How to convert a ruby hash object to JSON?
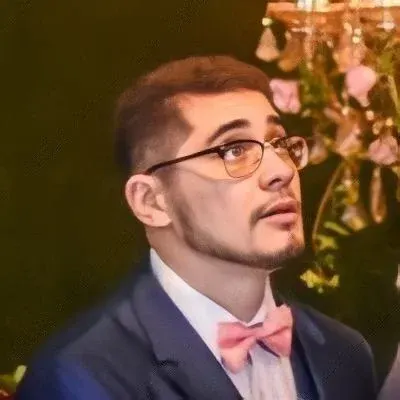
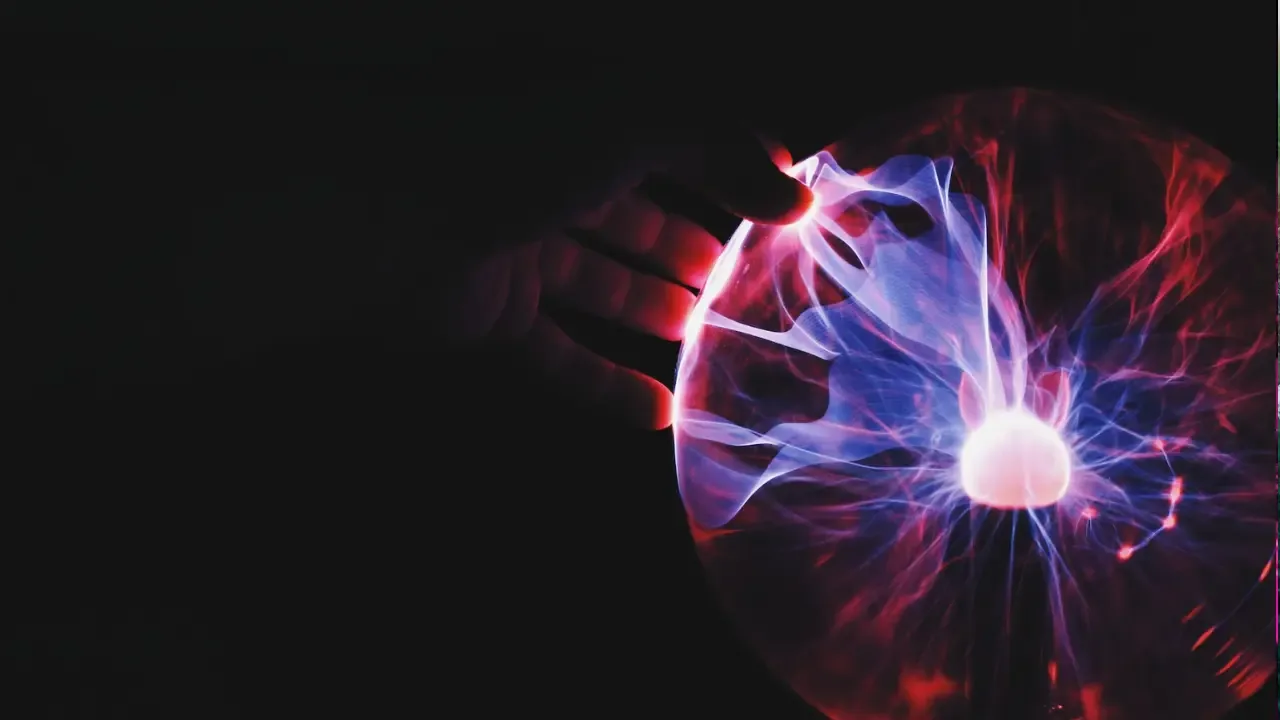
🖐️ Hey there tech enthusiasts! Are you struggling to convert a Ruby hash object to JSON? Don't worry, we've got you covered! 🌟
So, you have this Ruby hash object, and you want to convert it to JSON. You might have tried using the to_json
method on the hash object, but sadly, it didn't work for you. 😞 Let's dive into the issue and find the perfect solution! 💪
First, let's clarify something. The Hash
object itself doesn't have a to_json
method. However, in Rails, you can indeed use to_json
on a hash object. How? 🤔
Well, it's because Rails extends the functionality of the Hash
object by adding additional features like to_json
. But, in pure Ruby, without Rails, you won't find this method available. 🚫
But fear not! We have a simple and elegant solution for you! 🎉 You can use the JSON
module that's built right into Ruby. Here's how you can do it: 😉
require 'json'
car = { make: "bmw", year: "2003" }
car_json = JSON.generate(car)
puts car_json
In the code above, we first require the json
library, which gives us access to the JSON
module. Then, we create our car
hash object. Using JSON.generate
, we convert the hash object to JSON. Finally, we can print out the resulting JSON. 🚀
