Check if a table exists in Rails
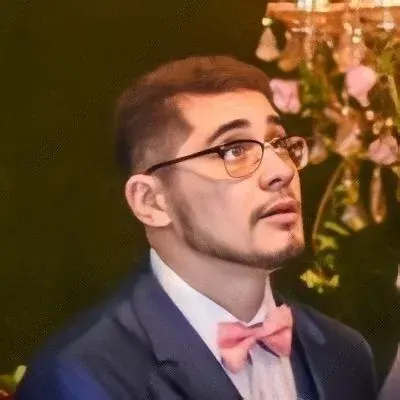
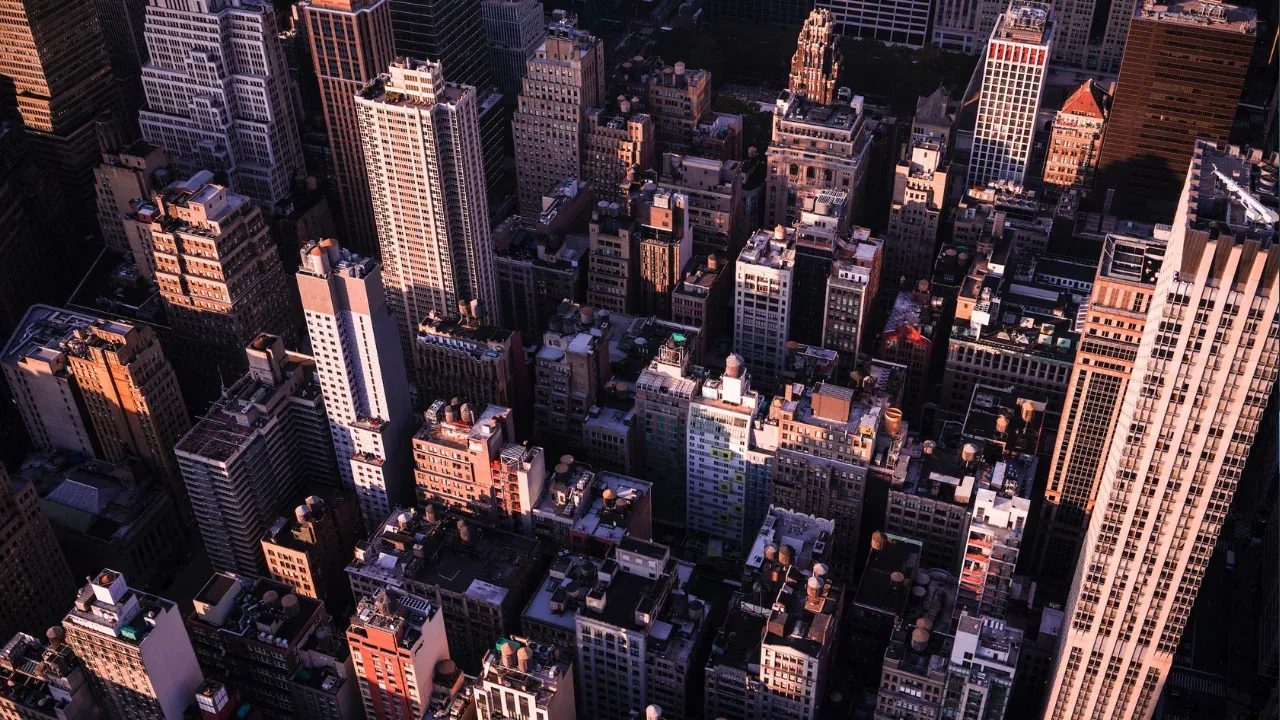
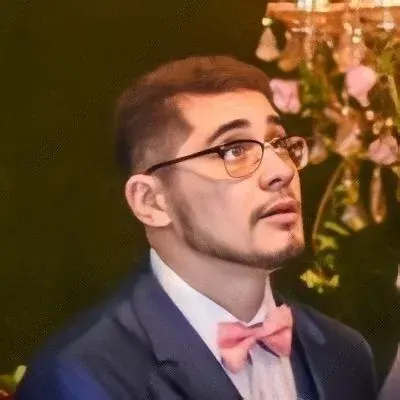
π Title: Checking if a Table Exists in Rails: A Quick and Foolproof Guide! π
Introduction: The Quest for Table Existence π΅οΈββοΈ
Are you a Rails developer working with a team on a website that requires a table to be migrated before certain tasks can be executed? π€ Fret not, my friend! In this blog post, we'll explore common issues, provide easy solutions, and ensure that your team can seamlessly check if a table exists in Rails. Let's dive in! πͺ
The Challenge: Ensuring Successful Table Migration ποΈ
Imagine this scenario: You have a painstakingly crafted rake task that can only function if a specific table exists. With a sizable team of 20 engineers, you want to save everyone's time by ensuring the table has been migrated successfully before they run that rake task. But how do you accomplish this? π€·ββοΈ
Solution 1: ActiveRecord to the Rescue π¦ΈββοΈ
Thankfully, ActiveRecord comes to the rescue! The good news is that Rails provides a method called table_exists?
that you can use to check if a table is already migrated. π‘
Here's how it works:
if ActiveRecord::Base.connection.table_exists?('your_table_name')
puts 'Hooray! The table exists!'
else
puts 'Oops! The table does not exist!'
end
You can replace 'your_table_name'
with the actual name of the table you want to check. Voila! You now have a foolproof way to determine whether the table has been migrated successfully. Simple, isn't it? π
Solution 2: Exception Handling for Extra Confidence π
But wait, there's more! To ensure an even smoother experience, you can leverage exception handling. By rescuing a specific exception, you can gracefully handle any unforeseen errors while attempting to check if the table exists. π
Here's an enhanced version of our previous code snippet:
begin
if ActiveRecord::Base.connection.table_exists?('your_table_name')
puts 'Hooray! The table exists!'
else
puts 'Oops! The table does not exist!'
end
rescue ActiveRecord::NoDatabaseError
puts 'Oops! The database does not exist!'
rescue => e
puts "Oops! An unexpected error occurred: #{e.message}"
end
Now, you not only handle the scenario when the table is missing but also gracefully catch potential database connection errors. This extra layer of care will save you from frustrating moments and empower you to tackle any unforeseen challenges like a true Rails champ! π
Conclusion: Smooth Sailing with Table Existence Checks β΅
Congratulations, fellow Rails developer! You now possess the knowledge to effortlessly check if a table exists in Rails. Whether you choose the straightforward table_exists?
method or embrace the fortification of exception handling, rest assured that your team won't stumble upon unexpected hurdles during the rake tasks. π
So, what are you waiting for? Spice up your codebase, simplify your team's workflow, and save precious time! Go ahead, check if your table exists, and unleash the power of better collaboration and efficiency within your Rails project! You got this! πͺπ»
Have any interesting experiences or additional tips on this subject? Share them in the comments below and let's dive into a lively discussion! π£οΈπ