Determine what attributes were changed in Rails after_save callback?
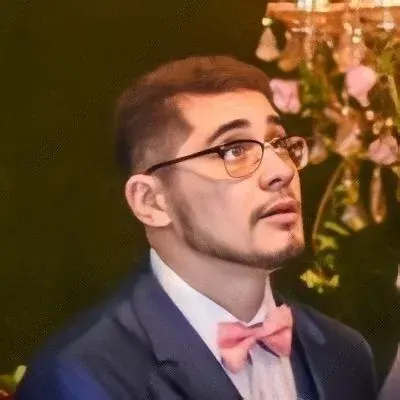
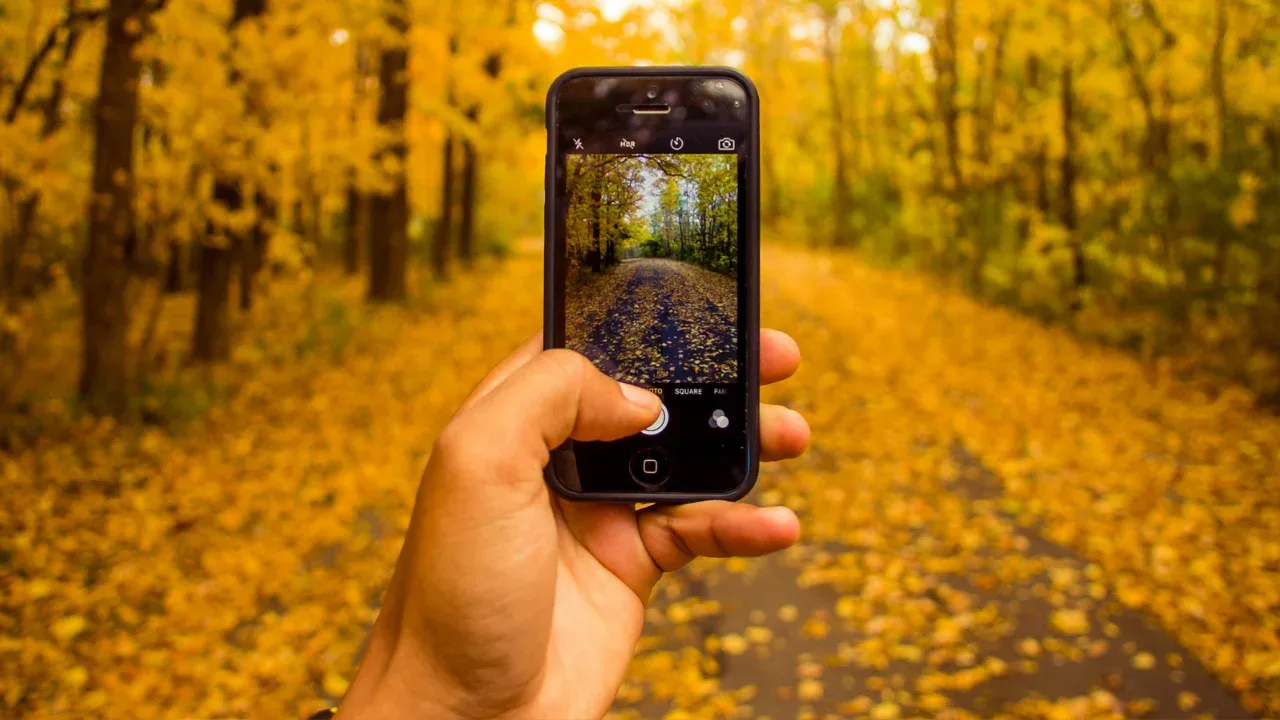
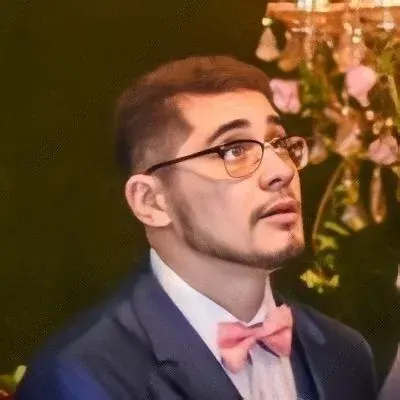
🌟 Rails Model Observers: Handling attribute changes in the after_save callback 🌟
So, you want to send a notification in your Rails application only when a specific attribute, let's say published
, is changed from false
to true
using an after_save
callback. You've come to the right place! 🎉
The Challenge: Determining the attribute change after save
In Rails, the after_save
callback is triggered after the model has been saved to the database. However, it can be a bit tricky to determine which attributes were changed in this callback, especially when you want to filter changes based on a specific condition.
The code snippet you provided attempts to save the original value of the published
attribute before the save occurs. Then, in the after_save
callback, it compares the original value with the current value to determine if the change meets your criteria for sending a notification.
def before_save(blog)
@og_published = blog.published?
end
def after_save(blog)
if @og_published == false and blog.published? == true
Notification.send(...)
end
end
However, this approach might not work as expected due to the order of execution and the potential for race conditions. But fear not, there's a better way to handle this scenario using ActiveRecord's built-in methods. Let's dive in! 💪
The Solution: Utilizing ActiveRecord's dirty tracking
Rails provides a convenient feature called dirty tracking to track changes made to attributes of a model. We can leverage this feature to determine whether the published
attribute has changed from false
to true
. Here's how you can do it:
class BlogObserver < ActiveRecord::Observer
def after_save(blog)
if blog.saved_change_to_published?(from: false, to: true)
Notification.send(...)
end
end
end
In the solution above, we define an observer called BlogObserver
(let's assume that's the model you're working with). Inside the after_save
callback, we use the saved_change_to_attribute?
method provided by ActiveRecord to check if the published
attribute has changed specifically from false
to true
. If it has, we send the notification. 💌
Going Above and Beyond: Cleaner code with Rails 6
Starting from Rails 6, there's an even more concise way to achieve the same result using the new after_commit
callback. This callback is triggered after the transaction is committed to the database, ensuring consistency and reducing the chance of race conditions.
class BlogObserver < ActiveRecord::Observer
def after_commit(blog)
if blog.saved_change_to_published?(from: false, to: true)
Notification.send(...)
end
end
end
By leveraging this new callback, you can simplify your code and enhance the reliability of your notifications. 🚀
The Call-to-Action: Share your tips and tricks!
Congratulations! You've learned how to determine attribute changes in the after_save
callback using Rails model observers. Now it's your turn to share your thoughts, tips, and any alternative solutions you might have. Let's start a discussion below! 💬
If you found this guide helpful, don't forget to share it with fellow Rails developers who might encounter a similar challenge. Happy coding! 😄