How to write a switch statement in Ruby
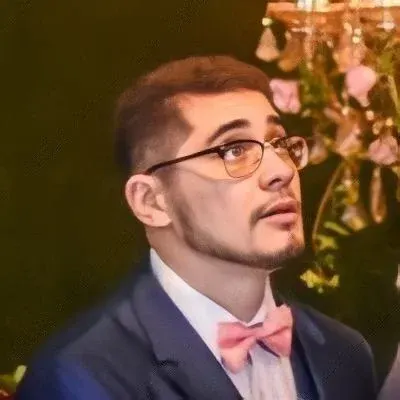
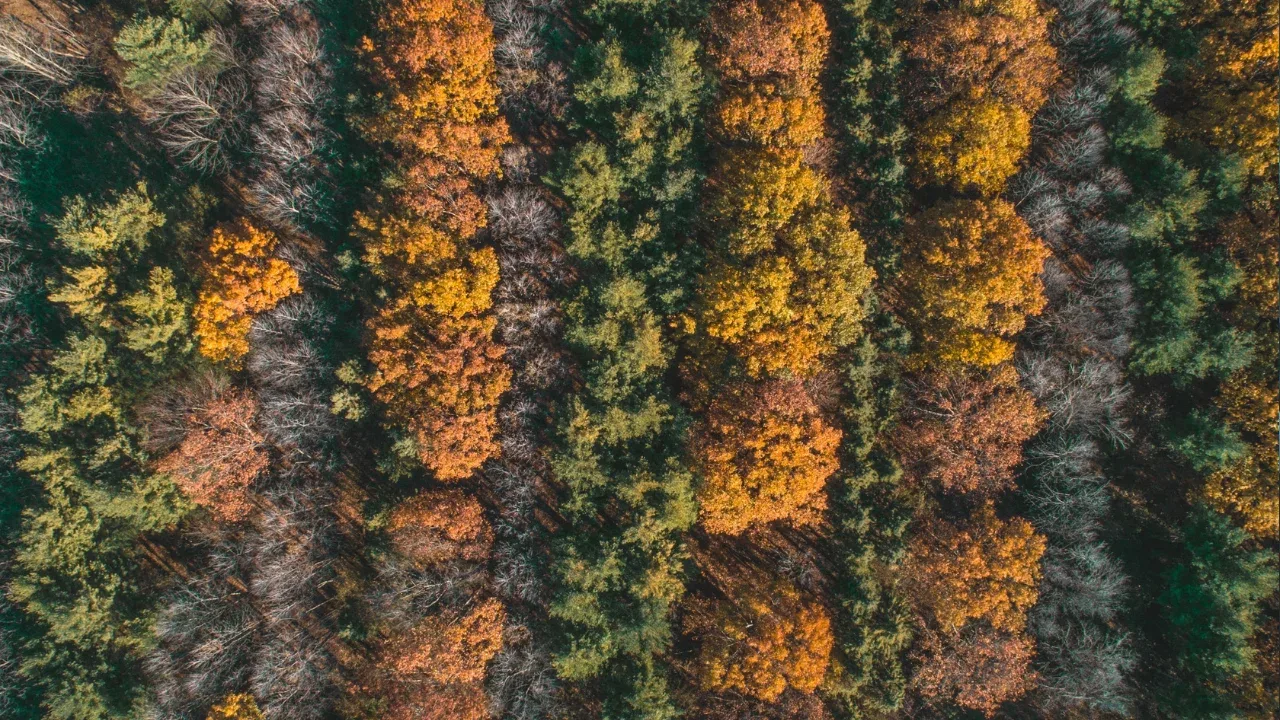
How to Write a Switch Statement in Ruby: Simplified Guide 🚀
Introduction
Ruby, an elegant and dynamic programming language, offers a variety of control structures to handle different scenarios. However, Ruby does not have a built-in switch
statement like some other languages. But worry not! In this guide, we'll explore alternative techniques to achieve similar functionality using Ruby.
The Problem
Ruby does not have a switch
statement like languages such as Java or C++. This often confuses developers who are familiar with these languages, leading to code that is less expressive and harder to maintain.
The Solution: Case Statements
In Ruby, we can leverage the power of the case
statement to achieve the same functionality as a switch
statement. The case
statement enables us to evaluate one or more expressions and execute a block of code based on the evaluation result.
