How to generate a random string in Ruby
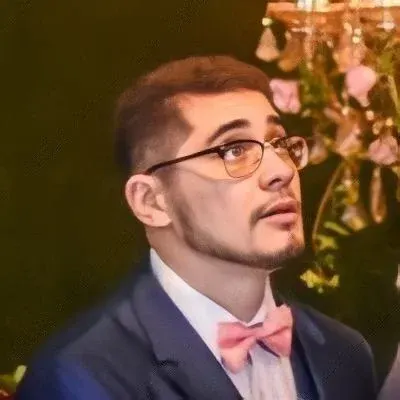
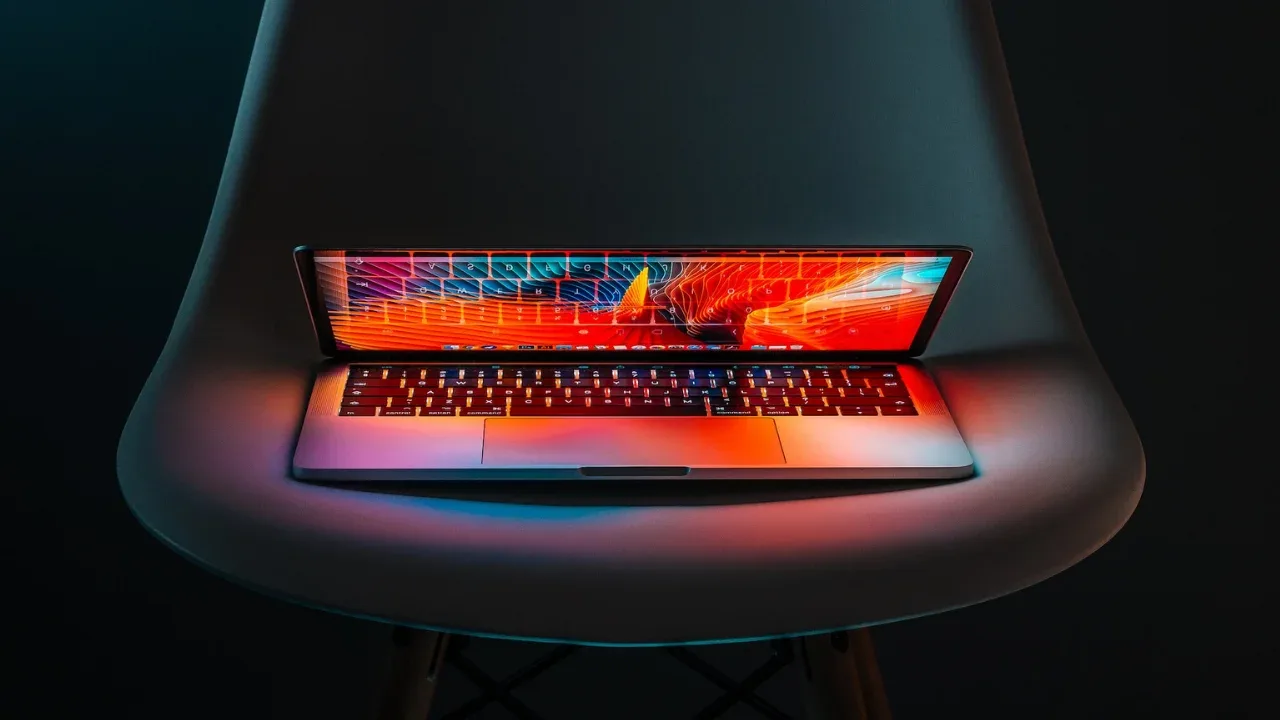
How to Generate a Random String in Ruby: Easy Solutions for a Common Issue
Are you tired of using messy and inefficient code to generate a random string in Ruby? Look no further! In this blog post, we'll explore the common issues faced when trying to generate a random string and provide you with some easy solutions that will make your code look cleaner and more efficient. ๐
The Initial Problem
Let's start by examining the problem at hand. The initial code provided generates an 8-character pseudo-random uppercase string from "A" to "Z". Here's the code snippet:
value = ""; 8.times{value << (65 + rand(25)).chr}
While it may work, there are a couple of issues with this code:
Messy and unreadable: The code isn't concise and can be hard to read due to the embedded logic.
Not easily adaptable: Since it's not a single statement, it can't be easily passed as an argument to other functions or methods.
