Can I get the name of the current controller in the view?
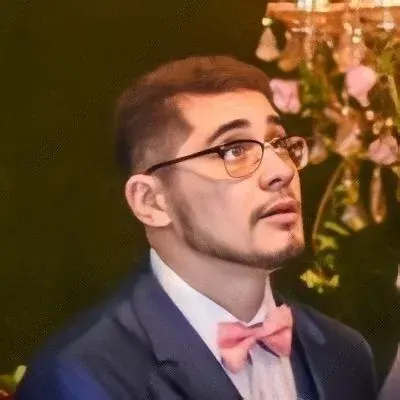
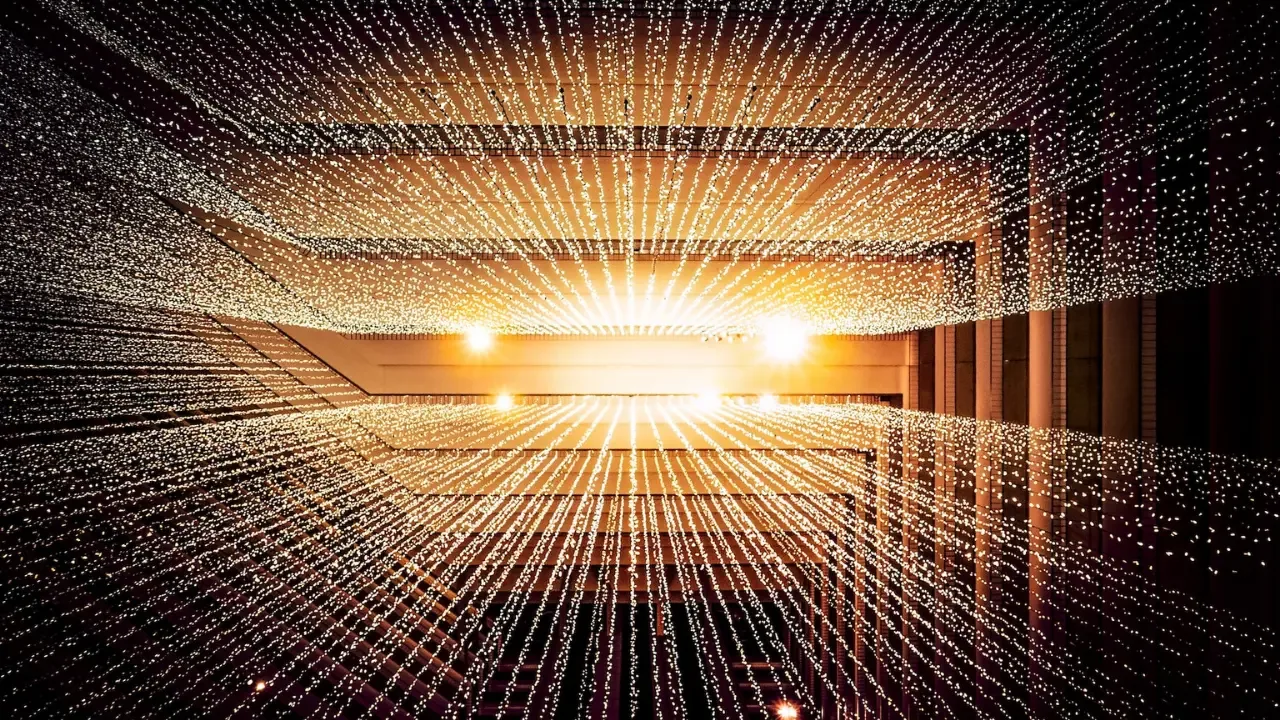
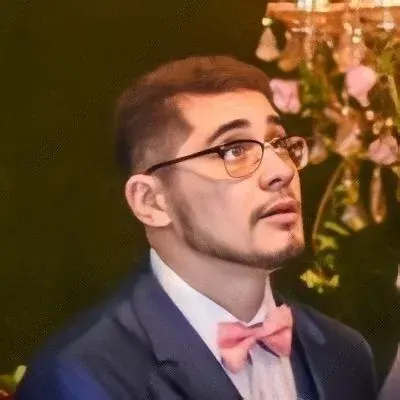
Getting the Name of the Current Controller in Rails Views
š Hey there! Have you ever been in a situation where you wanted to highlight the active menu item in your Rails app based on the current controller? It's a common need when you have multiple controllers sharing the same layout. You might be wondering if there's a built-in way to get the name of the current controller within your view without having to manually specify it in each controller.
š¤ Well, good news! Rails does provide a solution for this problem. In this blog post, we'll explore different approaches to get the name of the current controller in your view and discuss the preferred way to achieve this. Let's dive in!
Approach 1: Using the controller_name
Method
One straightforward way to get the name of the current controller is to use the controller_name
method provided by Rails. This method returns the name of the current controller as a lowercase string.
Here's an example of how you can use it in your view:
<p>Current controller: <%= controller_name.capitalize %></p>
In the above example, we're using the controller_name
method to get the name of the current controller and then capitalizing it to display it in a more friendly format. Feel free to modify this as per your requirements.
Approach 2: Utilizing the params
Hash
Another way to determine the current controller is by using the params
hash. The params
hash contains all the parameters passed to the controller action, including the current controller name.
To access the current controller name from params
, you can simply use params[:controller]
. Here's an example:
<p>Current controller: <%= params[:controller] %></p>
This approach provides you with more flexibility as you can access other parameters as well if needed. However, for just getting the current controller name, Approach 1 is recommended.
Preferred Approach: Using Helper Methods
While both of the above approaches can be used to get the name of the current controller, a more preferred and reusable way is to create a helper method.
In your application_helper.rb
file, you can define a helper method that encapsulates the logic to retrieve the current controller name. For example:
module ApplicationHelper
def current_controller_name
controller_name.capitalize
end
end
Now, you can simply call this helper method in your view:
<p>Current controller: <%= current_controller_name %></p>
By encapsulating the logic in a helper method, you make your code more maintainable and readable. Plus, you can easily reuse this helper method in other views or even in your controllers if needed.
Conclusion and Your Next Step
š Congratulations! You now have different methods to get the name of the current controller in your Rails views. Whether you choose to use the controller_name
method, the params
hash, or create a helper method, it ultimately depends on your specific use case and preferences.
š¬ Now, we'd love to hear from you! Which approach do you find most convenient? Do you have any other approaches to share? Leave a comment below to let us know!
If you found this blog post helpful, consider sharing it with your fellow Rails developers. Happy coding! š