How to sum array of numbers in Ruby?
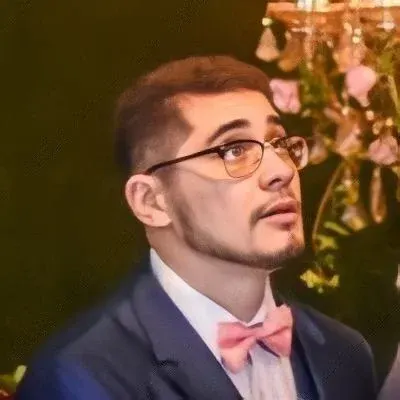
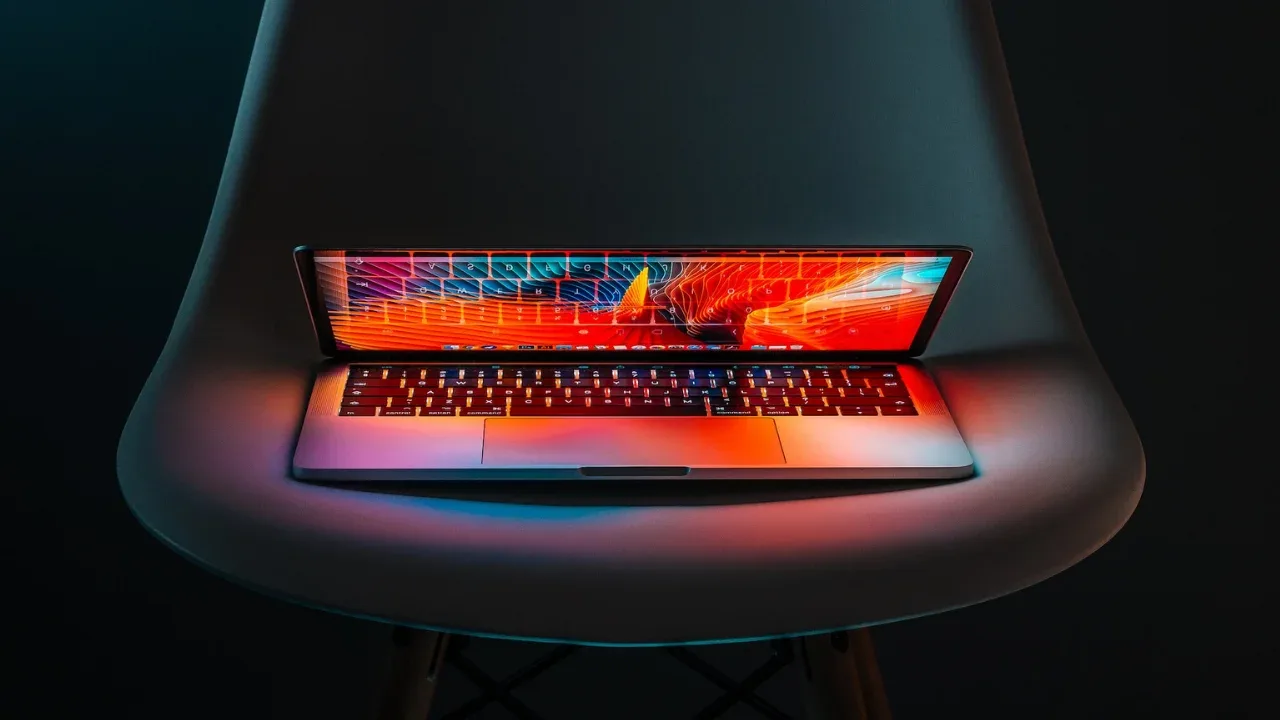
How to Sum Array of Numbers in Ruby? 💎
Are you struggling to find an easy way to sum an array of numbers in Ruby? Look no further! In this guide, we'll explore a simple and efficient solution to tackle this common problem. Let's dive right in! 💪
The Problem 🤔
So, you have an array of integers, and you want to calculate their sum. For instance, consider the following array:
array = [123, 321, 12389]
How can we get the sum of all the numbers in this array? 💡
The Solution 🌟
The good news is, Ruby provides multiple ways to sum an array of numbers. Let's explore two of the most popular and convenient methods.