But fear not! We have some easy and elegant solutions coming right up. ๐
Solution 1: Generating a Uppercase and Lowercase Random String
If you're looking to generate a mixed-case string ranging from "a" to "z" and "A" to "Z", we've got a simple solution for you. Check out the code snippet below:
value = ""; 8.times{value << ((rand(2)==1 ? 65 : 97) + rand(26)).chr}
This solution utilizes a ternary operator to determine whether to add 65 or 97 to the randomly generated number. This way, you'll get a uniform mix of uppercase and lowercase letters.
However, we understand that this code may not look as clean and elegant as you'd like. So, let's move on to an even better solution. ๐
Solution 2: Utilizing Ruby's SecureRandom
Library
Ruby provides a powerful library called SecureRandom
, which is perfect for generating random strings. This library offers a wide range of options, including generating random numbers, UUIDs, and random bytes. In this case, we'll focus on generating random strings.
Here's how you can utilize SecureRandom
to generate a random string:
require 'securerandom'
value = SecureRandom.alphanumeric(8).upcase
With just a single line of code, you can generate an 8-character alphanumeric string in uppercase. The alphanumeric
method ensures that the generated string contains both letters and numbers (a-z, A-Z, and 0-9).
Isn't that much cleaner and easier to read? Plus, using SecureRandom
provides added security and randomness to your generated strings.
Your Turn to Experiment!
Now that you have some easy solutions to generate random strings in Ruby, it's time for you to get creative and try them out in your own code. ๐งช
Experiment with different lengths, variations, and use cases. Whether you need a random password generator, unique identifiers, or any other random string, these solutions should have you covered.
We'd love to hear about your experiences and any improvements you make. Share your thoughts, sample code, or questions in the comments section below. Let's create some truly random and awesome strings together! ๐
Happy coding! ๐ป
Note: It's important to consider your specific requirements and security needs when generating random strings. Make sure to research and understand the implications before implementing any solution.
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
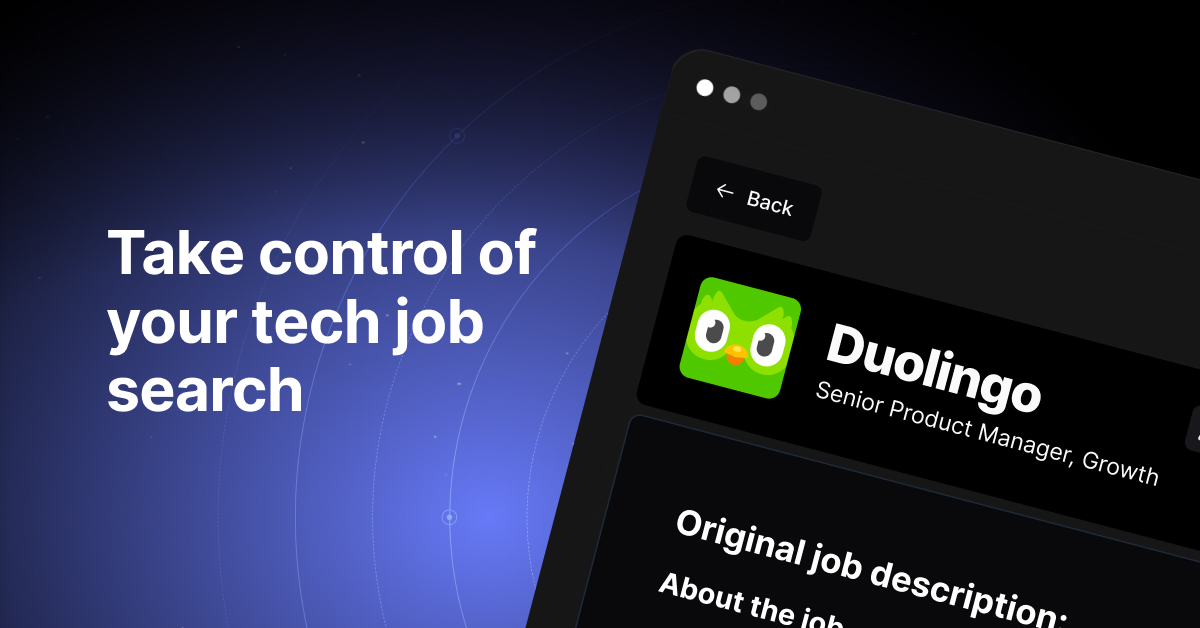