How to redirect to previous page in Ruby On Rails?
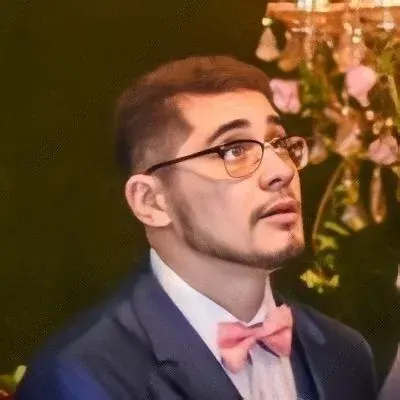
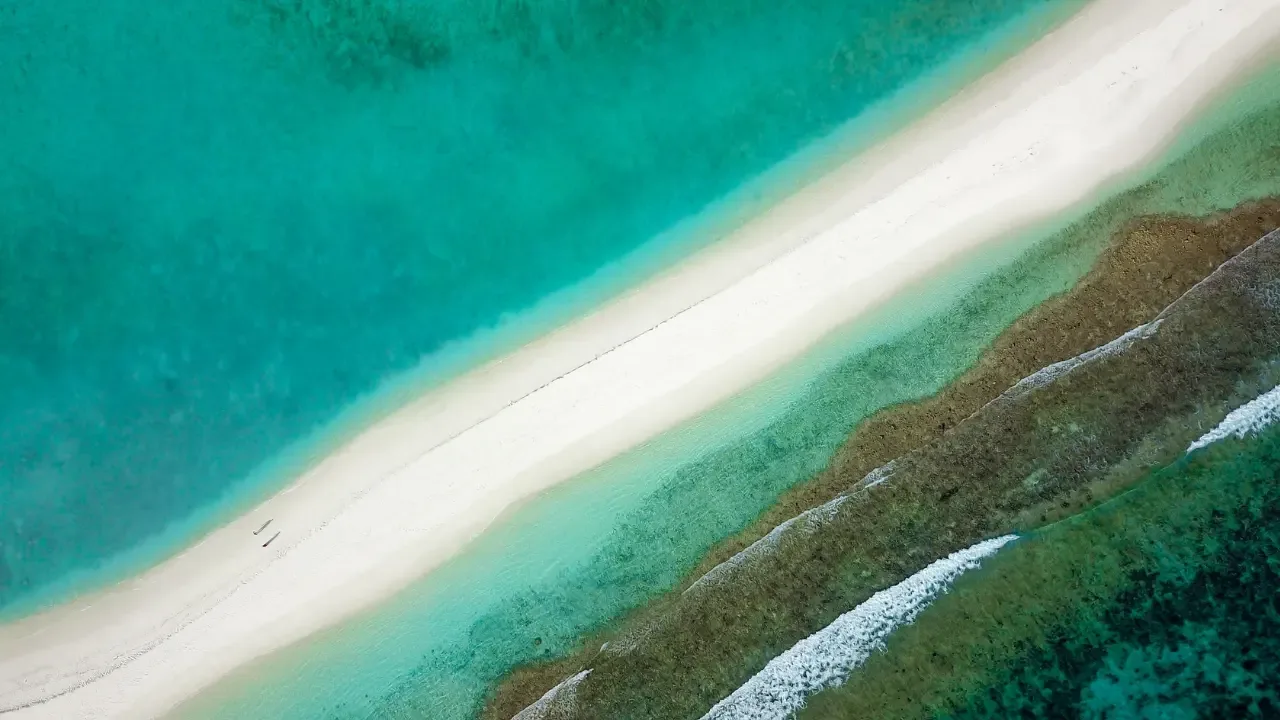
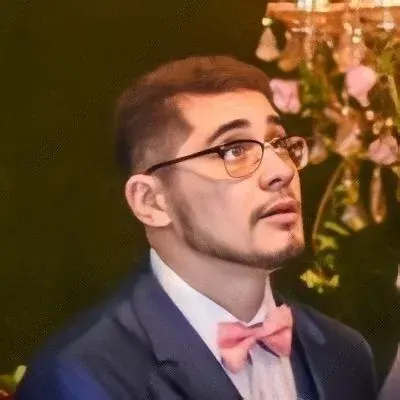
🚀 How to Redirect to Previous Page in Ruby On Rails?
So, you're trying to redirect back to the previous page in your Ruby on Rails application? You want to ensure that after updating some data, you're redirected to the exact same page with the same sorting and filters. Well, you're in luck! We've got the solution for you.
📌 The Challenge
Let's understand the problem you're facing. You have a page that lists projects with sortable headers and pagination. When you choose to edit one of the projects, you're taken to a new page where you can make your changes. After updating the project, you want to be redirected back to the previous page with the same sorting and pagination intact.
🔍 The Solution
The redirect_back
method comes to the rescue! This method allows you to redirect the user back to the page from which they came. In your case, it will take you back to the project list page with the same sorting and pagination.
Here's how you can use it in your Ruby on Rails application:
def update
# Your update logic here
redirect_back(fallback_location: root_path)
end
🔎 Understanding the Code
The
redirect_back
method takes an optionalfallback_location
parameter, which specifies the default URL to redirect to if the previous URL is unknown. In this example, we're usingroot_path
as the fallback location, but you can replace it with any URL of your choice.By default,
redirect_back
uses therequest.referrer
value to determine the previous URL. It looks for theReferer
header in the HTTP request, which is usually set by the client. If theReferer
header is missing or invalid, thefallback_location
will be used.
💡 Pro Tips
Handling Different Controllers or Actions: If you want to redirect to a specific controller or action other than the previous one, you can use the
redirect_to
method with the desired URL. For example:def update # Your update logic here redirect_to projects_url(order: 'asc', page: 3, sort: 'code') end
Dealing with AJAX Requests: If you're using AJAX to update your data,
redirect_back
may not be applicable. In such cases, you can send a JSON response from your controller and handle redirection on the client-side using JavaScript.
🎯 Call-to-Action
Now that you know how to redirect back to the previous page in your Ruby on Rails application, it's time to implement it and save yourself from the hassle of manually tracking and redirecting. Give it a try and let us know how it works for you!
Have any other Ruby on Rails questions or challenges? Let us know in the comments below. Happy coding! 💻🚀