Add timestamps to an existing table
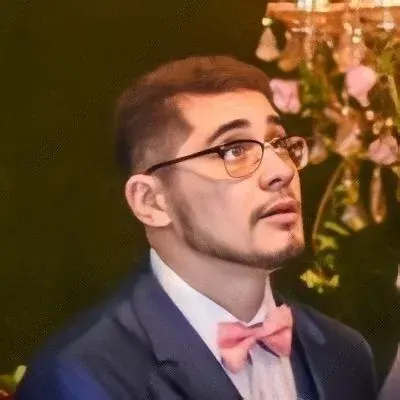
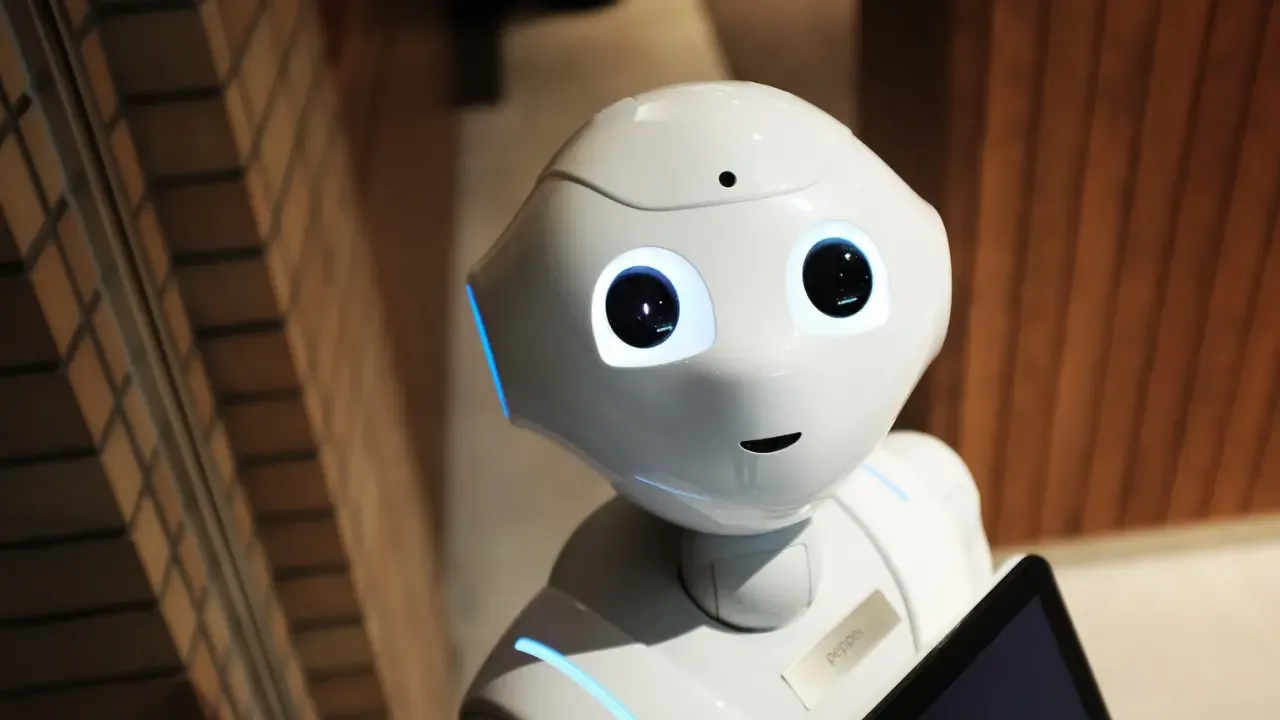
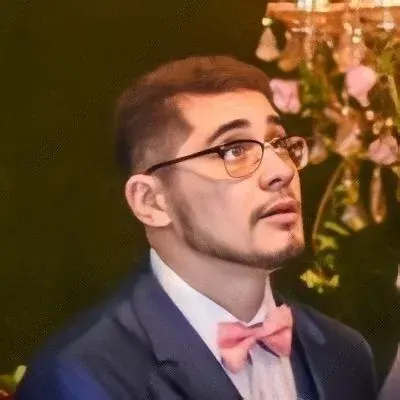
📝 Adding Timestamps to an Existing Table: A Quick Guide
So, you need to add timestamps to an existing table but ran into some issues with the code? Don't worry, we've got you covered! In this blog post, we'll address common problems with adding timestamps and provide you with easy solutions. Let's dive in! 💪
The Code Snippet
Here's the code snippet you tried:
class AddTimestampsToUser < ActiveRecord::Migration
def change_table
add_timestamps(:users)
end
end
The Problem
The issue you faced is that the code didn't work as expected. To understand why, let's take a closer look at the usage of the change_table
method in ActiveRecord migrations.
Understanding change_table
Method
The change_table
method is typically used when making structural changes to a table, like adding or removing columns. However, when it comes to adding timestamps, a different approach is required.
Solution: Using change
Method
To add timestamps to an existing table, we can make use of the change
method instead. Here's an updated version of the code:
class AddTimestampsToUser < ActiveRecord::Migration
def change
add_timestamps(:users)
end
end
By using the change
method, Rails will automatically generate the necessary code to add the created_at
and updated_at
columns as timestamps to the users
table.
Applying the Migration
To apply this migration, you need to run the following command in your terminal:
$ rails db:migrate
This command will execute all pending migrations, including the one we just created to add timestamps to the users
table.
Conclusion
Adding timestamps to an existing table is a common requirement in many applications. By following the correct approach and using the change
method instead of change_table
, you can easily accomplish this task.
We hope this guide helped you resolve the issue you faced and provided you with a better understanding of the underlying concepts. If you have any further questions or need additional assistance, please feel free to leave a comment below. Happy coding! 😄🚀
[Code snippet and context provided by the user]