How to change a nullable column to not nullable in a Rails migration?
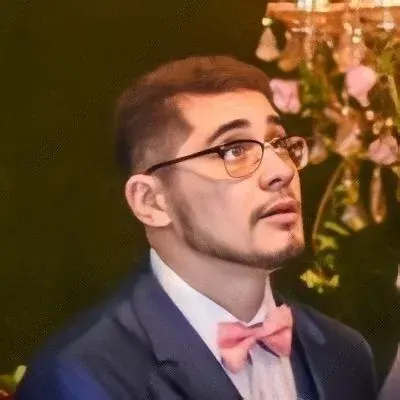
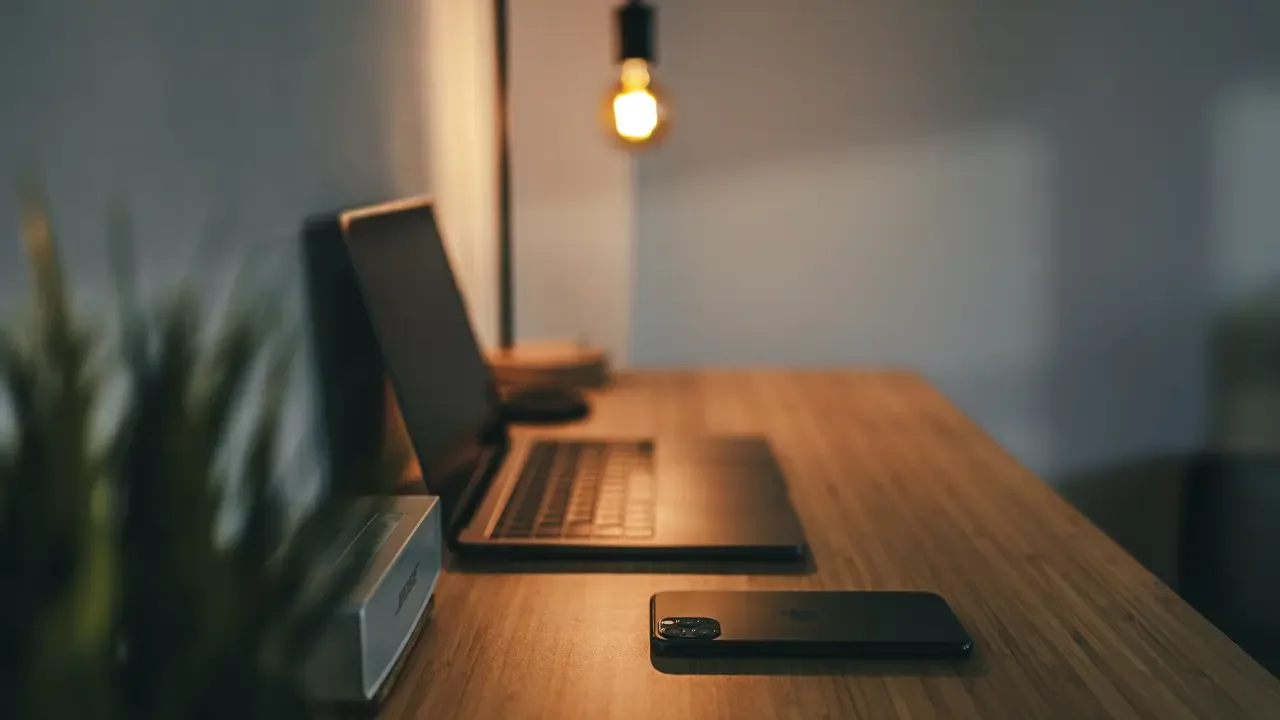
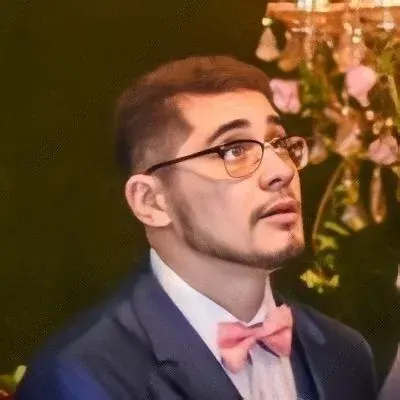
The Ultimate Guide to Changing a Nullable Column to Not Nullable in a Rails Migration
Are you struggling with changing a nullable column to not nullable in a Rails migration? Don't worry, we've got you covered! π οΈπ‘
The Scenario
Imagine this scenario: you created a date column in a previous migration and set it to be nullable. Now, you want to change it to not nullable. However, there are null rows in that database, and you're okay with setting those columns to the current time (Time.now) if they are currently null.
Common Issues
Before diving into the solution, let's address some common issues you might encounter:
1. Error: Default column value
If you try to change a nullable column to not nullable without setting a default value, you might encounter an error. Rails requires a default value for non-nullable columns to avoid breaking existing data integrity.
2. Existing null entries
If your nullable column has null entries, you need to handle those before making the column not nullable. Failing to do so will result in errors during the migration process.
Easy Solutions
To address these common issues and successfully change a nullable column to not nullable, follow these steps:
Step 1: Add a default value
In your migration file, add a default value for the nullable column using the :default
option. This will prevent any potential errors from occurring during the migration.
change_column :table_name, :column_name, :data_type, default: -> { 'CURRENT_TIMESTAMP' }
In the above example, we are using the CURRENT_TIMESTAMP
SQL function as the default value, which sets the column to the current timestamp.
Step 2: Update existing null entries
Next, update any null entries in the column with the desired value. In this case, we will set them to the current time using Time.now
.
Model.where(column_name: nil).update_all(column_name: Time.now)
Replace Model
with the name of your ActiveRecord model, and column_name
with the name of the column you're modifying.
Step 3: Change column to not nullable
Now that we have taken care of the default value and updated the existing null entries, it's time to change the column to not nullable.
change_column_null :table_name, :column_name, false
This line of code will make the column not nullable, ensuring that future entries cannot be null.
Call-To-Action and Reader Engagement
Congratulations! You've successfully changed a nullable column to not nullable in a Rails migration. Now itβs time to go out there and apply this knowledge to your own projects! ππͺ
Remember, if you found this guide helpful or have any further questions, leave a comment below and share this post with your fellow developers. Let's connect and help each other grow in our coding journey. Happy coding! πβ¨