Determine if ActiveRecord Object is New
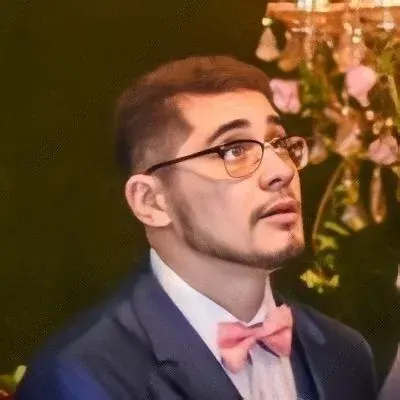
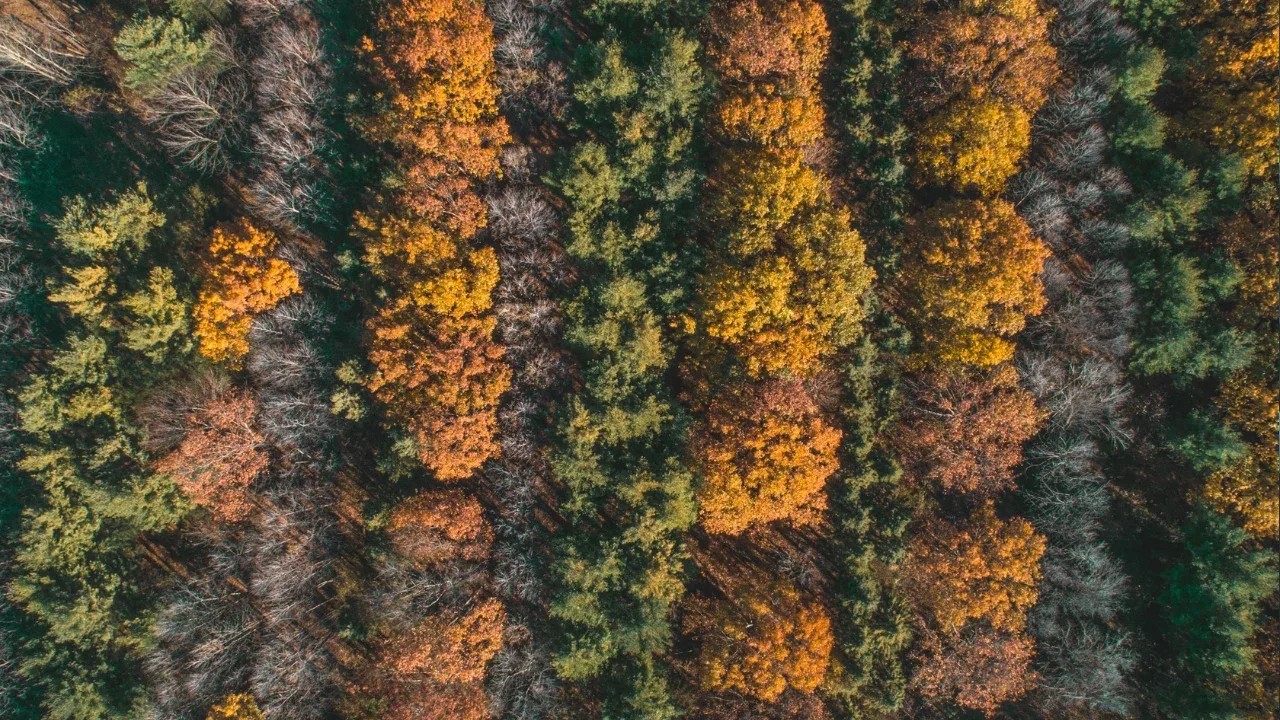
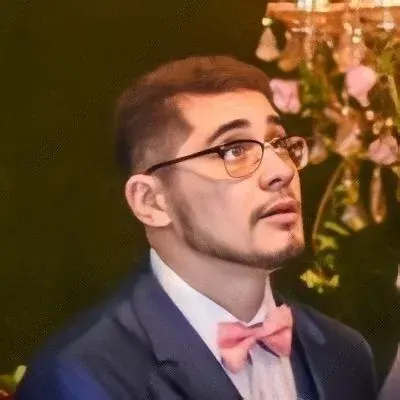
🕵️♂️ Determining if an ActiveRecord Object is New
Do you often find yourself wondering if an ActiveRecord object is new or has already been persisted? 🤔 Fear not, as we've got you covered! In this blog post, we'll tackle this common issue head-on and provide you with some easy solutions. Let's dive right in! 💪
The Challenge
Imagine you have an ActiveRecord object but you're not sure if it has been saved to the database yet. This knowledge can come in handy when you want to handle objects differently based on their persistence status. But how can you determine whether an object is new or already persisted? 🤔
🛠️ Easy Solutions
Thankfully, ActiveRecord provides us with some simple methods to solve this problem effortlessly. Let's explore a few of these methods:
1. new_record?
The new_record?
method is your go-to solution for checking if an object is new. It returns true
if the object has not been saved to the database and false
otherwise.
user = User.new
user.new_record? # returns true
user.save
user.new_record? # returns false
2. persisted?
On the other hand, if you want to determine if an object has already been persisted, you can rely on the persisted?
method. It returns true
if the object has been saved to the database and false
otherwise.
user = User.new
user.persisted? # returns false
user.save
user.persisted? # returns true
3. id.present?
Another approach to check if an ActiveRecord object is new is by using the id.present?
method. It returns true
if the object has been saved to the database and has a valid primary key (id
), and false
otherwise.
user = User.new
user.id.present? # returns false
user.save
user.id.present? # returns true
4. attributes.key?(:id)
In some cases, you may find the attributes
method helpful. You can use it in combination with the key?(:id)
method to determine if an object is new. It returns true
if the object's attributes hash has an id
key, indicating that it has been persisted. Otherwise, it returns false
.
user = User.new
user.attributes.key?(:id) # returns false
user.save
user.attributes.key?(:id) # returns true
🤩 Time to Take Action!
Now that you know some handy methods to determine if an ActiveRecord object is new or persisted, it's time to apply this knowledge to your own projects. Experiment with these methods and find the one that works best for your specific use case.
Remember, understanding an object's persistence status can be crucial for implementing logic that revolves around new and existing records. So why not give it a try today? 😉
Feel free to share your experience with us in the comments below. We'd love to hear your thoughts and any additional tips you might have. Happy coding! 💻🚀