How to "pretty" format JSON output in Ruby on Rails
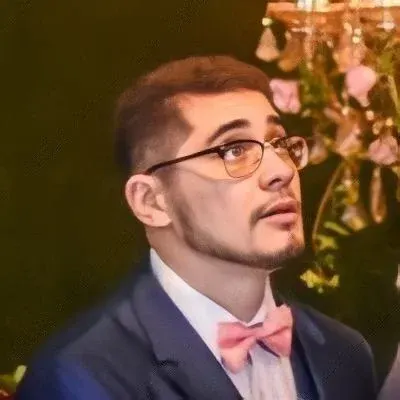

Formatting JSON Output in Ruby on Rails: A Pretty Solution 🌟
Are you tired of dealing with messy and unreadable JSON output in your Ruby on Rails applications? Do you find it challenging to identify issues in your JSON output stream? Fret not! In this blog post, we'll explore how you can format your JSON output to be visually appealing and easy to read. 💎🔮
The Problem: Messy JSON Output 🤔
By default, when we call the to_json
method in Ruby on Rails, the resulting JSON is all on one line. This lack of formatting can make it difficult to spot errors or analyze the structure of the JSON. Here's an example:
{"name":"John Doe","age":25,"email":"johndoe@example.com"}
As you can see, all the key-value pairs are cramped together, which makes it challenging to identify any inconsistencies or missing data. 🚫📦
The Solution: Pretty Formatting 🎁🎨
Thankfully, Rails provides an easy way to configure your JSON output to be "pretty" or nicely formatted. The secret lies in using the JSON.pretty_generate
method. Let's dive right in with an example:
json_data = {"name":"John Doe","age":25,"email":"johndoe@example.com"}
pretty_json = JSON.pretty_generate(json_data)
puts pretty_json
By using JSON.pretty_generate
, we can obtain a much more readable and beautifully formatted JSON output:
{
"name": "John Doe",
"age": 25,
"email": "johndoe@example.com"
}
Voila! 🎉✨ Now we have a JSON output that's neatly organized with each key-value pair on its own line, indented properly for better visibility.
Implementation: Updating Your Rails Code 🚀
To apply pretty formatting to your JSON output throughout your Rails application, there are a couple of strategies you can employ.
1. To Pretty-print All JSON Responses 💫
If you want to configure Rails to pretty-print all JSON responses by default, you can add the following code to your config/application.rb
file:
config.middleware.use "PrettyJsonMiddleware"
The PrettyJsonMiddleware
is a custom middleware that injects pretty formatting for all JSON responses. You can implement it as follows:
class PrettyJsonMiddleware
def initialize(app)
@app = app
end
def call(env)
status, headers, response = @app.call(env)
if headers['Content-Type']&.include?('application/json')
body = JSON.parse(response.body)
pretty_body = JSON.pretty_generate(body)
response = [pretty_body]
headers['Content-Length'] = pretty_body.bytesize.to_s
end
[status, headers, response]
end
end
After making these changes, all your JSON responses will be automatically pretty-formatted. 🖌️🔍
2. To Pretty-print Specific JSON Responses ✨
In some cases, you might only want to pretty-print specific JSON responses. You can achieve this by modifying your controller code as shown below:
def show
# Fetch data and generate JSON
json_data = @model.to_json
# Render pretty-formatted JSON
render json: JSON.pretty_generate(JSON.parse(json_data))
end
By using JSON.parse
and JSON.pretty_generate
, you can transform the JSON output into its visually appealing counterpart, limited to specific actions or responses.
Your Turn: Engage and Share! 📣
Now that you know how to "pretty" format JSON output in Ruby on Rails, it's time to put this knowledge into action! Go ahead and apply these techniques to your own applications and enjoy the beauty and readability of your JSON responses. 💅👀
If you found this guide helpful, share it with your fellow Rubyists and fellow developers who struggle with messy JSON output. Let's make the JSON world a prettier place! 💖🌍
Feel free to leave a comment below and let me know if you have any questions or if there's anything else you'd like to learn about. Happy coding! ✌️😊
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
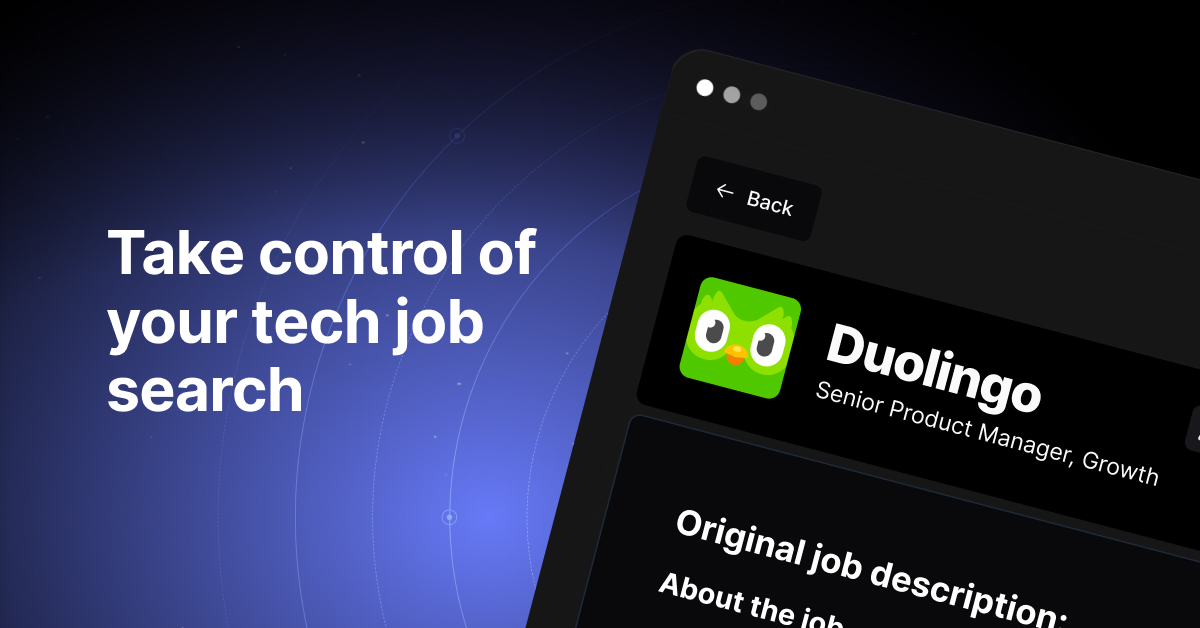