How to remove a key from Hash and get the remaining hash in Ruby/Rails?
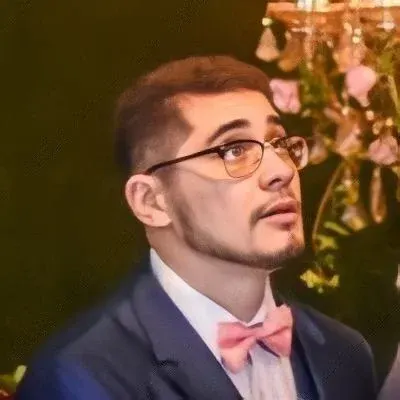
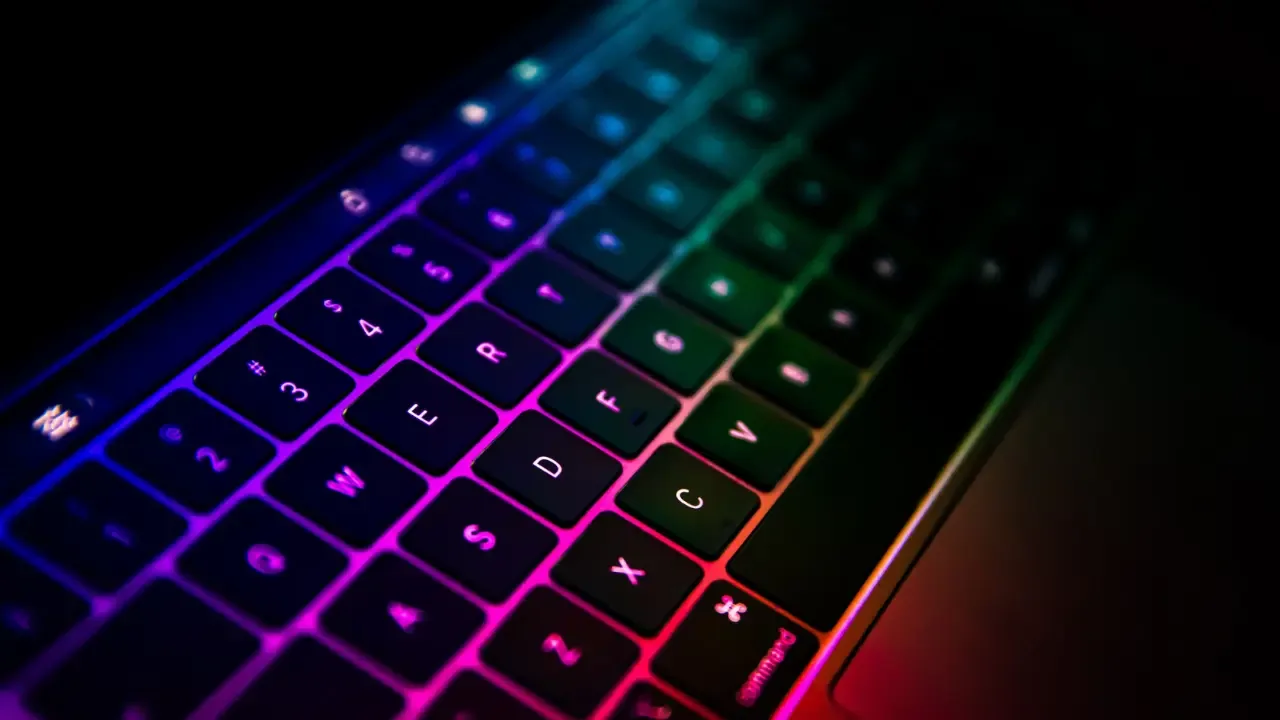
How to Remove a Key from Hash and Get the Remaining Hash in Ruby/Rails 🗝️🗑️
So you have a Hash in Ruby/Rails and you want to remove a specific key from it, but you also want to get the remaining hash after the removal. You're not alone! Many developers have encountered this problem and have come up with different solutions. In this blog post, we'll explore some common issues and provide easy solutions to help you tackle this challenge. Let's dive in! 💪
The Challenge: Removing a Key from Hash 🚧
By default, Ruby provides the delete
method to remove a key-value pair from a Hash. However, this method only returns the value of the deleted key, not the modified Hash itself. This can be inconvenient if you need to perform additional operations on the modified Hash in a single line of code.
