Do rails rake tasks provide access to ActiveRecord models?
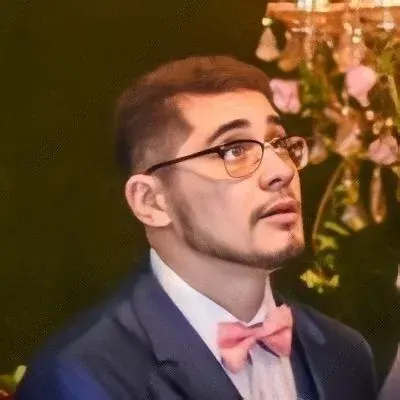
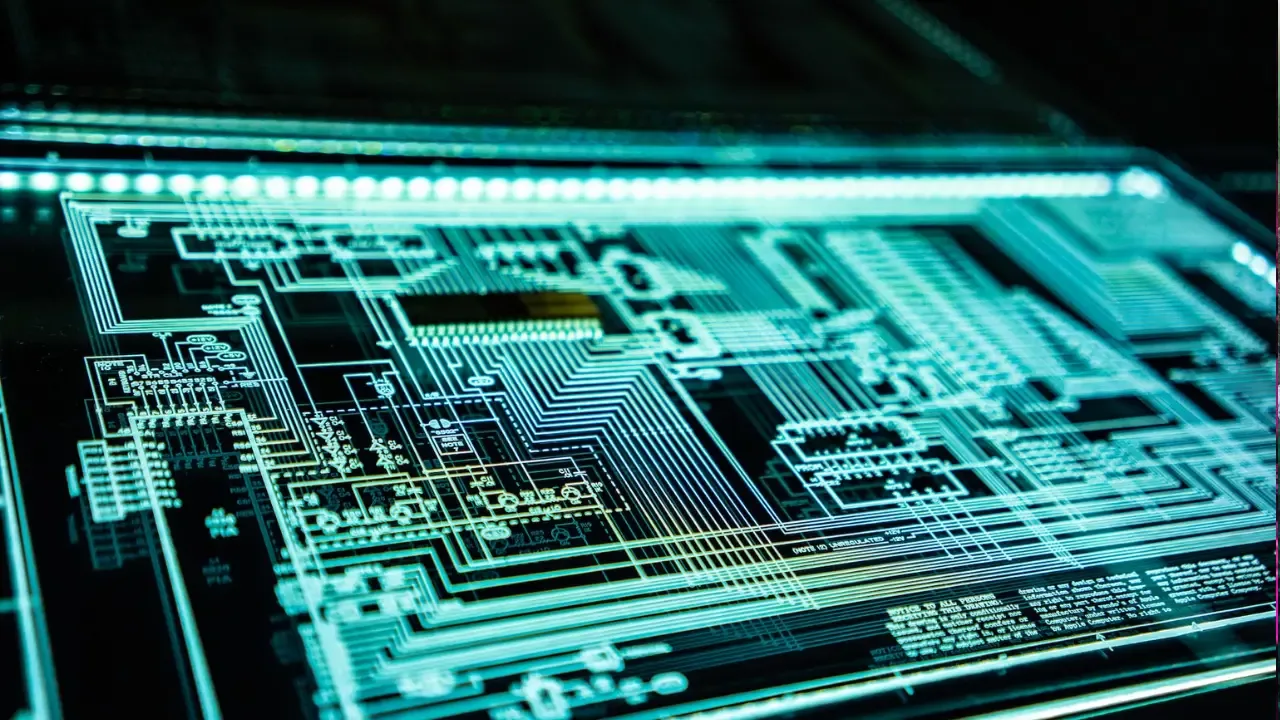
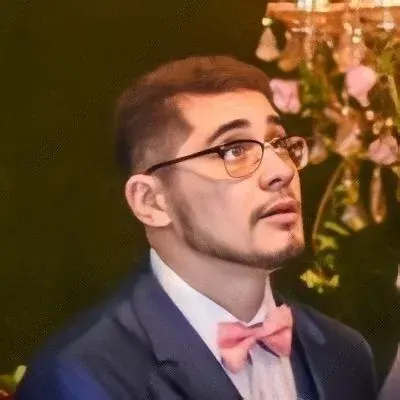
🚀 Why Rails Rake Tasks Don't Provide Access to ActiveRecord Models
So, you're trying to create a custom rake task in your Rails application, but it seems like you don't have access to your models? You might be wondering why this is happening, especially when it's something that's implicitly included with Rails tasks. Don't worry, you're not alone in facing this issue.
🤔 The Problem
Let's take a look at the code snippet you provided:
namespace :test do
task :new_task do
puts Parent.all.inspect
end
end
And the Parent
model:
class Parent < ActiveRecord::Base
has_many :children
end
Seems pretty straightforward, right? But when you run the rake task rake test:new_task
, you encounter the dreaded error: uninitialized constant Parent
.
💡 The Explanation
The reason why you're seeing this error is that rake tasks do not automatically load the Rails environment, which means that your models are not loaded as well. This behavior is different from the usual Rails operations where the environment is loaded by default.
🚀 The Solution
To access your ActiveRecord models in rake tasks, we need to explicitly require the Rails environment. We can do this by adding the following line at the beginning of your lib/tasks/test.rake
file:
task :environment do
require_relative '../../config/environment'
end
This code will load up the Rails environment, making your models available within the rake task.
🎉 Time to Celebrate
With the task :environment
block in place, your updated rake task should now look like this:
namespace :test do
task :environment do
require_relative '../../config/environment'
end
task :new_task => :environment do
puts Parent.all.inspect
end
end
🙌 Hooray! You should now be able to run your rake task rake test:new_task
successfully without encountering the "uninitialized constant" error.
📢 Your Turn to Share
We hope this guide helped you understand why Rails rake tasks don't provide access to ActiveRecord models and how to overcome this issue. If you found this information useful, be sure to tweet it 🐦, share it on Facebook 📘, or spread the word however you like 🌍.
Have you encountered any similar issues or found other creative ways to handle this situation? Feel free to share your experiences and insights in the comments section below. Together, we can conquer any roadblocks in our Rails journey! 💪