Here's the basic syntax of a case
statement:
case expression
when value1
# code to execute if expression matches value1
when value2
# code to execute if expression matches value2
else
# code to execute if expression matches none of the values
end
The
expression
represents the value you want to compare.The
when
keyword specifies the value to match against.The
else
block is optional and executes when none of the values match.
Here's an example to illustrate the usage of a case
statement in Ruby:
def convert_grade(grade)
case grade
when 90..100
'A'
when 80..89
'B'
when 70..79
'C'
else
'F'
end
end
puts convert_grade(85)
# Output: B
In the example above, we define a method called convert_grade
that takes a grade
argument. The case
statement checks the value of the grade
and returns the corresponding letter grade.
Advanced Techniques
The case
statement in Ruby can handle various types of expressions, not just simple values. Here are a few advanced techniques to enhance your code:
Pattern Matching with Regular Expressions
Instead of using plain values, you can utilize regular expressions to match more complex patterns. This is useful when dealing with string comparisons.
case email
when /[A-Z0-9._%+-]+@[A-Z0-9.-]+\.[A-Z]{2,}/i
puts 'Valid email address'
else
puts 'Invalid email address'
end
Method Calls
You can even call a method directly inside a case
statement to determine which code block to execute.
case get_user_role(user)
when 'admin'
puts 'Access granted!'
when 'user'
puts 'Access granted with limitations.'
else
puts 'Access denied!'
end
Your Turn
Now that you understand how to write a switch
statement in Ruby using case
, it's time to put your knowledge into practice. Try refactoring existing code or create new projects while utilizing this powerful control structure. Share your experiences and code in the comments below!
Conclusion
Although Ruby doesn't have a switch
statement like other languages, the case
statement provides a flexible alternative. By leveraging the case
statement, we can write expressive and concise code, solving complex problems with ease. Remember to make the most out of advanced techniques like regular expressions and method calls within your case
statements.
So, go ahead and level up your Ruby skills by embracing the versatility of the case
statement. Happy coding! 💻✨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
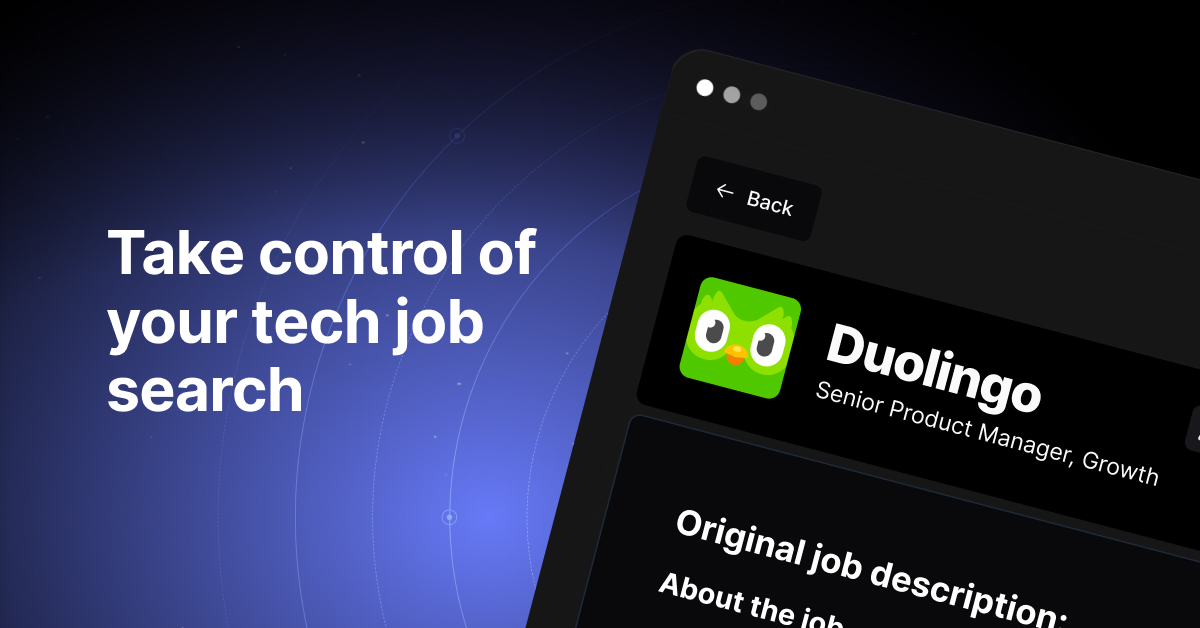