Method 1: Using the inject
Method 🚀
Ruby's inject
method is an excellent option to calculate the sum in a concise and elegant way. Here's how you can use it:
array = [123, 321, 12389]
sum = array.inject(0) { |result, element| result + element }
In this example, we start with an initial value of 0
(specified as the argument to inject
). Then, for every element in the array, we add it to the result. The final result is stored in the sum
variable.
Method 2: Using the sum
Method 🎯
Ruby 2.4 introduced the sum
method as a convenient way to calculate the sum of an array of numbers. Here's how you can use it:
array = [123, 321, 12389]
sum = array.sum
Yes, it's as simple as that! Just call the sum
method on your array, and it will return the sum of all the numbers.
Choosing the Right Method 😎
Both methods are valid, but which one should you choose? It depends on your specific needs and the version of Ruby you are using.
If you're running Ruby 2.4 or newer, the
sum
method provides a clean and straightforward solution. It's easy to read and requires less code.If you're using an older version of Ruby or want more control over the summing process, the
inject
method gives you the flexibility to customize the logic.
Don't worry; both methods are efficient and will get the job done! 👍
Time to Sum it Up! ✨
Now, armed with the knowledge of these two powerful methods, you can easily sum an array of numbers in Ruby. Whether you prefer the elegance of the inject
method or the simplicity of the sum
method, you have the tools to conquer this problem.
So go ahead, give these methods a try, and start summing those arrays like a Ruby pro! Happy coding! 😄
If you have any questions or any other cool ways to sum arrays in Ruby, let me know in the comments below. Let's learn from each other! 👇
Remember, great things happen when you sum it up in Ruby! 💎
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
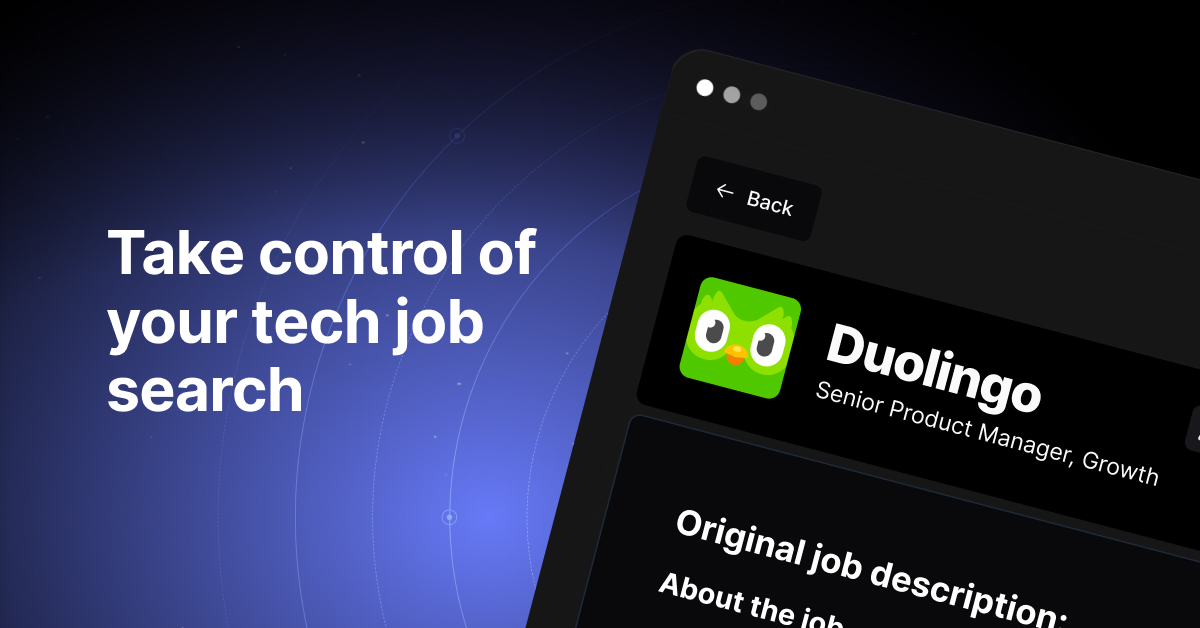