Solution 1: Using the delete
Method and Assignment Operator ⚙️
One way to solve this problem is by using the delete
method and the assignment operator. Here's an example:
my_hash = { a: 1, b: 2 }
my_key = :a
my_hash.delete(my_key)
remaining_hash = my_hash # Assign the modified Hash to another variable
puts remaining_hash # Output: { b: 2 }
By assigning the modified Hash to another variable (remaining_hash
in this example), you can easily access and work with the remaining Hash in subsequent lines of code.
Solution 2: Utilizing the tap
Method and Block 🚀
If you prefer a more concise solution, you can use the tap
method along with a block. This approach allows you to chain multiple operations together in a single line. Here's an example:
my_hash = { a: 1, b: 2 }
my_key = :a
remaining_hash = my_hash.tap { |hash| hash.delete(my_key) }
puts remaining_hash # Output: { b: 2 }
By using the tap
method, the modified Hash is passed to the block as a parameter (hash
in this example), and you can perform any additional operations on it within the block. The final result is then assigned to the remaining_hash
variable.
Solution 3: Implementing a Custom Method in Ruby/Rails 🛠️
If you find yourself frequently performing this operation and want a more reusable solution, you can define a custom method in Ruby/Rails. Here's an example implementation:
class Hash
def delete_and_return(key)
delete(key)
self
end
end
With this custom method, you can simply call delete_and_return
on any Hash instance, passing in the desired key to be deleted. The modified Hash is automatically returned, enabling you to perform further operations seamlessly:
my_hash = { a: 1, b: 2 }
my_key = :a
remaining_hash = my_hash.delete_and_return(my_key)
puts remaining_hash # Output: { b: 2 }
Conclusion and Your Next Step 🎉
Removing a key from a Hash and obtaining the remaining Hash can be achieved through various approaches. Whether you choose to use the delete
method with assignment, leverage the tap
method and block, or define a custom method, make sure to pick the solution that best suits your needs.
Now it's your turn to try these techniques in your Ruby/Rails projects. Experiment with different scenarios and see which solution works best for you. Feel free to share your experiences and any additional solutions you come up with in the comments below. Happy coding! 💻✨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
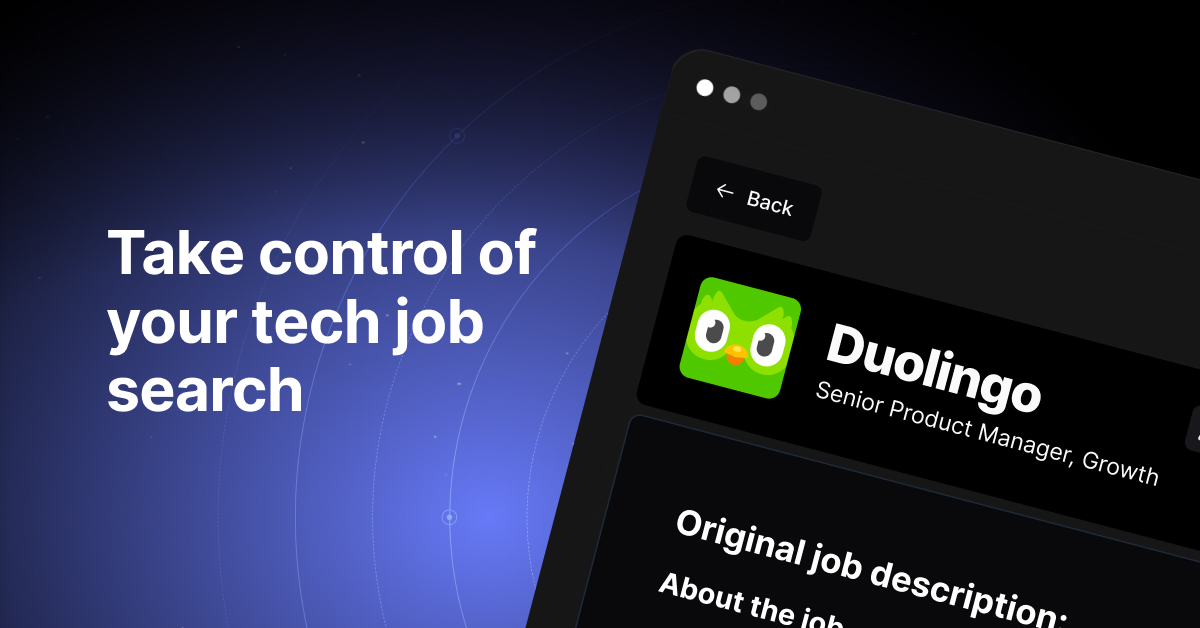