Now you can successfully convert your Ruby hash object to JSON without relying on Rails-specific methods. Pretty cool, right? 😎
But wait, there's more! If you're using Ruby on Rails, you have an even simpler option. Rails provides an incredibly handy method called to_json
for ActiveRecord objects, which includes the Hash
object. Here's an example: 🌈
require 'active_record'
class Car < ActiveRecord::Base
end
car = Car.new(make: "bmw", year: "2003")
car_json = car.to_json
puts car_json
In this example, we create a Car
model using ActiveRecord. We then create a new instance of the Car
model with the desired attributes. By calling to_json
on the car
object, Rails automatically converts the Hash
object to JSON for us. How convenient! 😄
So whether you're using pure Ruby or Ruby on Rails, we've given you two fantastic solutions to convert your hash object to JSON. 🎊
We hope this guide helped you overcome any obstacles you were facing. If you have any further questions or suggestions, feel free to share them in the comments below. Let's keep the conversation going! 💬
Now it's your turn! Try out the code snippets, experiment, and let us know how it goes. Start converting those hash objects to JSON like a pro! 🚀
Keep coding! ✨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
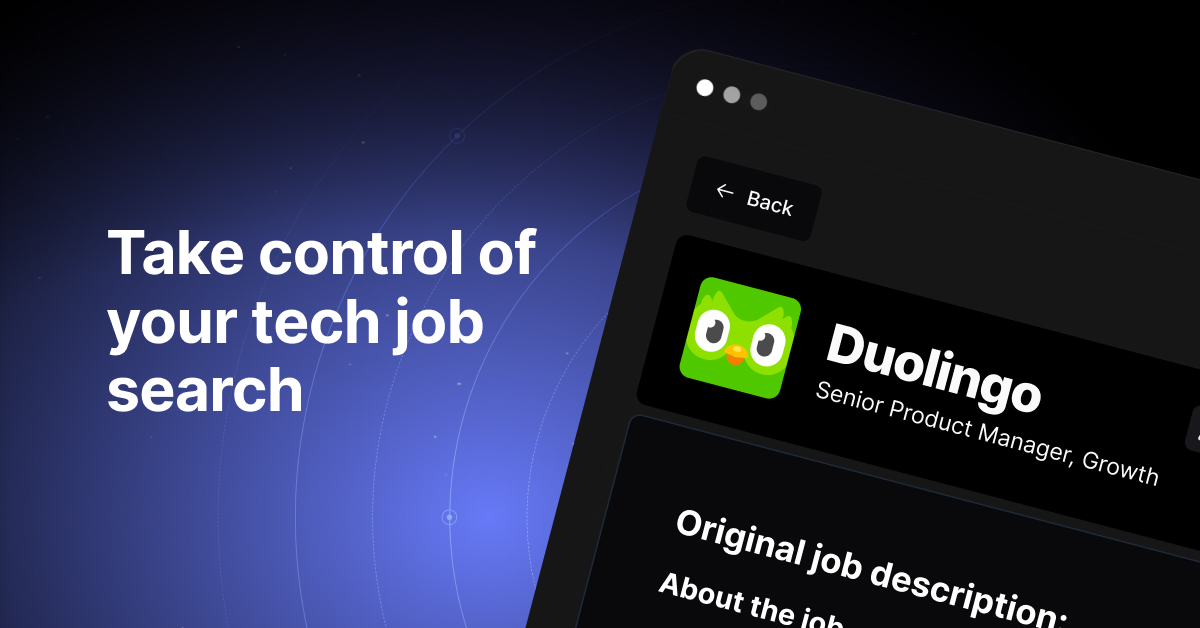