How can I download a file from a URL and save it in Rails?
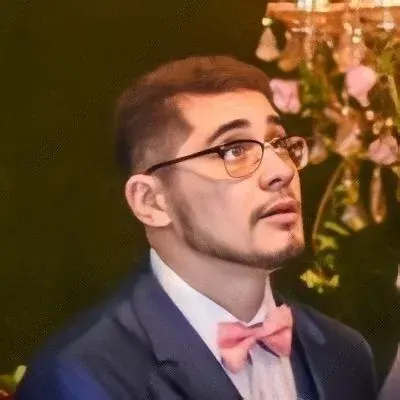
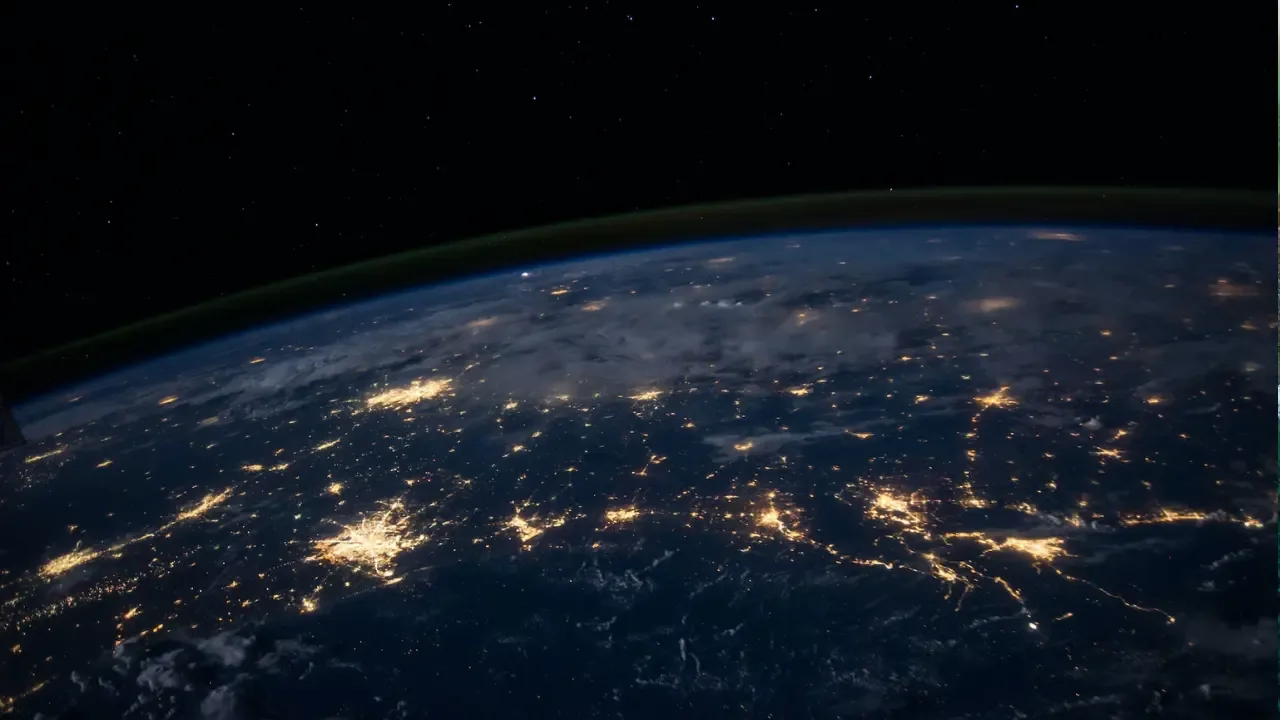
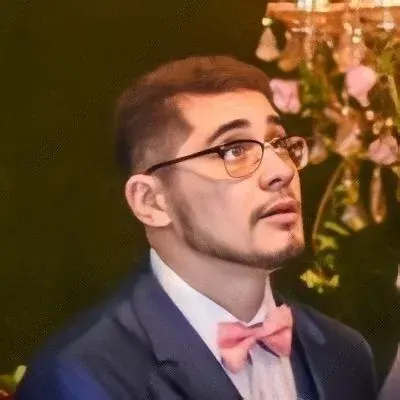
How to Easily Download and Save Files from a URL in Rails 🚀
Are you struggling to download and save files from a URL in your Rails application? You're not alone! Many developers face this challenge when working with remote resources like images, files, or even videos. But fear not, because I've got you covered! In this blog post, I'll walk you through the process of downloading and saving a file from a URL in Rails, giving you easy solutions to tackle this problem. 🌟
The Problem at Hand 💡
Let's start by understanding the specific dilemma you're facing. In your case, you have a URL pointing to an image that you want to save locally. This is a common scenario when working with image processing libraries like Paperclip, which require files to be stored locally for thumbnail generation. However, you haven't been able to find any relevant solutions using Ruby file handling. So, what's the best way to proceed? 🤔
Solution 1: Using the open-uri
Library ✅
The good news is that Ruby provides a convenient library called open-uri
that makes downloading files from a URL a breeze. Here's how you can use it to solve your problem:
require 'open-uri'
def download_file(url, destination)
open(url) do |file|
IO.copy_stream(file, destination)
end
end
download_file('https://example.com/image.jpg', 'path/to/local/image.jpg')
In this solution, we use the open
method from the open-uri
library to open the URL and obtain a file object. We then use IO.copy_stream
to copy the contents of the file to the specified local destination. That's it! You've successfully downloaded and saved the file from the provided URL. 🎉
Solution 2: Leveraging HTTP Client Gems 🌐
If you prefer a more advanced approach or need additional features, you can also consider using HTTP client gems like HTTParty
, Faraday
, or Net::HTTP
. These gems provide higher-level abstractions and additional functionality for interacting with remote resources, including file downloads. Here's an example using the HTTParty
gem:
require 'httparty'
def download_file(url, destination)
response = HTTParty.get(url)
File.open(destination, 'wb') do |file|
file.write(response.body)
end
end
download_file('https://example.com/image.jpg', 'path/to/local/image.jpg')
In this solution, we use the HTTParty.get
method to send an HTTP GET request to the specified URL and retrieve the response. We then open a local file using File.open
, set it to binary mode ('wb'
), and write the response body to the file. Voila! You've successfully saved the file locally using the HTTParty
gem. 🙌
Call-to-Action: Share Your Success Story! 📢
I hope these solutions have helped you overcome the challenge of downloading and saving files from a URL in your Rails application. Give them a try and let me know how it goes! If you have any other tips, tricks, or alternative solutions, feel free to leave a comment below and share your expertise with the community. Together, we can make Rails development even better! 😊
Have you ever struggled with downloading files from a URL in your Rails app? I've got you covered with easy solutions! Check out my latest blog post: [INSERT LINK] #RailsDevelopment #RubyOnRails
Happy coding! 💻🔥