How to map/collect with index in Ruby?
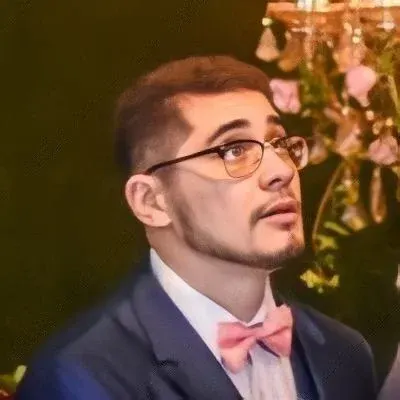
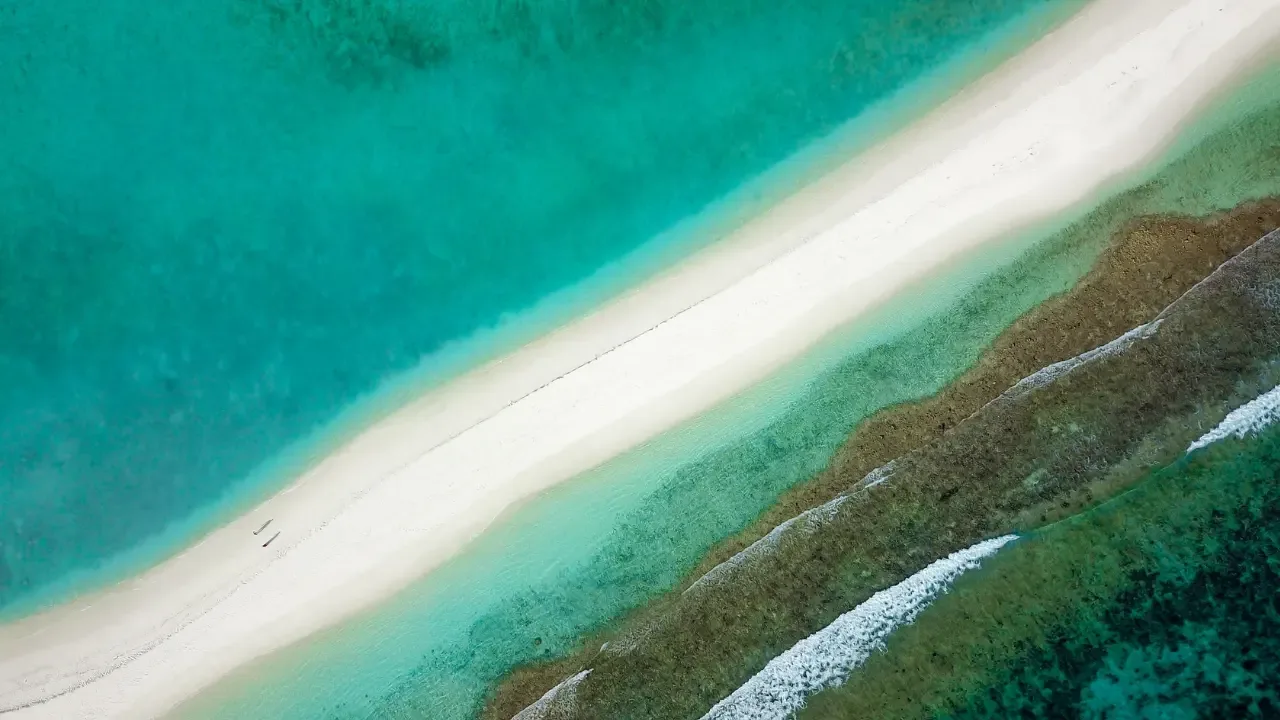
ποΈ Easy Mapping and Collecting with Index in Ruby π
Are you tired of struggling with converting arrays in Ruby? Do you find it challenging to map and collect with indexes efficiently? Fear not! In this blog post, we are going to address a common issue and provide you with easy solutions. Get ready to level up your Ruby skills! π
The Challenge π€
Let's dive into the problem you're facing. You have an array, like this:
[x1, x2, x3, ..., xN]
And you want to convert it into a new array that contains sub-arrays where each element is paired with its respective index plus one, like this:
[[x1, 2], [x2, 3], [x3, 4], ..., [xN, N+1]]
How can we accomplish this task easily and efficiently? Let's explore some solutions together! π‘
Solution 1: Using .each_with_index
π
One possible solution is to use the .each_with_index
method in Ruby. This method allows us to iterate over each element of the array while also providing its index. Here's an example of how this solution would look:
result = []
array.each_with_index do |element, index|
result << [element, index + 2]
end
In this code snippet, we create an empty array called result
to store our transformed data. Then, for each element in the original array, we build a sub-array that contains the element itself and its respective index plus one. Finally, we append this sub-array to the result
array. Easy-peasy! π
Solution 2: Using .map.with_index
πΊοΈ
Another elegant solution involves using the .map.with_index
method in Ruby. This combination allows us to achieve the desired transformation in a single line of code, making it both concise and efficient. Take a look at this example:
