Converting string from snake_case to CamelCase in Ruby
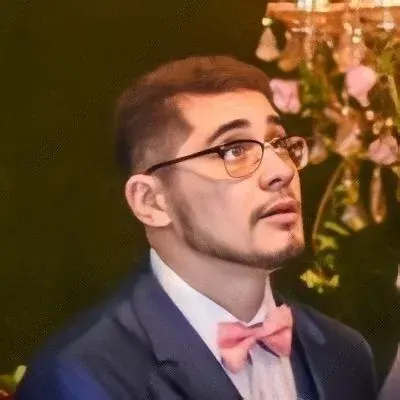
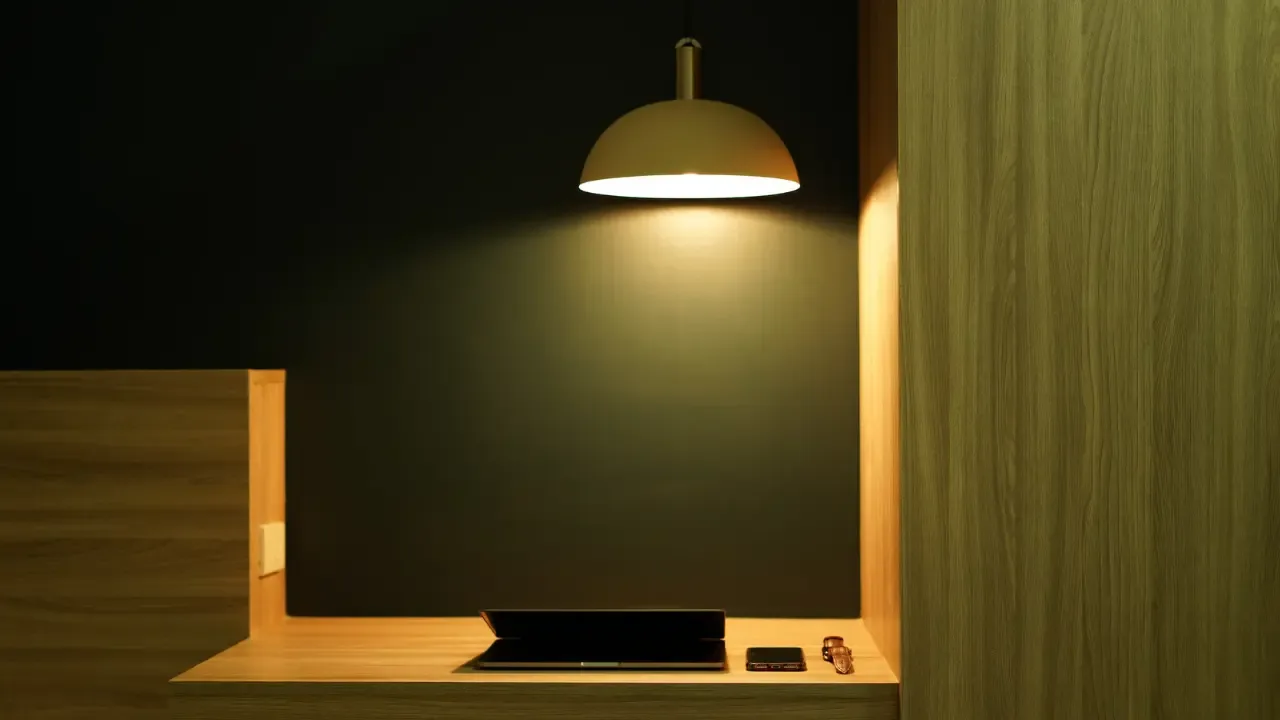
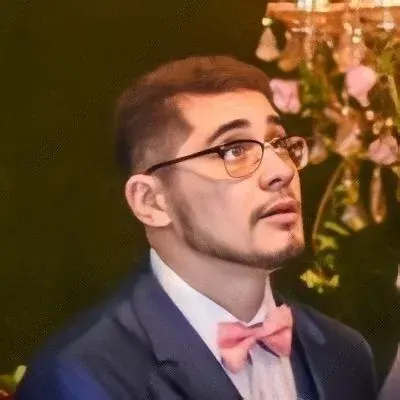
Converting string from snake_case to CamelCase in Ruby: Unleashing the Power of the Ruby Language! πππ₯
Are you tired of manually converting your snake_case strings to CamelCase in Ruby? Don't worry, Ruby has got your back! In this blog post, we'll explore some easy solutions to convert a string from snake_case to CamelCase in Ruby. So, without further ado, let's dive right in! πͺ
The Challenge: Converting "app_user" to "AppUser" πβ‘οΈπ«
Let's say we have a string "app_user" that needs to be converted to "AppUser". The goal is to convert the snake_case string to CamelCase, where each word begins with an uppercase letter except for the first word. This format is commonly used in Ruby for class names and other similar conventions. But how do we achieve this transformation? Let's find out! ππ‘
Solution 1: The Power of String Manipulation π§Άπ§
Ruby provides us with built-in methods to manipulate strings easily. One way to convert snake_case to CamelCase is by splitting the string into words, capitalizing each word's first letter, and then joining them back together. Here's an example code snippet:
def snake_to_camel(snake_case)
snake_case.split('_').map(&:capitalize).join
end
# Usage:
puts snake_to_camel("app_user") # Output: "AppUser"
In this solution, we split the string using the underscore character as the delimiter and then use the map
method with the capitalize
method to capitalize each word's first letter. Finally, we join the words together using the join
method. Voila! We get our desired CamelCase string: "AppUser". π
Solution 2: The Power of Regular Expressions π©βπ¬π
Another efficient way to convert snake_case to CamelCase is by utilizing the power of regular expressions, a powerful tool for pattern matching and manipulation. By capturing each word and applying some transformations, we can easily achieve the desired CamelCase format. Take a look at this code snippet:
def snake_to_camel(snake_case)
snake_case.gsub(/(?:_)([a-z\d]*)/i) { $1.capitalize }
end
# Usage:
puts snake_to_camel("app_user") # Output: "AppUser"
In this solution, we use the gsub
method with a regular expression to find all occurrences of an underscore followed by a word and capture that word. We then apply the capitalize
method to each captured word using a block. The result is the transformed CamelCase string: "AppUser". π
The Power of Ruby: Simplifying Your Development Workflow! πππΌ
With these easy-to-implement solutions, you can effortlessly convert any snake_case string to CamelCase in Ruby. Whether you prefer manipulating strings or harnessing the power of regular expressions, Ruby offers you the flexibility to choose the approach that suits you best. So why waste valuable time and effort on manual conversions when Ruby has your back? Start streamlining your development workflow today with these powerful techniques! β‘οΈπ©
Engage with the Community: Share Your Thoughts! π£οΈπ€π
We hope you found this guide helpful in your quest to convert string from snake_case to CamelCase in Ruby. Now, it's time for you to join the conversation! Share your experiences, other solutions, or any exciting Ruby-related insights in the comments below. Let's learn, share, and grow together as a vibrant tech community! πππ¬
Now go forth and conquer the challenges of string transformations in Ruby! Happy coding! ππ©βπ»π¨βπ»