The problem lies in the usage of the defined?
keyword. In this context, defined?
checks if a variable is defined, but it doesn't verify if the variable has a value or if the parameter exists in the request. It simply returns nil
if the parameter is not defined, leading to unexpected behavior.
Solution 1: Testing for Presence Using .present?
โ๏ธ
A more reliable approach is to use the .present?
method to check if the parameters exist. Let's refactor the code snippet to incorporate this solution:
if params[:one].present? && params[:two].present?
... do something ...
elsif params[:one].present?
... do something ...
end
By using .present?
, the code will only execute the corresponding block if both params[:one]
and params[:two]
have values.
Solution 2: Utilizing the &&
Operator Correctly ๐ค
Alternatively, you can modify the initial IF statement to correctly leverage the &&
operator:
if (defined?(params[:one]) && defined?(params[:two])) && (params[:one].present? && params[:two].present?)
... do something ...
elsif params[:one].present?
... do something ...
end
This solution checks if the variables are defined and if their values are also present. It provides a more explicit condition that ensures both parameters exist.
Call-to-Action: Share Your Experience and Learn More! ๐ข๐
Now that you have two reliable solutions to test the existence of parameters in Ruby on Rails, it's time to put them into action! Try implementing the suggested code snippets in your own project and see the difference.
Share your experience using the hashtag #RailsParamTesting and let the tech community know how it worked for you. Additionally, if you have any further questions or want to learn more about Rails development, leave a comment below or visit our website's community forum. Let's keep the conversation going! ๐ฉโ๐ป๐จโ๐ป
Happy coding! ๐ก๐ป