result = array.map.with_index { |element, index| [element, index + 2] }
In this snippet, we call .map.with_index
on the original array and pass a block that will be executed for each element. This block builds the sub-array and automatically returns it, resulting in the transformed array we desire. Isn't that amazing? π
Solution 3: Using each_with_object
π
If you prefer a different approach, we can also achieve the desired result using the each_with_object
method in Ruby. This method allows us to accumulate elements into a new object, which is perfect for our case. Check out this example to see how it works:
result = array.each_with_object([]).with_index { |(element, acc), index| acc << [element, index + 2] }
In this code snippet, we call .each_with_object([])
on the original array to specify the object we want to accumulate our transformed data into. Then, we chain .with_index
to access both the element and the object during the iteration. Finally, we append the sub-array to the object using the accumulator (acc
). Fantastic! π
Wrap Up and Get Mapping! π
We've explored three easy and efficient solutions to convert an array into a transformed array in Ruby. From using .each_with_index
to .map.with_index
and each_with_object
, you now have multiple options to choose from. Use the solution that resonates with you the most and achieve your mapping goals effortlessly. πͺ
So what are you waiting for? Apply these techniques to your code and say goodbye to array mapping headaches! Let us know in the comments which solution you prefer or if you have any additional tips to share. Happy coding! ππ₯
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
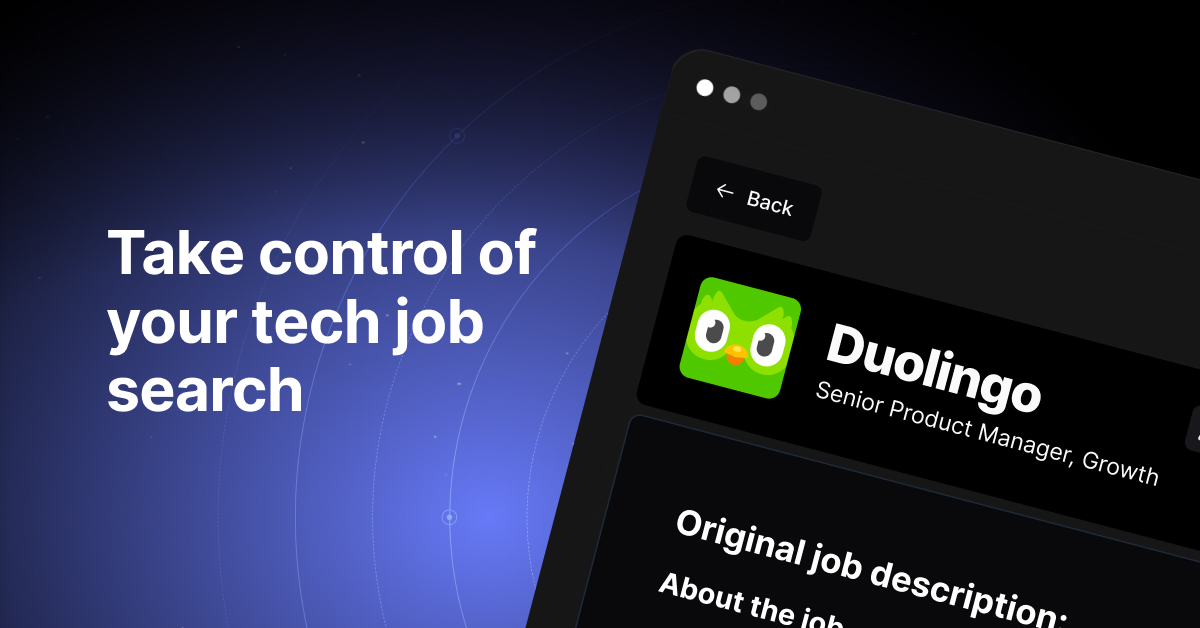